· Charlotte Will · 6 min read
The Ultimate Guide to Making API Requests in Python for Web Scraping
Master making API requests in Python for efficient web scraping. Learn how to set up your environment, make GET & POST requests, handle authentication, parse JSON responses, and follow best practices. Enhance your data extraction skills today!
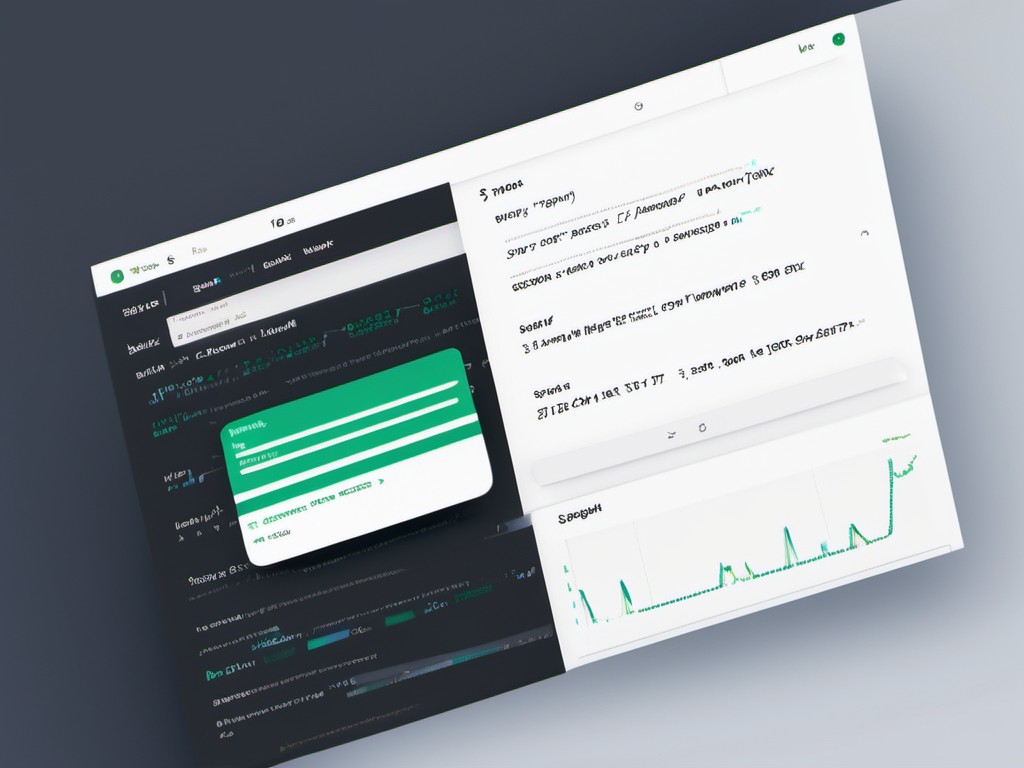
Welcome to the ultimate guide on making API requests in Python for web scraping! In this comprehensive article, we’ll delve deep into understanding APIs, setting up your environment, making various types of API requests, handling authentication, parsing responses, and best practices to ensure you can effectively extract data from the web.
Introduction to APIs and Web Scraping
What is an API?
An Application Programming Interface (API) is a set of rules and protocols that allows different software applications to communicate with each other. In simpler terms, APIs enable developers to access data or functionality provided by another service without knowing the underlying implementation details.
Why Use APIs for Web Scraping?
While traditional web scraping involves parsing HTML directly from a website, using APIs provides several advantages:
- Structured Data: APIs return data in a structured format like JSON or XML, making it easier to parse and use.
- Legal Compliance: Many websites offer public APIs that allow you to access their data legally.
- Rate Limits: APIs often come with rate limits, which help manage the load on the server and prevent your requests from being blocked.
Setting Up Your Environment
Before diving into making API requests, let’s set up our Python environment.
Installing Necessary Libraries
To make API requests in Python, you’ll primarily need the requests
library. You can install it using pip:
pip install requests
The requests
library is a simple and elegant HTTP library for Python that makes sending HTTP requests straightforward.
Making Basic API Requests
Now that we have our environment set up, let’s start making some basic API requests.
GET Requests
A GET request is used to retrieve data from the server. Here’s a simple example:
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
print(response.json())
else:
print('Failed to retrieve data')
In this example, we send a GET request to the specified URL and check if the response status code is 200 (indicating success). If successful, we print the JSON data returned by the API.
POST Requests
A POST request is used to send data to the server for processing. Here’s how you can make a POST request:
import requests
data = {
'key1': 'value1',
'key2': 'value2'
}
response = requests.post('https://api.example.com/submit', json=data)
if response.status_code == 201:
print(response.json())
else:
print('Failed to submit data')
In this example, we send a POST request with some JSON data and handle the response similarly to the GET request.
Handling Authentication and Headers
Many APIs require authentication or custom headers to access their data. Let’s see how you can handle these aspects.
API Keys and Tokens
Some APIs use API keys or tokens for authentication. You typically include them in the request headers:
import requests
headers = {
'Authorization': 'Bearer YOUR_API_KEY'
}
response = requests.get('https://api.example.com/data', headers=headers)
if response.status_code == 200:
print(response.json())
else:
print('Failed to retrieve data')
Replace YOUR_API_KEY
with your actual API key.
Custom Headers
Sometimes, APIs require additional headers for proper functioning:
import requests
headers = {
'User-Agent': 'your-user-agent',
'Accept': 'application/json'
}
response = requests.get('https://api.example.com/data', headers=headers)
if response.status_code == 200:
print(response.json())
else:
print('Failed to retrieve data')
Including custom headers can help you avoid common issues like being blocked by the server for not providing a user-agent string.
Parsing JSON Responses
Most APIs return data in JSON format. Let’s see how we can parse and use this data in Python:
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json()
print(data['key']) # Accessing a specific key in the JSON data
else:
print('Failed to retrieve data')
The response.json()
method converts the JSON response into a Python dictionary, making it easy to access and manipulate the data.
Best Practices for Web Scraping with APIs
Error Handling
Always include error handling in your code to manage unexpected responses:
import requests
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status() # Raises an HTTPError for bad status codes
data = response.json()
except requests.exceptions.HTTPError as http_err:
print(f'HTTP error occurred: {http_err}')
except Exception as err:
print(f'Other error occurred: {err}')
else:
print(data)
Using response.raise_for_status()
helps catch HTTP errors early, making your code more robust.
Rate Limiting
Respect the API’s rate limits to avoid getting banned:
import time
import requests
url = 'https://api.example.com/data'
max_retries = 3
retry_delay = 2 # seconds
for attempt in range(max_retries):
response = requests.get(url)
if response.status_code == 429: # Too Many Requests
print('Rate limit exceeded, retrying...')
time.sleep(retry_delay)
else:
break
if response.status_code == 200:
print(response.json())
else:
print('Failed to retrieve data')
By implementing a simple retry mechanism with a delay, you can handle rate limits gracefully.
Common Pitfalls and How to Avoid Them
Ignoring API Documentation
Always read the API documentation thoroughly to understand the endpoints, parameters, authentication methods, and rate limits.
Not Handling Exceptions Properly
Failing to handle exceptions can lead to your script crashing unexpectedly. Always include error handling in your code.
Overlooking Rate Limits
Respecting rate limits is crucial for maintaining access to the API. Implement rate-limiting strategies to avoid getting banned.
Conclusion
Making API requests in Python for web scraping can be a powerful and efficient way to extract data from the web. By understanding APIs, setting up your environment properly, making various types of requests, handling authentication, parsing responses, and following best practices, you can become proficient in web scraping with APIs.
FAQs
What are the best libraries for making API requests in Python?
The requests
library is widely regarded as the best for making API requests due to its simplicity and powerful features. Other notable mentions include httpx
and aiohttp
, which support async I/O for handling multiple requests concurrently.
How do I handle rate limits when scraping APIs?
To handle rate limits, you can implement a retry mechanism with delays between requests. Additionally, some APIs provide headers like Retry-After
that specify the delay before you can make another request. Always respect these limits to avoid getting banned.
What is the difference between GET and POST requests?
A GET request is used to retrieve data from a server, while a POST request is used to send data to the server for processing. GET requests are typically used for reading data, whereas POST requests are often used for submitting forms or creating new resources.
How can I parse JSON responses in Python?
You can use the json()
method provided by the requests
library to convert the JSON response into a Python dictionary. This makes it easy to access and manipulate the data as needed.
What should I do if an API returns a 403 Forbidden error?
A 403 Forbidden error typically indicates that you don’t have the necessary permissions to access the resource. To resolve this issue, check your API key or token, ensure that it is included in the request headers correctly, and verify that you have the required access rights for the specific endpoint.