· Charlotte Will · 5 min read
Python Webscraping 101: Getting Started with Scraping Data
Python Web Scraping 101: Learn how to extract data from websites using Python with this beginner-friendly guide. Discover step-by-step tutorials, best practices, and essential tools like Beautiful Soup and Scrapy. Start scraping today!
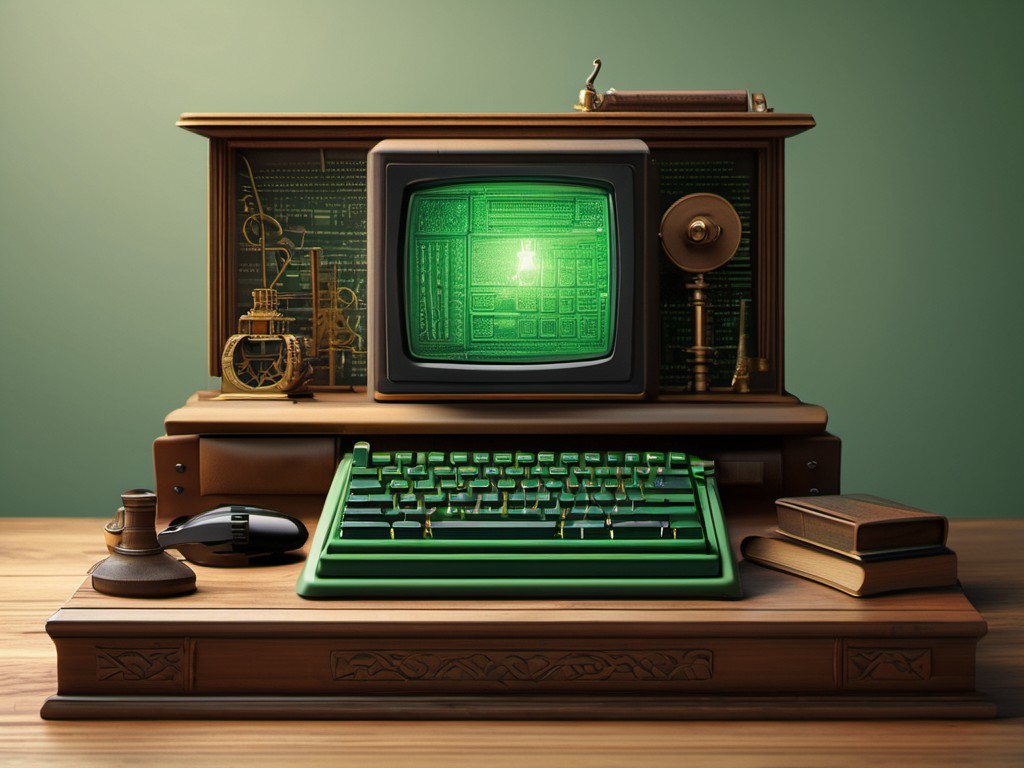
Introduction to Python Web Scraping
Web scraping is an essential skill for data scientists, developers, and anyone interested in extracting information from websites. In simple terms, web scraping involves writing programs that automatically visit websites and extract the desired data. Python, with its rich ecosystem of libraries, is one of the best programming languages to learn web scraping.
What is Web Scraping?
Web scraping is a technique used to extract data from websites by sending HTTP requests to the server and parsing the HTML responses. It automates the process of collecting information that would otherwise require manual effort.
Why Use Python for Web Scraping?
Python is widely used for web scraping due to its simplicity, readability, and the availability of powerful libraries such as Beautiful Soup, Scrapy, and Selenium. These tools make it easier to handle HTTP requests, parse HTML content, and manage large datasets.
Setting Up Your Environment
Before diving into web scraping, you need to set up your development environment. Here’s a step-by-step guide:
- Install Python: Download and install the latest version of Python from python.org.
- Set Up a Virtual Environment: Use
venv
orvirtualenv
to create an isolated environment for your project.python -m venv myenv source myenv/bin/activate # On Windows, use `myenv\Scripts\activate`
- Install Required Libraries: Install the necessary libraries using pip.
pip install requests beautifulsoup4 scrapy
Understanding HTML Structure
HTML (HyperText Markup Language) is the standard language used to create web pages. Understanding the structure of HTML is crucial for effective web scraping. Here are some basic tags you should be familiar with:
<html>
: The root element of an HTML document.<head>
: Contains meta-information about the document (e.g., title, character set).<body>
: Contains the content visible to the user (e.g., text, images, links).<div>
,<span>
: Used for grouping and styling HTML elements.<a>
: Defines a hyperlink.<h1>
to<h6>
: Headings of different levels.<p>
: Paragraphs.<table>
,<tr>
,<td>
: Tables and their rows/cells.
Using Beautiful Soup for Basic Scraping
Beautiful Soup is a popular library for parsing HTML and XML documents. It creates a parse tree from the page’s source code, which can then be used to extract data in a hierarchical manner.
Installing and Importing Libraries
First, install Beautiful Soup:
pip install beautifulsoup4
Then, import the required libraries in your Python script:
import requests
from bs4 import BeautifulSoup
Parsing HTML Content
Here’s a simple example of how to use Beautiful Soup to scrape data from a website:
- Send an HTTP request using the
requests
library. - Parse the response content with Beautiful Soup.
- Extract the desired data by navigating through the parse tree.
# Send a GET request to the webpage
response = requests.get('https://example.com')
# Parse the HTML content using BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
# Extract data
headlines = soup.find_all('h1')
for headline in headlines:
print(headline.text)
Advanced Techniques with Scrapy
Scrapy is a powerful and flexible web scraping framework built on top of Twisted, an asynchronous networking library written in Python. It’s ideal for scraping large websites efficiently.
Introduction to Scrapy
Unlike Beautiful Soup, which is more suitable for small-scale projects, Scrapy is designed for robust, industrial-strength web crawling and scraping. It comes with built-in support for handling cookies, sessions, and other complexities of modern web scraping.
Writing a Scrapy Spider
A Scrapy spider is the core component that defines how to follow links, extract data, and handle responses. Here’s an example of writing a simple Scrapy spider:
- Create a new Scrapy project:
scrapy startproject myproject cd myproject
- Generate a new spider:
scrapy genspider example_spider example.com
- Edit the generated spider file (
example_spider.py
) to include your scraping logic:import scrapy class ExampleSpider(scrapy.Spider): name = "example_spider" start_urls = ['http://example.com'] def parse(self, response): for headline in response.css('h1'): yield {'headline': headline.text} # Follow pagination links next_page = response.css('a.next-page::attr(href)').get() if next_page: yield response.follow(next_page, self.parse)
- Run the spider:
scrapy crawl example_spider -o output.json
Ethical Considerations in Web Scraping
While web scraping can be immensely beneficial, it’s essential to consider ethical and legal implications:
- Respect Robots.txt: Most websites have a
robots.txt
file that specifies which pages are allowed to be crawled by bots. Always check this file before starting your web scraping project. - Avoid Overloading Servers: Make sure to include delays between requests to prevent overwhelming the server with too many requests at once.
- Comply with Legal Requirements: Familiarize yourself with the legal requirements and terms of service for the websites you plan to scrape.
Common Challenges and Solutions
Dynamic Content Loading
Some websites load content dynamically using JavaScript. In such cases, tools like Selenium can be used to render JavaScript before extracting data.
from selenium import webdriver
from bs4 import BeautifulSoup
# Initialize the Selenium WebDriver (e.g., for Chrome)
driver = webdriver.Chrome()
driver.get('https://example.com')
# Wait for JavaScript to load content
time.sleep(5)
# Extract data with Beautiful Soup
soup = BeautifulSoup(driver.page_source, 'html.parser')
headlines = soup.find_all('h1')
for headline in headlines:
print(headline.text)
# Close the driver
driver.quit()
Handling Captchas
Captchas are used to prevent automated access to websites. While there’s no straightforward solution, you can often bypass captchas by using proxies or manually solving them for initial requests.
Conclusion
Web scraping is a powerful technique that allows you to extract valuable data from the web. With Python and libraries like Beautiful Soup and Scrapy, you have all the tools needed to get started. Always remember to use these techniques ethically and responsibly.
Happy scraping!
FAQs
What are some common uses of web scraping? Web scraping is used for a variety of purposes, including data analysis, price monitoring, lead generation, and content aggregation.
How can I handle rate limiting while web scraping? Rate limiting can be managed by adding delays between requests using libraries like
time
in Python, or more advanced techniques such as rotating proxies and user agents.What is the difference between Beautiful Soup and Scrapy? Beautiful Soup is a lightweight library for parsing HTML and XML documents, ideal for simple and small-scale scraping projects. Scrapy, on the other hand, is a full-fledged web crawling framework suitable for large-scale and complex scraping tasks.
How do I handle JavaScript-rendered content? For handling JavaScript-rendered content, tools like Selenium can be used to render JavaScript before extracting data with libraries such as Beautiful Soup.
What should I include in my robots.txt file? Your
robots.txt
file should specify which parts of your website are allowed or disallowed for web crawlers. It typically includes directives likeUser-agent
,Allow
, andDisallow
.