· Charlotte Will · 4 min read
The Importance of Python Webscraping in Data Analysis
Discover how Python web scraping can revolutionize your data analysis projects. Learn practical techniques, benefits, and best practices for efficient and ethical web scraping with Python.
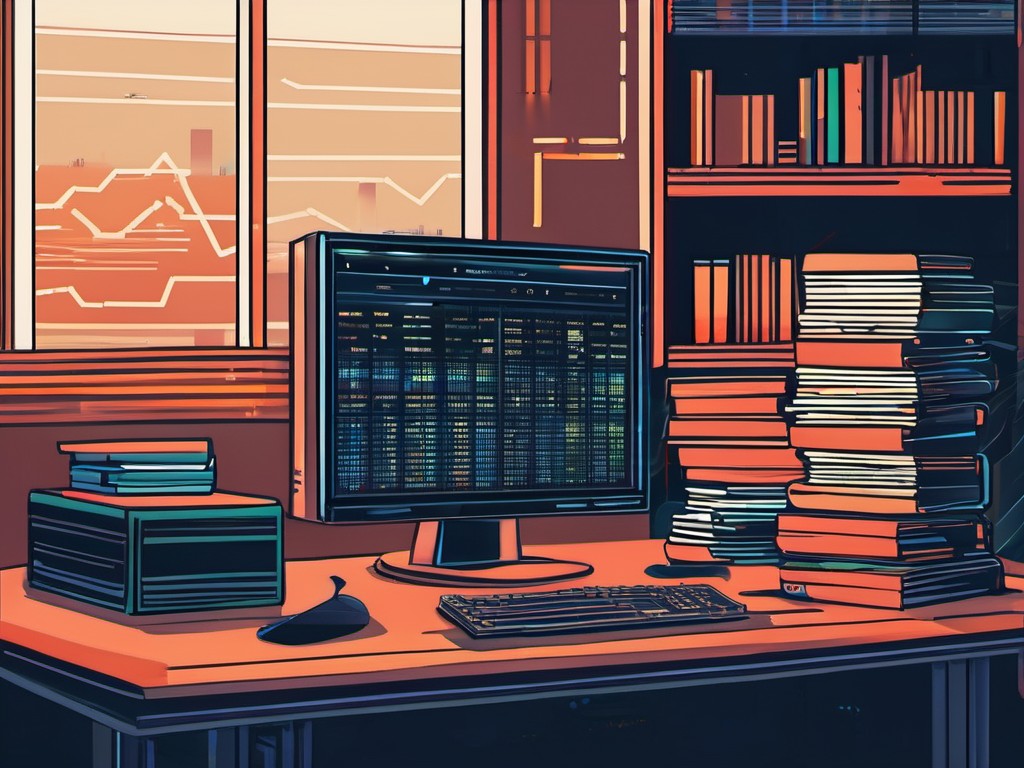
Introduction to Python Web Scraping
What is Web Scraping?
Web scraping, also known as web data extraction, is the process of extracting information from websites. This data can be used for various purposes, such as market research, price monitoring, or content aggregation. With Python, web scraping becomes more efficient and powerful due to its extensive libraries and ease of use.
Why Choose Python for Web Scraping?
Python is an excellent choice for web scraping due to several reasons:
- Ease of Use: Python’s syntax is straightforward, making it accessible even for beginners.
- Libraries: Libraries like BeautifulSoup, Scrapy, and Selenium simplify the scraping process.
- Community Support: A large community means plenty of resources, tutorials, and forums to help you out.
Benefits of Python Web Scraping in Data Analysis
Efficient Data Collection
Web scraping allows you to collect data from multiple sources quickly and efficiently. This data can be used to identify trends, perform comparative analysis, or even train machine learning models.
Automation and Scalability
Python scripts can automate the entire web scraping process, allowing for scalable data collection. This is particularly useful for monitoring large datasets over time or gathering data from numerous websites simultaneously.
Practical Guide to Python Web Scraping
Setting Up Your Environment
To start with web scraping, you’ll need a few essential tools:
- Python: Ensure you have the latest version of Python installed.
- Libraries: Install libraries like BeautifulSoup, Scrapy, and Selenium using pip.
- Editor/IDE: Choose an editor or IDE that suits your workflow (e.g., VS Code, PyCharm).
Writing Your First Web Scraper
Here’s a simple example using BeautifulSoup to scrape data from a website:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.find_all('div', class_='example-class')
for item in data:
print(item.text)
Handling Dynamic Content and APIs
For websites with dynamic content, you might need to use Selenium, which can render JavaScript. Additionally, some sites offer APIs that provide structured data, making scraping easier and more ethical.
Best Practices for Effective Data Analysis with Web Scraping
Ethical Considerations
When web scraping, always consider the legal and ethical implications:
- Respect Robots.txt: This file indicates which parts of a website can be scraped.
- Avoid Overloading Servers: Implement delays between requests to avoid overwhelming servers.
- Comply with Terms of Service: Ensure you are not violating the site’s terms of service.
Optimizing Performance
To optimize performance:
- Use Headless Browsers: Tools like Selenium support headless browsing, which is faster and less resource-intensive.
- Parallelize Requests: Libraries like Scrapy can handle multiple requests simultaneously, speeding up the process.
Tools and Libraries for Python Web Scraping
BeautifulSoup
BeautifulSoup is a powerful library for parsing HTML and XML documents. It creates a parse tree from page source code that can be used to extract data in a hierarchical, readable manner.
Scrapy
Scrapy is an open-source web crawling framework for Python. It’s particularly useful for large-scale scraping projects due to its built-in support for handling concurrent requests and scheduling.
Selenium
Selenium is a suite of tools used for automating web browsers. It’s ideal for scraping dynamic content that relies on JavaScript, as it can render the full page before extracting data.
FAQ Section
Q: What legal considerations should I keep in mind when web scraping? A: Always respect the website’s robots.txt
file and terms of service. Avoid scraping sensitive or personal information without explicit permission.
Q: How can I handle CAPTCHAs while web scraping? A: Handling CAPTCHAs can be challenging but using services like 2Captcha or Anti-Capture might help automate the process. Alternatively, consider switching to a more scrape-friendly site.
Q: What are some advanced techniques for web scraping with Python? A: Advanced techniques include handling rate limits, rotating proxies, and using machine learning to extract data from complex structures.
Q: Can I scrape data in real-time? A: Yes, you can set up scripts to scrape data at regular intervals or use tools that support real-time scraping through APIs.
Q: How do I store and analyze the scraped data? A: Store the data in databases like SQLite, MySQL, or MongoDB. Use libraries such as Pandas for data analysis and visualization tools like Matplotlib or Seaborn to gain insights from your data.