· Charlotte Will · webscraping · 6 min read
Mastering Scrapy for Large-Scale Web Scraping Projects
Mastering Scrapy for large-scale web scraping projects involves setting up Scrapy, optimizing performance, handling rate limits, implementing middleware, using proxies, and managing errors effectively. This guide provides practical tips and strategies to help you extract data efficiently and ethically.
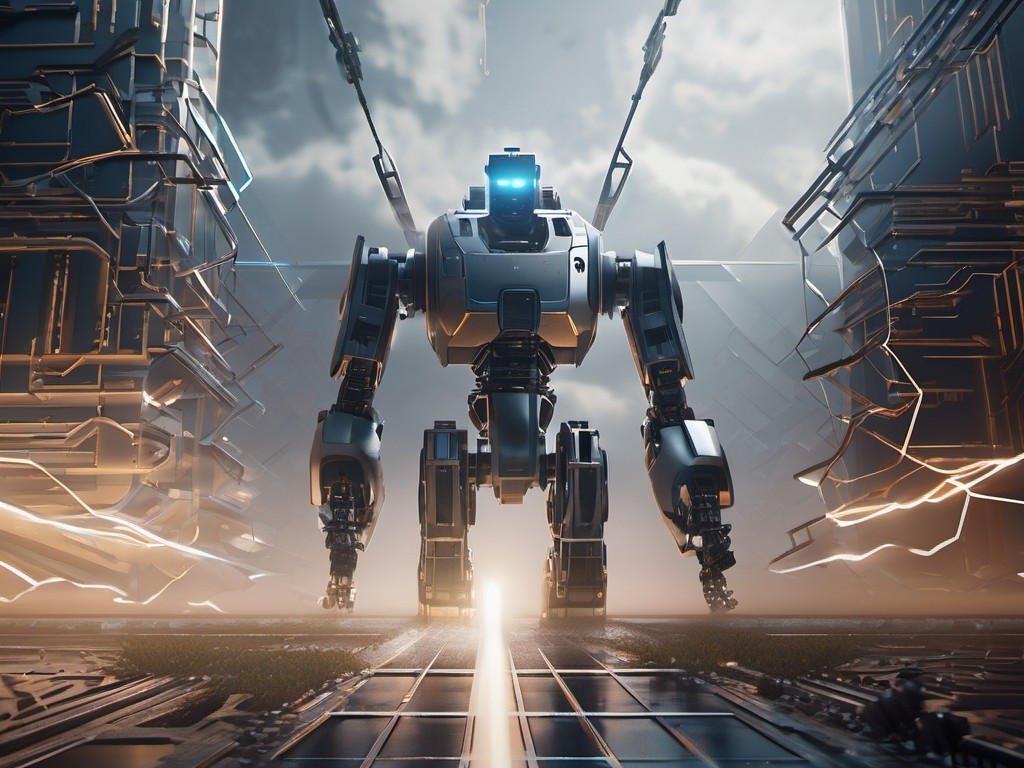
Web scraping has become an essential tool in the digital age, allowing businesses to gather valuable data from websites. When it comes to large-scale web scraping projects, Scrapy stands out as a powerful and flexible framework written in Python. This article will guide you through setting up Scrapy for large-scale projects, optimizing performance, handling rate limits, implementing middleware, using proxies, error handling, and avoiding common pitfalls.
Why Choose Scrapy for Large-Scale Web Scraping?
Scrapy is renowned for its efficiency and scalability, making it an ideal choice for large-scale web scraping projects. Written in Python, Scrapy offers a robust set of features that enable you to extract data from websites quickly and effectively.
Key Features of Scrapy
- Asynchronous Processing: Scrapy’s asynchronous nature allows it to send multiple requests concurrently, improving the speed of data extraction significantly.
- Flexible Pipelines: With customizable pipelines, you can process and store extracted data according to your specific needs.
- Built-in Middleware: Scrapy comes with a range of built-in middlewares that handle tasks like cookies, caching, and compression automatically.
- Extensible: You can extend Scrapy’s functionality with custom middleware and downstream processors to suit your project requirements.
Setting Up Scrapy for Large-Scale Projects
To start using Scrapy for large-scale projects, you’ll need to install it and set up a basic spider. Here are the steps:
Installation
First, make sure Python is installed on your system. Then, install Scrapy using pip:
pip install scrapy
Creating a New Project
Create a new directory for your project and navigate into it:
mkdir myproject
cd myproject
Initialize a new Scrapy project:
scrapy startproject myproject
Creating Your First Spider
Generate a new spider with the following command:
scrapy genspider example_spider example.com
This will create a spider called example_spider.py
, which you can customize to fit your scraping needs.
Optimizing Scrapy for High-Performance Web Scraping
To ensure optimal performance in large-scale web scraping projects, consider the following strategies:
Concurrency Settings
Scrapy allows you to control concurrency through several settings:
# settings.py
CONCURRENT_REQUESTS = 16
CONCURRENT_REQUESTS_PER_DOMAIN = 8
CONCURRENT_REQUESTS_PER_IP = 2
Adjust these values based on your target website’s capabilities and your network bandwidth.
Download Delays
Setting a download delay can help prevent overloading the server:
# settings.py
DOWNLOAD_DELAY = 1
RANDOMIZE_DOWNLOAD_DELAY = True
Randomizing the download delay makes your requests appear more human-like, reducing the risk of being blocked.
Handling Rate Limits in Scrapy
Rate limiting is crucial to avoid overwhelming target servers and getting blocked. Here are some strategies:
Using AutoThrottle Extension
Scrapy’s autothrottle
extension automatically adjusts the delay between requests based on the server’s response time:
# settings.py
EXTENSIONS = {
'scrapy.extensions.telnet.TelnetConsole': None,
'scrapy.extensions.autothrottle.AutoThrottleExtension': 2000, # Max download delay (seconds)
}
Implementing Custom Rate Limiting
For more control, you can implement custom rate limiting logic:
import time
from scrapy import signals
from scrapy.utils.log import configure_logging
class CustomRateLimiterMiddleware(object):
@classmethod
def from_crawler(cls, crawler):
s = cls()
crawler.signals.connect(s.spider_opened, signal=signals.spider_opened)
return s
def spider_opened(self, spider):
spider.log('Rate limiter middleware enabled')
spider.crawled = 0
spider.max_crawl_rate = 10 # Adjust this value based on your needs
def process_request(self, request, spider):
time.sleep(1 / spider.max_crawl_rate)
spider.crawled += 1
Add the middleware to your settings:
# settings.py
DOWNLOADER_MIDDLEWARES = {
'myproject.middlewares.CustomRateLimiterMiddleware': 543,
}
Implementing Middleware in Scrapy
Middleware components let you extend and customize Scrapy’s behavior. Here’s how to create and use middleware:
Creating Custom Middleware
Define your middleware in a new file (e.g., middleware.py
):
class MyCustomMiddleware(object):
def process_request(self, request, spider):
# Add custom logic here
return None
def process_response(self, request, response, spider):
# Add custom logic here
return response
Enabling Middleware
Enable your middleware in the settings.py
file:
# settings.py
DOWNLOADER_MIDDLEWARES = {
'myproject.middlewares.MyCustomMiddleware': 543,
}
Using Proxies for Anonymous Web Scraping with Scrapy
Proxies help you rotate IP addresses to avoid being blocked by target servers. Here’s how to configure proxies in Scrapy:
Configuring Proxy Settings
Add your proxy settings in settings.py
:
# settings.py
PROXY_POOL_PAGE = 100
DOWNLOADER_MIDDLEWARES = {
'scrapy.downloadermiddlewares.httpproxy.HttpProxyMiddleware': 1,
}
HTTP_PROXY_POOL_ENABLED = True
Rotating Proxies
Use a proxy pool to rotate proxies automatically:
# middleware.py
import requests
from scrapy import signals
from scrapy.utils.log import configure_logging
class ProxyPoolMiddleware(object):
@classmethod
def from_crawler(cls, crawler):
s = cls()
crawler.signals.connect(s.spider_opened, signal=signals.spider_opened)
return s
def spider_opened(self, spider):
spider.log('Proxy pool middleware enabled')
def process_request(self, request, spider):
response = requests.get("http://your-proxy-pool-api/")
proxy = response.json()["proxy"] # Assuming the API returns a JSON object with a "proxy" field
request.meta['proxy'] = proxy
Add your middleware to settings.py
:
# settings.py
DOWNLOADER_MIDDLEWARES = {
'myproject.middlewares.ProxyPoolMiddleware': 543,
}
Error Handling in Scrapy
Effective error handling is crucial to ensure the stability of your scraping projects. Here are some strategies:
Retry Mechanism
Scrapy includes a built-in retry mechanism that can handle transient errors:
# settings.py
RETRY_TIMES = 5
RETRY_HTTP_CODES = [500, 502, 503, 504, 429]
Custom Error Handling
For more complex error handling, you can create custom middleware:
class CustomErrorHandlerMiddleware(object):
def process_exception(self, request, exception, spider):
# Add custom logic here to handle exceptions
return None
Avoiding Common Pitfalls in Large-Scale Web Scraping Projects
Here are some common pitfalls and how to avoid them:
Overloading the Server
Be mindful of your scraping rate. Too many requests too quickly can overload the server and get you blocked. Use rate limiting and concurrency settings to manage this.
Handling Dynamic Content
Modern websites use a lot of JavaScript to load content dynamically. Scrapy doesn’t handle JavaScript out of the box, so consider using tools like Selenium or Playwright in conjunction with Scrapy for such cases.
Respecting Robots.txt
Always check the robots.txt
file of the website you’re scraping to ensure you’re respecting their rules and not scraping disallowed pages.
Conclusion
Scrapy is a powerful tool for large-scale web scraping projects, offering flexibility, scalability, and performance optimization out of the box. By setting up Scrapy correctly, optimizing its performance, handling rate limits, implementing middleware, using proxies, managing errors effectively, and avoiding common pitfalls, you can extract data efficiently and ethically.
FAQs
What is Scrapy and why should I use it for large-scale web scraping?
Scrapy is an open-source web crawling framework written in Python. It’s ideal for large-scale web scraping due to its asynchronous nature, flexibility, and robust set of features.
How can I optimize Scrapy for high-performance web scraping?
Optimize Scrapy by adjusting concurrency settings, using download delays, implementing rate limiting, and utilizing middleware effectively.
What is the best way to handle rate limits in Scrapy?
Use Scrapy’s autothrottle
extension or implement custom rate limiting logic to manage rate limits effectively.
How can I use proxies for anonymous web scraping with Scrapy?
Configure proxy settings in your settings.py
file and use a proxy pool to rotate proxies automatically.
What are some common pitfalls to avoid in large-scale web scraping projects?
Avoid overloading the server, handle dynamic content properly, respect robots.txt
, and implement effective error handling strategies.