· Charlotte Will · 5 min read
Making API Calls in Python: A How-to Guide for Web Scrapers
Learn how to make efficient API calls in Python for web scraping, including handling rate limits and errors, managing authentication, and best practices for secure coding. Enhance your data extraction skills with this comprehensive guide.
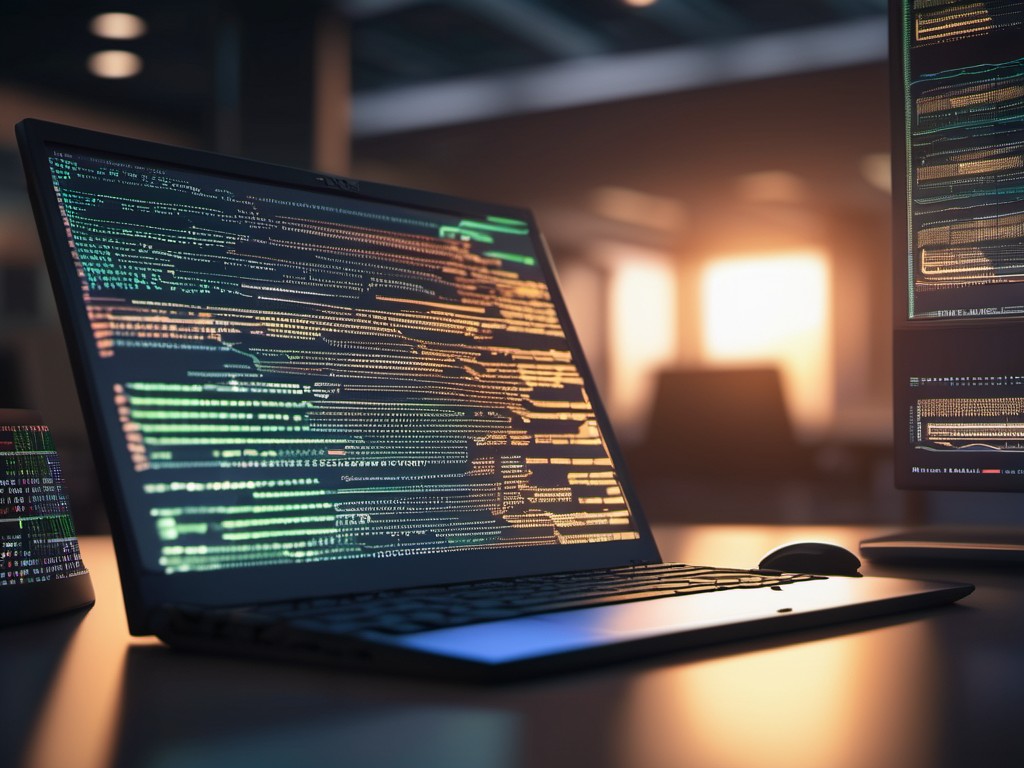
Web scraping is a powerful technique used to extract data from websites, but sometimes it can be challenging and even against the terms of service of certain platforms. This is where APIs (Application Programming Interfaces) come into play. APIs provide a legal and structured way to access data programmatically. In this guide, we’ll walk you through making API calls in Python, perfect for web scrapers looking to enhance their skills.
Introduction to APIs and Web Scraping
What is an API?
An API, or Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate with each other. In the context of web scraping, APIs can be particularly useful because they offer a legal way to access data without having to parse HTML directly.
Why Use APIs for Web Scraping?
Using APIs for web scraping offers several advantages:
- Legal Compliance: By using APIs, you’re less likely to violate the terms of service of a website.
- Efficiency: APIs often provide data in a structured format (like JSON), making it easier to parse and use.
- Reliability: APIs are designed for programmatic access and are more reliable than scraping, which can break if a site’s layout changes.
Setting Up Your Environment
Installing Required Libraries
Before you start making API calls in Python, you need to install some essential libraries. The most popular library for this purpose is requests
. You can install it using pip:
pip install requests
Basic Python Syntax Overview
If you’re new to Python, here’s a quick overview of the basic syntax you’ll need to know:
- Variables:
name = "John"
- Functions:
def greet(name): return f"Hello, {name}!"
- Loops:
for i in range(10): print(i)
- Conditionals:
if x > 0: print("Positive")
Making Simple API Calls
Using the Requests Library
The requests
library is incredibly user-friendly and makes it easy to send HTTP requests. Here’s a basic example of how to make a GET request:
import requests
response = requests.get('https://api.example.com/data')
print(response.status_code) # Check if the request was successful
print(response.json()) # Parse and print the JSON response
Handling Responses
After you make an API call, you’ll need to handle the response. This typically involves checking the status code and parsing the data. Here’s how you can do it:
if response.status_code == 200:
data = response.json()
# Process your data here
else:
print(f"Error: {response.status_code}")
Advanced Techniques for Efficient Web Scraping
Managing Rate Limits
APIs often have rate limits to prevent abuse. It’s crucial to handle these limits gracefully. You can use the time.sleep()
function to pause your script until you’re allowed to make another request:
import time
response = requests.get('https://api.example.com/data')
if "X-RateLimit-Remaining" in response.headers:
remaining_calls = int(response.headers["X-RateLimit-Remaining"])
if remaining_calls == 0:
retry_after = int(response.headers["X-RateLimit-Reset"]) - time.time()
print(f"Rate limit hit, retrying in {retry_after} seconds")
time.sleep(retry_after)
Error Handling and Retries
It’s a good practice to include error handling in your code. You can use the try-except
block to catch exceptions and implement retries:
import requests
from requests.exceptions import RequestException
for attempt in range(3):
try:
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json()
break
except RequestException as e:
print(f"Attempt {attempt + 1} failed: {e}")
Common Mistakes When Using APIs for Web Scraping
Not Checking Response Status Codes
Always check the response status codes to ensure that your requests are successful. A 200 OK
status code indicates a successful request, while other codes (like 404 Not Found
or 500 Internal Server Error
) indicate something went wrong.
Ignoring Rate Limits
Ignoring rate limits can lead to your IP being blocked by the API provider. Always respect the rate limits and handle them appropriately.
Not Including Error Handling
Errors are a part of programming, and it’s crucial to include error handling in your code to make it more robust.
Best Practices for Making API Calls in Python
Use Environment Variables for API Keys
Storing API keys directly in your code is not secure. Instead, use environment variables:
import os
api_key = os.getenv('API_KEY')
response = requests.get(f'https://api.example.com/data?api_key={api_key}')
Log Your Requests and Responses
Logging can be extremely helpful for debugging and monitoring your API calls:
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger()
response = requests.get('https://api.example.com/data')
logger.info(f"Request URL: {response.url}")
logger.info(f"Response Status Code: {response.status_code}")
logger.info(f"Response Data: {response.json()}")
Conclusion
Making API calls in Python is a powerful skill for web scrapers, providing legal and efficient access to data. By following the steps outlined in this guide, you can make simple and advanced API requests, handle responses, manage rate limits, and implement error handling. Always remember to respect the terms of service of APIs and use best practices to ensure your code is secure and robust.
FAQs
What are the best libraries for making API calls in Python?
The requests
library is the most popular and user-friendly library for making HTTP requests in Python. Other notable libraries include httpx
(for more advanced features) and aiohttp
(for asynchronous HTTP requests).
How do I handle authentication with APIs?
Authentication methods vary depending on the API. Common methods include API keys, OAuth, and Bearer tokens. Always refer to the API documentation for specific authentication requirements.
What should I do if I hit an API rate limit?
If you hit an API rate limit, you can either wait until the rate limit resets or implement a retry mechanism with exponential backoff (increasing the delay between retries) in your code.
Are there any legal considerations when scraping data from APIs?
Yes, it’s crucial to read and understand the terms of service of any API you use. Some APIs may require you to sign up for an account or agree to specific usage conditions. Always respect the API provider’s rules to avoid legal issues.
How can I ensure my code is secure when making API calls?
To ensure your code is secure, store API keys in environment variables rather than hardcoding them into your scripts. Use HTTPS for all requests and implement proper error handling and logging to monitor and debug your API interactions.