· Charlotte Will · webscraping · 5 min read
Leveraging Headless Browsers for Scraping Complex JavaScript Sites
Discover how to leverage headless browsers like Puppeteer and Selenium for scraping complex JavaScript sites. Learn practical steps, code examples, and best practices for extracting dynamic content efficiently. Optimize your web scraping projects today!
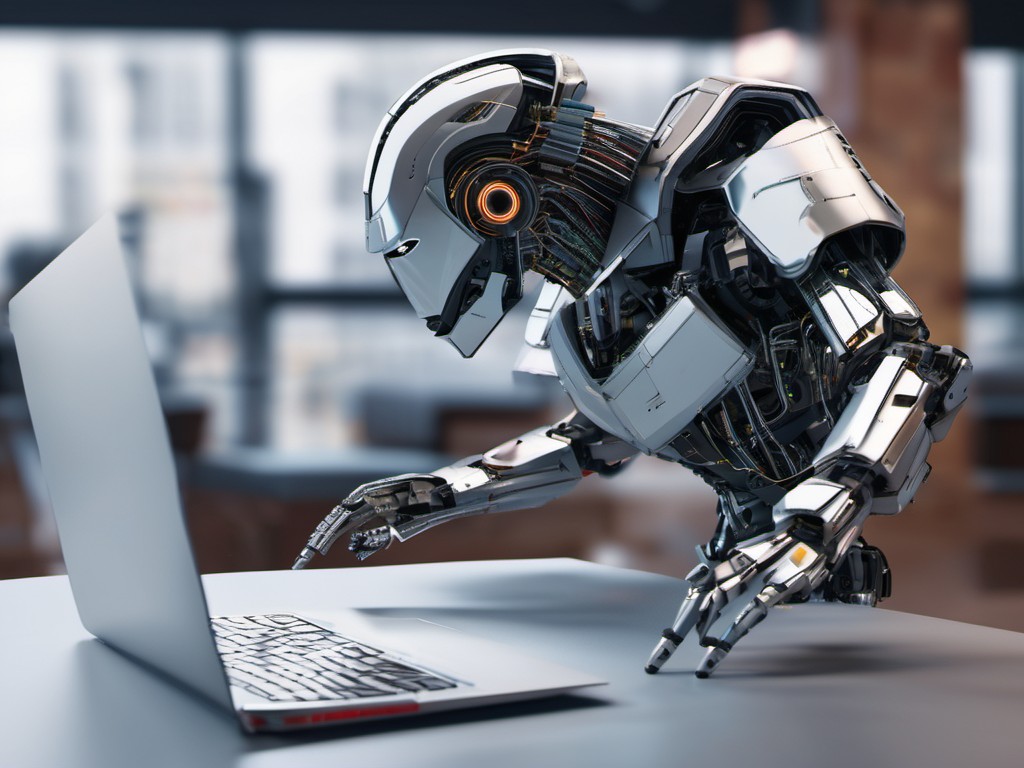
In the dynamic world of web development, JavaScript has become ubiquitous, powering everything from simple interactions to complex single-page applications (SPA). While this enhances user experience, it also poses significant challenges for traditional web scraping techniques. Enter headless browsers: a powerful tool that allows you to automate browser interactions and extract data from even the most intricate JavaScript-heavy websites.
What are Headless Browsers?
Headless browsers are web browsers without a graphical user interface, designed specifically for automated testing and scraping. They simulate real browser behavior but run in the background, making them ideal for tasks that require interaction with modern, JavaScript-heavy websites. Popular headless browsers include Puppeteer and Selenium, which offer robust APIs to control and manipulate web pages programmatically.
Why Use Headless Browsers?
Traditional scraping tools often struggle with modern websites that rely heavily on JavaScript for content rendering. Headless browsers overcome this limitation by:
- Rendering JavaScript: They execute JavaScript code, allowing you to scrape dynamically generated content.
- Simulating User Interactions: You can simulate mouse clicks, keyboard inputs, and other user interactions necessary to access hidden data.
- Handling Complex Interfaces: Ideal for SPA where content loads asynchronously through AJAX calls or frameworks like React, Angular, and Vue.js.
Setting Up Headless Browsers
Prerequisites
Before diving into the technical aspects, ensure you have:
- Node.js: Most headless browsers require Node.js for installation and execution.
- npm (Node Package Manager): To install necessary packages and dependencies.
- Basic Knowledge of JavaScript/TypeScript: Familiarity with these languages will help you write effective scraping scripts.
Installation
Puppeteer
Puppeteer is a popular headless browser built on the Chromium engine, maintained by the Chrome DevTools team.
npm install puppeteer
Selenium
Selenium supports multiple browsers (Chrome, Firefox) and offers more extensive testing capabilities.
npm install selenium-webdriver chromedriver
Scraping with Puppeteer
Basic Setup
Create a new JavaScript file (e.g., scraper.js
) and set up your environment:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Your scraping code here
await browser.close();
})();
Rendering JavaScript
Puppeteer waits for the page to fully render before executing any scripts:
await page.goto('https://example.com');
await page.waitForSelector('.dynamic-content');
const content = await page.$eval('.dynamic-content', el => el.innerText);
console.log(content);
Interacting with the Page
Simulate user actions to extract hidden data:
await page.click('#show-more-button');
await page.waitForSelector('.additional-content');
const additionalContent = await page.$eval('.additional-content', el => el.innerText);
console.log(additionalContent);
Scraping with Selenium
Basic Setup
Initialize Selenium with a headless browser:
const { Builder, By, until } = require('selenium-webdriver');
let driver = new Builder().forBrowser('chrome').setChromeOption('headless').build();
(async function example() {
try {
let driver = await new Builder().forBrowser('chrome').setChromeOption('headless').build();
await driver.get('https://example.com');
// Your scraping code here
await driver.quit();
} catch (error) {
console.error(error);
}
})();
Rendering JavaScript
Wait for specific elements to load:
await driver.get('https://example.com');
let dynamicElement = await driver.wait(until.elementLocated(By.css('.dynamic-content')), 10000);
let content = await dynamicElement.getText();
console.log(content);
Interacting with the Page
Automate clicks and other user actions:
let showMoreButton = await driver.findElement(By.id('show-more-button'));
await showMoreButton.click();
let additionalContent = await driver.wait(until.elementLocated(By.css('.additional-content')), 10000);
content = await additionalContent.getText();
console.log(content);
Handling CAPTCHAs and Bot Detection
One common challenge with headless browsers is bot detection mechanisms like CAPTCHAs. To circumvent this:
- Use Proxies: Rotate IP addresses to avoid detection.
- Emulate Real User Behavior: Randomize delays between actions, simulate mouse movements, and adjust browser settings (e.g., screen resolution, user agent).
- Solve CAPTCHAs Programmatically: Utilize third-party services like 2Captcha or Anti-Captcha.
Optimizing Performance
Resource Management
Headless browsers can be resource-intensive. Optimize performance by:
- Reusing Browser Instances: Avoid launching a new browser for each request.
- Parallel Processing: Use libraries like
async
orPromise.all
to run multiple scraping tasks concurrently. - Memory Management: Clear cache and close unnecessary tabs/pages regularly.
Error Handling
Implement robust error handling to manage network issues, timeouts, and unexpected page behavior:
try {
await page.goto('https://example.com');
} catch (error) {
console.error('Page load error:', error);
// Handle the error or retry
}
Best Practices
- Respect Robots.txt: Always check a website’s
robots.txt
file to understand what content can be scraped. - Ethical Scraping: Avoid overloading servers and respect the site’s terms of service.
- Data Storage: Store scraped data efficiently using databases or cloud storage solutions.
- Logging and Monitoring: Implement logging to track scraping progress and monitor for errors.
- Regular Updates: Keep your headless browser and dependencies up to date to benefit from the latest features and security patches.
Conclusion
Headless browsers have revolutionized web data extraction, enabling you to scrape even the most complex JavaScript-driven websites effectively. Whether using Puppeteer or Selenium, these tools offer powerful APIs and extensive customization options. By adhering to best practices and optimizing performance, you can harness the full potential of headless browsers for your web scraping projects.
FAQs
What is a headless browser? A headless browser is a web browser without a graphical user interface, designed for automated testing and scraping. It simulates real browser behavior but runs in the background, making it ideal for tasks that require interaction with modern, JavaScript-heavy websites.
Why use headless browsers for web scraping? Headless browsers render JavaScript, simulate user interactions, and handle complex interfaces, allowing you to extract data from dynamic content effectively.
Which is better: Puppeteer or Selenium? Both have their strengths. Puppeteer is lightweight and specifically designed for headless Chrome, while Selenium supports multiple browsers and offers more extensive testing capabilities. Choose based on your specific needs and preferences.
How do I handle CAPTCHAs with headless browsers? You can use proxies to rotate IP addresses, emulate real user behavior, or utilize third-party services like 2Captcha or Anti-Captcha to solve CAPTCHAs programmatically.
What are some best practices for optimizing headless browser performance? Reuse browser instances, use parallel processing, clear cache regularly, and implement robust error handling. Also, always respect the
robots.txt
file and practice ethical scraping.