· Charlotte Will · Amazon API · 6 min read
How to Use the Amazon Product Advertising API for Data Extraction
Learn how to use the Amazon Product Advertising API for data extraction with this comprehensive guide. Discover step-by-step instructions, sample code examples, and best practices for effective API usage. Ideal for developers looking to extract product data from Amazon's vast catalog.
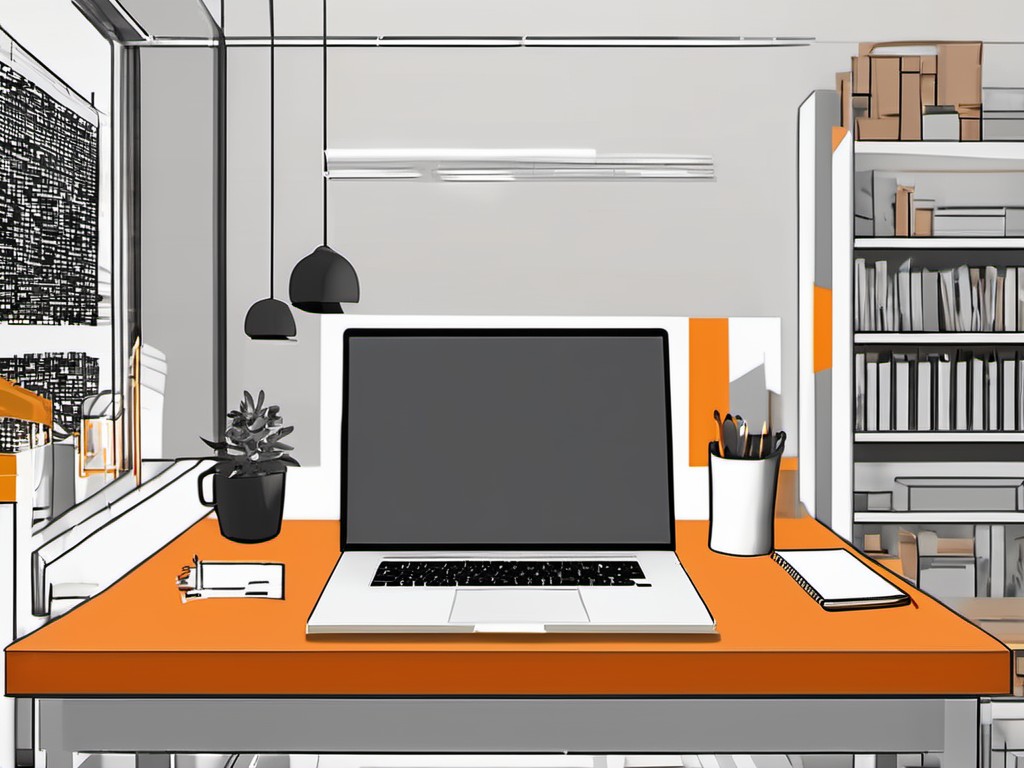
If you’re looking to extract product data from Amazon, the Amazon Product Advertising API is a powerful tool that can help you achieve this. This comprehensive guide will walk you through everything you need to know about using the Amazon Product Advertising API for data extraction. Whether you’re a beginner or an intermediate user, we’ve got you covered with practical, step-by-step guidance.
Introduction to Amazon Product Advertising API
The Amazon Product Advertising API allows developers to access Amazon’s product catalog and retrieve detailed information about products. This can be incredibly useful for e-commerce websites, price comparison tools, affiliate marketing, and more. By leveraging this API, you can extract valuable data such as product descriptions, prices, images, reviews, and much more.
Setting Up Your Environment
Before diving into the specifics of making API requests, it’s essential to set up your environment correctly. This involves several steps:
Prerequisites
- Basic Programming Knowledge: Familiarity with a programming language such as Python, JavaScript, or PHP is necessary for implementing the API.
- Amazon Developer Account: You need to have an Amazon Associates account and register for AWS (Amazon Web Services).
- API Keys: Obtain your Access Key ID and Secret Access Key from AWS.
Registering for AWS Access
- Sign Up for Amazon Associates: If you haven’t already, sign up for the Amazon Associates program.
- Create an AWS Account: Go to the AWS Management Console and create a new account if you don’t have one.
- Generate API Keys: Once your AWS account is set up, navigate to the IAM (Identity and Access Management) dashboard to generate your Access Key ID and Secret Access Key.
Making API Requests
With your environment set up, you’re ready to start making API requests. Here are the key steps involved:
Authentication and Authorization
To authenticate your API requests, you need to sign them using your AWS credentials. This process involves creating a signature that verifies your identity.
- Create a Canonical Request: This includes the HTTP method, URI, query string parameters, headers, and payload (if any).
- Create a String to Sign: This combines the canonical request with additional information such as the current timestamp and region.
- Calculate the Signature: Use your Secret Access Key to create an HMAC-SHA256 signature of the string to sign.
- Add the Authorization Header: Include the calculated signature in the
Authorization
header of your API request.
Sample Code Examples
Here’s a simple example using Python and the requests
library:
import requests
from datetime import datetime
import hashlib
import hmac
def sign(key, msg):
return hmac.new(key, msg.encode('utf-8'), hashlib.sha256).digest()
def get_signature_key(key, dateStamp, regionName, serviceName):
kDate = sign(('AWS4' + key).encode('utf-8'), dateStamp)
kRegion = sign(kDate, regionName)
kService = sign(kRegion, serviceName)
kSigning = sign(kService, 'aws4_request')
return kSigning
access_key = 'YOUR_ACCESS_KEY'
secret_key = 'YOUR_SECRET_KEY'
region = 'us-east-1'
service = 'execute-api'
host = 'webservices.amazon.com'
endpoint = f"https://{host}/onca/xml"
method = 'GET'
canonical_uri = '/onca/xml'
canonical_querystring = ''
canonical_headers = 'host:' + host + '\n'
signed_headers = 'host'
payload_hash = hashlib.sha256(('').encode('utf-8')).hexdigest()
t = datetime.utcnow()
amzdate = t.strftime('%Y%m%dT%H%M%SZ')
datestamp = t.strftime('%Y%m%d') # Date w/o time, used in credential scope
canonical_request = method + '\n' + canonical_uri + '\n' + canonical_querystring + '\n' + canonical_headers + '\n' + signed_headers + '\n' + payload_hash
algorithm = 'AWS4-HMAC-SHA256'
credential_scope = datestamp + '/' + region + '/' + service + '/' + 'aws4_request'
string_to_sign = algorithm + '\n' + amzdate + '\n' + credential_scope + '\n' + hashlib.sha256(canonical_request.encode('utf-8')).hexdigest()
signing_key = get_signature_key(secret_key, datestamp, region, service)
signature = hmac.new(signing_key, (string_to_sign).encode('utf-8'), hashlib.sha256).hexdigest()
authorization_header = algorithm + ' ' + 'Credential=' + access_key + '/' + credential_scope + ', ' + 'SignedHeaders=' + signed_headers + ', ' + 'Signature=' + signature
headers = {'x-amz-date': amzdate, 'Authorization': authorization_header}
response = requests.get(endpoint, headers=headers)
print(response.content)
Extracting Product Data
Once your API request is authenticated and authorized, you can start extracting product data. Here’s how to understand the response structure and handle errors:
Understanding the Response Structure
Amazon Product Advertising API responses are typically in XML format. The response will include various nodes such as ItemAttributes
, OfferSummary
, EditorialReviews
, and more. Here’s a brief overview of some key elements:
- Title: The product title.
- ASIN: Amazon Standard Identification Number, a unique block of 10 letters and/or numbers that identify products.
- DetailPageURL: The URL to the product detail page on Amazon.
- ItemAttributes: Contains detailed information about the product such as brand, model, color, etc.
- OfferSummary: Provides pricing and availability information.
- EditorialReviews: Includes customer reviews and ratings.
Handling Errors and Limits
Errors can occur due to various reasons such as invalid parameters, quota limits, or service issues. Here are some common errors and how to handle them:
- InvalidParameterValue: Check your request parameters for typos or incorrect values.
- RequestThrottled: You’ve exceeded the rate limit. Implement exponential backoff and retry logic.
- InternalError: This is a server-side error. Retry after some time.
Best Practices for API Usage
To ensure efficient and effective use of the Amazon Product Advertising API, follow these best practices:
- Rate Limiting: Be aware of the rate limits imposed by Amazon. Typically, you can make up to 1 request per second.
- Caching: Cache responses to reduce the number of API calls and improve performance.
- Error Handling: Implement robust error handling to manage different types of errors gracefully.
- Pagination: For large datasets, use pagination to retrieve data in smaller chunks.
- Security: Protect your AWS credentials and never hardcode them in your application code. Use environment variables or secure vaults.
Conclusion
The Amazon Product Advertising API is a powerful tool for extracting product data from Amazon’s vast catalog. By following the steps outlined in this guide, you can set up your environment, make authenticated API requests, and effectively extract valuable product information. Whether you’re building an e-commerce site, a price comparison tool, or any other application that requires product data, mastering the Amazon Product Advertising API will give you a significant advantage.
FAQs
What are the rate limits for the Amazon Product Advertising API?
- The rate limit is typically 1 request per second, but this can vary. Always check the latest documentation from Amazon.
How do I handle pagination in API responses?
- Use the
ItemPage
parameter to specify the page number you want to retrieve. Increment this value to get subsequent pages of results.
- Use the
What should I do if I encounter a RequestThrottled error?
- Implement exponential backoff and retry logic. Wait for a short period before trying again, increasing the wait time with each attempt.
Can I use the Amazon Product Advertising API without an AWS account?
- No, you need to have an AWS account to generate the necessary API keys for authentication.
How can I extract product reviews using the Amazon Product Advertising API?
- You can retrieve customer reviews by including the
Reviews
node in your response group and parsing the XML response accordingly.
- You can retrieve customer reviews by including the