· Charlotte Will · 4 min read
How to Use Amazon Scraping API for E-commerce Data Extraction
Learn how to use Amazon Scraping API for efficient e-commerce data extraction. Discover best practices, tools, and code examples to automate product information gathering from Amazon.
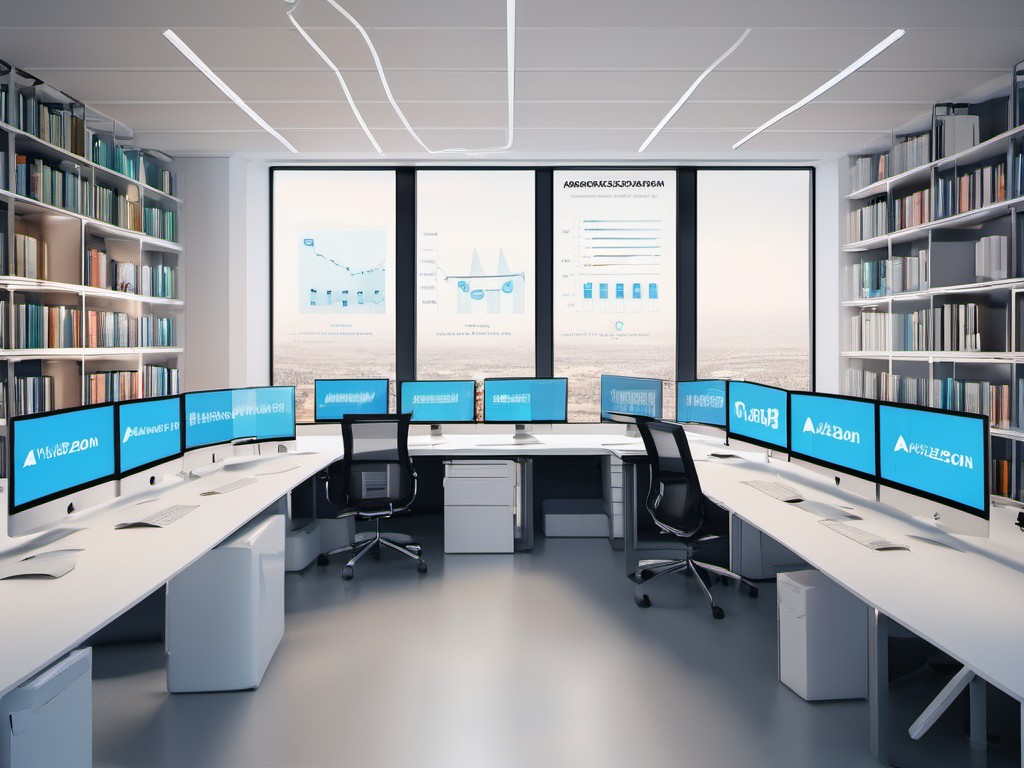
In today’s competitive e-commerce landscape, having access to up-to-date product information is crucial. This is where web scraping comes in—and more specifically, the Amazon Scraping API. By leveraging this tool, you can extract valuable data from Amazon, such as product details, prices, and reviews, to stay ahead of your competition. In this comprehensive guide, we’ll walk you through everything you need to know about using the Amazon Scraping API for e-commerce data extraction.
Understanding the Basics of Amazon Scraping API
What is Amazon Scraping API?
The Amazon Scraping API is a tool designed to extract product information from Amazon’s website in a structured format. Unlike manual web scraping, which can be time-consuming and error-prone, the API allows for automated extraction of data, making it an efficient solution for businesses and developers alike.
Benefits of Using an API for Data Extraction
- Efficiency: Automated data extraction saves time and effort compared to manual methods.
- Accuracy: APIs ensure that the data collected is accurate and consistent.
- Scalability: With APIs, you can extract large volumes of data efficiently.
- Compliance: Using an API ensures compliance with Amazon’s terms of service, reducing the risk of legal issues.
Setting Up Your Environment
Tools and Libraries Required
To get started with the Amazon Scraping API, you’ll need a few essential tools:
- A programming language (e.g., Python)
- HTTP client library (e.g.,
requests
in Python) - JSON processing library (e.g.,
json
in Python)
Step-by-Step Installation Guide
- Install Python: If you haven’t already, download and install Python from python.org.
- Set up a virtual environment: Create a virtual environment to isolate your project dependencies.
python -m venv env source env/bin/activate # On Windows use `env\Scripts\activate`
- Install required libraries: Use pip to install the necessary libraries.
pip install requests
Implementing Amazon Scraping API
Writing the Code
Here’s a basic example of how to use the Amazon Scraping API in Python:
import requests
# Define your API endpoint and parameters
api_url = "https://api.your-amazon-scraping-provider.com/products"
params = {
'keyword': 'laptop', # Example search term
'api_key': 'YOUR_API_KEY' # Replace with your actual API key
}
# Send a GET request to the API
response = requests.get(api_url, params=params)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Failed to retrieve data: {response.status_code}")
Handling Data Outputs
Once you’ve successfully extracted the data, you’ll need a way to handle and store it. Here are some tips:
- Data Cleaning: Remove any unnecessary information or formatting issues.
- Storage: Save the data in a database or a structured file format (e.g., CSV, JSON) for easy access and analysis.
- Analysis: Use data analysis tools to extract insights from the collected data.
Best Practices for E-commerce Data Extraction
Compliance with Terms of Service
Ensure that you comply with Amazon’s terms of service when using an API to scrape data. This often involves respecting rate limits and not overloading their servers.
Ethical Considerations
Be mindful of ethical considerations, such as respecting privacy and not misusing the extracted data.
Data Quality Assurance
Regularly check the quality and accuracy of the data you’re extracting to ensure it meets your needs.
Automation and Scheduling
Set up automated scripts to periodically extract data, ensuring that your information is always up-to-date.
FAQ Section
Is Amazon Scraping legal?
Yes, as long as you comply with Amazon’s terms of service and use ethical practices. However, always consult with a legal professional for advice tailored to your specific situation.
What are the limitations of using an API for data extraction?
Some limitations include rate limits set by the API provider, potential changes in Amazon’s website structure that could affect scraping accuracy, and the need for proper error handling.
How can I ensure the accuracy of extracted data?
By periodically verifying the data against the source and implementing checks to handle discrepancies or errors during extraction.
Can I scrape real-time pricing from Amazon?
Yes, many APIs offer real-time price extraction capabilities. Ensure your API provider supports this feature and check their documentation for specific implementation details.
What happens if I exceed the rate limits of the API?
Exceeding rate limits can lead to temporary or permanent IP bans from the API provider. Always monitor your usage and ensure you stay within the allowed limits.
By following these guidelines, you’ll be well on your way to effectively using the Amazon Scraping API for e-commerce data extraction. Happy scraping!