· Charlotte Will · Amazon API · 5 min read
Scraping Single Page Applications (SPA) with Headless Browsers: A Comprehensive Guide
Learn how to use headless browsers like Puppeteer to scrape Single Page Applications (SPA) effectively. This comprehensive guide covers practical techniques, error handling, and optimization strategies for reliable SPA scraping.
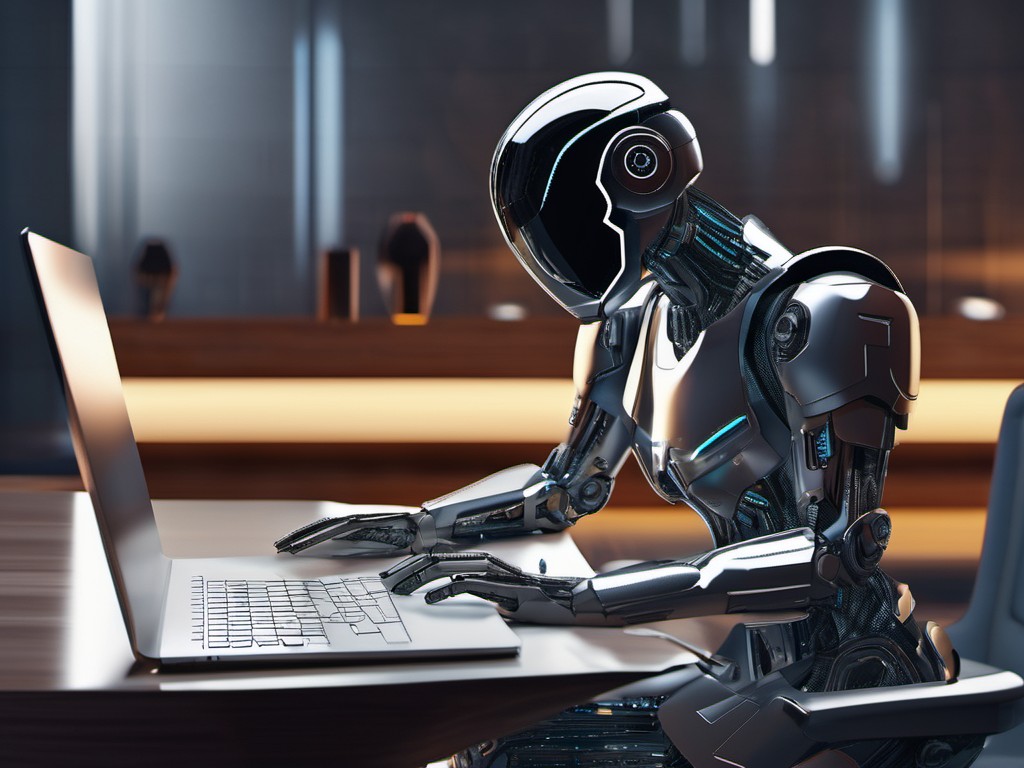
In today’s web development landscape, Single Page Applications (SPAs) have become increasingly popular due to their dynamic and responsive nature. However, this popularity has also introduced a significant challenge for web scraping tasks, as traditional scraping methods often fall short when dealing with SPAs. Enter headless browsers: powerful tools designed to automate browser interactions in the same way humans do, making them an essential asset for SPA scraping. This comprehensive guide will walk you through the process of using headless browsers to effectively scrape Single Page Applications.
What Are Headless Browsers?
Headless browsers operate like regular web browsers but without a graphical user interface (GUI). They allow you to automate browser actions programmatically, making them ideal for tasks such as web scraping and testing. Popular headless browsers include Puppeteer, Playwright, and Selenium.
Why Use Headless Browsers for SPA Scraping?
Single Page Applications rely heavily on JavaScript to load content dynamically, making traditional scraping methods ineffective. Headless browsers render pages just like a real user would, allowing them to interact with dynamic content and extract data efficiently.
Setting Up Your Environment
Before diving into the code, you’ll need to set up your development environment. This typically involves installing Node.js and npm (Node Package Manager), as most headless browsers are written in JavaScript.
Installing Puppeteer
Puppeteer is a popular choice for SPA scraping due to its ease of use and extensive feature set. To install Puppeteer, run the following command:
npm install puppeteer
Writing Your First Script
With Puppeteer installed, you can write your first headless browser script. Here’s a basic example to get you started:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
This script launches a headless instance of Chrome, navigates to https://example.com
, and takes a screenshot of the page.
Scraping Dynamic Content with Puppeteer
To scrape dynamic content from an SPA, you’ll need to interact with the page similarly to how a human would. This often involves waiting for elements to load, clicking buttons, and scrolling through pages.
Waiting for Elements to Load
Dynamic content might not be available immediately upon loading a page. To ensure your script waits for the necessary elements, use Puppeteer’s page.waitForSelector()
method:
await page.goto('https://example.com');
await page.waitForSelector('#dynamicContent'); // Wait for an element with ID 'dynamicContent'
const content = await page.$eval('#dynamicContent', el => el.innerText);
console.log(content);
Clicking Buttons and Interacting with the Page
Many SPAs load content upon user interaction, such as clicking a button or navigating through pagination. You can simulate these actions using Puppeteer:
await page.goto('https://example.com');
await page.waitForSelector('#nextButton');
await page.click('#nextButton'); // Click the 'next' button
await page.waitForNavigation(); // Wait for navigation to complete
const newContent = await page.$eval('#dynamicContent', el => el.innerText);
console.log(newContent);
Scrolling and Infinite Scroll Pages
Some SPAs load content as the user scrolls down the page. To handle these scenarios, you can use Puppeteer’s page.evaluate()
method to run JavaScript code in the context of the page:
await page.goto('https://example.com');
await page.evaluate(() => {
window.scrollTo(0, document.body.scrollHeight); // Scroll to the bottom of the page
});
await page.waitForSelector('#infiniteScrollContent');
const infiniteContent = await page.$eval('#infiniteScrollContent', el => el.innerText);
console.log(infiniteContent);
Advanced SPA Scraping Techniques
Handling Authentication
Many SPAs require user authentication to access their content. You can automate the login process using Puppeteer:
await page.goto('https://example.com/login');
await page.type('#username', 'your-username');
await page.type('#password', 'your-password');
await page.click('#loginButton');
await page.waitForNavigation();
const authenticatedContent = await page.$eval('#dynamicContent', el => el.innerText);
console.log(authenticatedContent);
Dealing with Captchas and Bot Detection
Captchas and bot detection mechanisms can pose challenges for automated scraping. While some techniques exist to bypass these protections, it’s essential to respect the website’s terms of service and robots.txt rules. Always prioritize ethical scraping practices.
Optimizing Your Scraping Script
Parallelization with Puppeteer Cluster
For large-scale scraping tasks, running multiple browser instances in parallel can significantly improve performance. Puppeteer Cluster is a library designed to facilitate this:
npm install puppeteer-cluster
Here’s an example of how to use it:
const cluster = require('puppeteer-cluster');
(async () => {
await cluster.launch({
concurrency: cluster.CONCURRENCY_CONTEXT, // Run multiple browsers in parallel
maxConcurrency: 4, // Limit the number of browsers to 4
});
await cluster.task(async ({ page }) => {
await page.goto('https://example.com');
const content = await page.$eval('#dynamicContent', el => el.innerText);
console.log(content);
});
})();
Error Handling and Retries
Web scraping is prone to errors, such as network issues or changes in the target website’s structure. Implementing robust error handling and retries can enhance the reliability of your scripts:
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
for (let i = 0; i < 3; i++) { // Retry up to 3 times
try {
await page.goto('https://example.com');
const content = await page.$eval('#dynamicContent', el => el.innerText);
console.log(content);
break; // Exit the loop if successful
} catch (error) {
console.error(`Attempt ${i + 1} failed:`, error);
await new Promise(resolve => setTimeout(resolve, 2000)); // Wait for 2 seconds before retrying
}
}
await browser.close();
})();
Conclusion
Scraping Single Page Applications (SPA) with headless browsers like Puppeteer is a powerful technique that enables you to extract dynamic content effectively. By following the best practices outlined in this guide, you can write robust and efficient scraping scripts tailored to your specific needs. Whether you’re scraping data for research purposes or automating workflows, headless browsers offer a versatile and reliable solution for modern web scraping tasks.
FAQs
What is the difference between headless browsers and traditional scraping tools? Headless browsers render JavaScript and dynamically load content, making them suitable for SPAs. Traditional scraping tools often struggle with dynamic content.
Can I use Puppeteer on Windows? Yes, Puppeteer supports Windows, macOS, and Linux. Ensure you have Node.js installed to run Puppeteer scripts.
How can I handle CAPTCHAs with headless browsers? While some techniques exist (e.g., using captcha-solving services), it’s crucial to respect the website’s terms of service and use ethical scraping practices.
What is the best way to extract data from an infinite scroll page? Use Puppeteer’s
page.evaluate()
method to simulate user scrolling and wait for new content to load before extracting it.Can I run multiple browser instances in parallel with Puppeteer? Yes, you can use libraries like Puppeteer Cluster to launch multiple browsers concurrently, improving the performance of your scraping tasks.