· Charlotte Will · webscraping · 5 min read
How to Scrape a Website Using Python
Learn how to scrape websites using Python in this comprehensive guide! Discover essential tools, libraries like Beautiful Soup and Scrapy, step-by-step tutorials, legal considerations, and best practices for efficient web scraping. Perfect for beginners and intermediate developers looking to master data extraction with Python.
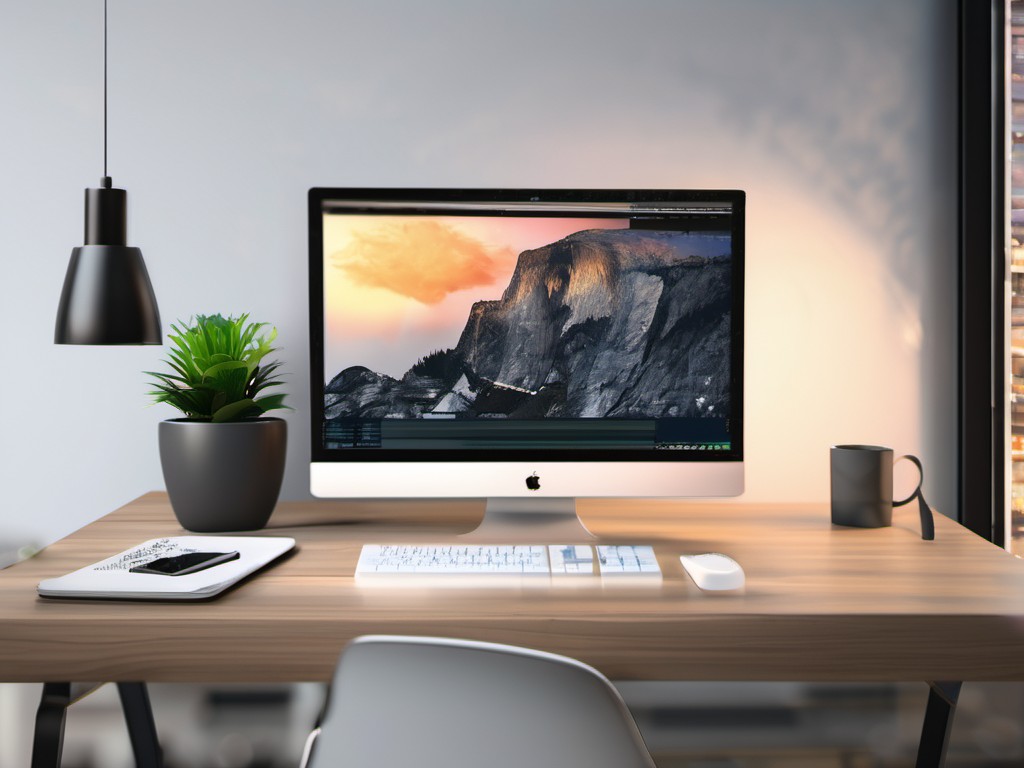
Are you looking to extract data from websites efficiently? Web scraping with Python is your answer! In this comprehensive guide, we’ll walk you through everything you need to know about web scraping using Python. From the basics to advanced techniques, legal considerations, and best practices—we’ve got it all covered.
Introduction to Web Scraping
Web scraping is the process of extracting data from websites programmatically. Whether you’re gathering data for research, building a dataset, or automating tasks, Python offers powerful tools that make web scraping straightforward and efficient.
What You Need to Know Before Starting
Before diving into coding, it’s important to understand the basics of web scraping:
- HTML/CSS: Basic knowledge of HTML (HyperText Markup Language) and CSS (Cascading Style Sheets) will help you identify data within a webpage.
- HTTP Requests: Understanding how HTTP requests work is essential for sending and receiving data from websites.
- Ethics and Legalities: Always respect the terms of service of the website you’re scraping, and ensure your actions are legal and ethical.
Tools and Libraries for Web Scraping in Python
Python has several libraries that simplify web scraping. Here’s a quick overview:
Beautiful Soup
Beautiful Soup is a library that makes it easy to parse HTML and XML documents. It’s perfect for beginners due to its simplicity and ease of use.
from bs4 import BeautifulSoup
import requests
# Send HTTP request to the website
url = 'https://example.com'
response = requests.get(url)
# Create a Beautiful Soup object
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify())
Requests Library
The requests
library is used to send HTTP requests in Python. It’s simple and powerful for making all kinds of requests.
import requests
url = 'https://example.com'
response = requests.get(url)
print(response.status_code) # Should be 200 if successful
Scrapy
Scrapy is an advanced web scraping framework that supports asynchronous operations, making it suitable for large-scale projects.
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
page = response.url.split("/")[-2]
filename = f'quotes-{page}.html'
with open(filename, 'wb') as f:
f.write(response.body)
Step-by-Step Guide to Web Scraping with Beautiful Soup
Let’s dive into a step-by-step guide on web scraping using Beautiful Soup and the requests library.
Setting Up Your Environment
First, install the required libraries:
pip install beautifulsoup4 requests
Sending an HTTP Request
Use the requests
library to fetch the HTML content of a webpage.
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.content
Parsing HTML with Beautiful Soup
Next, use Beautiful Soup to parse the HTML content and extract data.
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify()) # Print the parsed HTML in a readable format
Extracting Data from HTML Elements
Now you can navigate through the HTML elements and extract data using methods like find
, find_all
, and more.
# Find the first <h1> element
title = soup.find('h1').text
print(f'Title: {title}')
# Find all <p> elements
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
Advanced Web Scraping with Scrapy
For more complex projects, consider using Scrapy—an open-source web scraping framework.
Installing Scrapy
First, install Scrapy:
pip install scrapy
Creating a Scrapy Project
Start by creating a new Scrapy project:
scrapy startproject example_project
Navigate to the spiders
directory and create your first spider.
# example_project/spiders/example_spider.py
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
page = response.url.split("/")[-2]
filename = f'quotes-{page}.html'
with open(filename, 'wb') as f:
f.write(response.body)
Running Your Scrapy Spider
Execute your spider using the following command:
scrapy crawl example
Legal Considerations of Web Scraping
While web scraping is a powerful technique, it’s crucial to stay within legal boundaries. Always consider the following:
- Terms of Service: Check if the website’s terms of service allow for web scraping.
- Robots.txt File: Respect the
robots.txt
file which outlines what bots are allowed to do on a site. - Rate Limiting: Don’t overload servers with too many requests in a short period.
Best Practices for Web Scraping
To ensure your web scraping projects run smoothly, follow these best practices:
- Respect Robots.txt: Always check the
robots.txt
file to see which pages are allowed or disallowed for crawling. - Use Headers: Include headers in your requests to mimic a real user and avoid being blocked.
- Handle Exceptions: Implement error handling to manage issues like network errors, timeouts, etc.
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
headers = {'User-Agent': 'Mozilla/5.0'} # Add a User-Agent header
response = requests.get(url, headers=headers)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify())
else:
print('Failed to retrieve the page')
Conclusion
Web scraping with Python is a powerful way to extract data from websites efficiently. Whether you’re using Beautiful Soup for simple tasks or Scrapy for complex projects, always remember to follow legal guidelines and best practices. Happy scraping!
FAQs
What is web scraping?
Web scraping involves extracting data from websites programmatically. It’s used for various purposes such as research, dataset creation, and automation.
Is web scraping legal?
The legality of web scraping depends on the terms of service of the website you’re scraping and the laws in your jurisdiction. Always check the robots.txt
file and respect the site’s policies.
What are the best libraries for web scraping in Python?
Some of the best libraries for web scraping in Python include Beautiful Soup, Scrapy, and Requests. Each has its strengths depending on your needs.
How to handle dynamic content while scraping?
For dynamic content generated by JavaScript, you can use tools like Selenium or Playwright, which simulate a browser environment.
What are some best practices for web scraping?
Always respect the robots.txt
file, include headers in your requests to mimic real users, handle exceptions gracefully, and avoid overloading servers with too many requests at once.