· Charlotte Will · webscraping · 5 min read
How to Optimize Web Scraping Performance with Parallel Processing
Discover how to enhance your web scraping performance using parallel processing techniques such as multi-threading and asyncio. Learn practical strategies to boost speed, handle rate limits, and scale your web scrapers effectively.
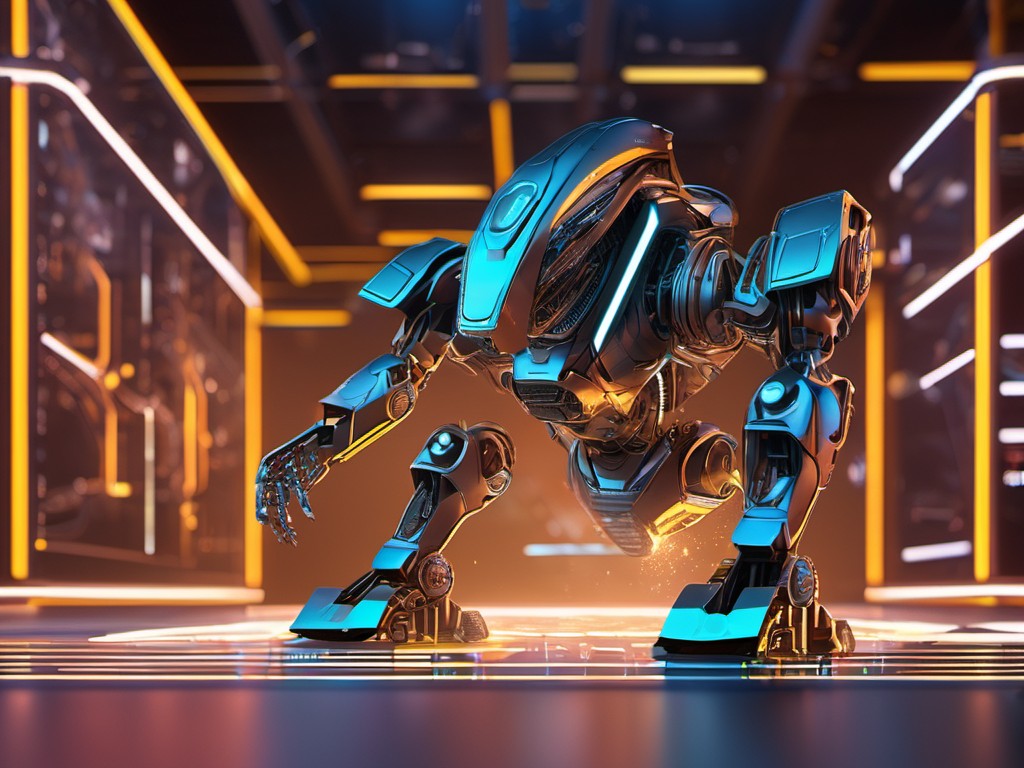
Web scraping has become an essential tool for data extraction and analysis, but it often faces performance bottlenecks. By leveraging parallel processing techniques such as multi-threading and asyncio, you can significantly enhance your web scraper’s efficiency and speed. This comprehensive guide will walk you through the best practices and strategies to optimize web scraping performance with parallel processing.
Understanding Web Scraping Performance
Web scraping involves extracting data from websites programmatically. However, the performance of a web scraper can be affected by various factors such as network latency, server response times, and rate limits imposed by websites. To optimize web scraping performance, it’s crucial to address these bottlenecks effectively.
Common Challenges in Web Scraping
- Network Latency: Delays in data transmission between the client and server can slow down the scraping process.
- Server Response Times: Websites with slower response times will naturally take longer to scrape.
- Rate Limits: Many websites impose rate limits to prevent overloading their servers, which can hinder the speed of your web scraper.
Introduction to Parallel Processing
Parallel processing involves running multiple tasks concurrently to improve efficiency and reduce completion time. In the context of web scraping, parallel processing can be achieved through multi-threading or asynchronous programming with libraries like asyncio and aiohttp.
Benefits of Parallel Processing in Web Scraping
- Improved Speed: By executing multiple requests simultaneously, you can drastically reduce the time taken to scrape data.
- Efficient Resource Utilization: Parallel processing allows for better utilization of available resources, such as CPU and network bandwidth.
- Handling Rate Limits: Distributing requests across multiple threads or connections helps in managing rate limits more effectively.
Optimizing Web Scraper Performance with Multi-threading
Multi-threading is a technique that allows for concurrent execution of tasks within the same program. Here’s how you can use multi-threading to enhance your web scraping performance:
Setting Up Multi-threaded Web Scraping in Python
import threading
import requests
def fetch_data(url):
response = requests.get(url)
return response.text
urls = ['http://example.com', 'http://example2.com']
threads = []
results = {}
for url in urls:
thread = threading.Thread(target=lambda: results.update({url: fetch_data(url)}))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(results)
Best Practices for Multi-threading
- Thread Pool: Use a thread pool to manage and reuse threads efficiently, avoiding the overhead of creating new threads repeatedly.
- Error Handling: Implement robust error handling to ensure that failures in one thread do not affect others.
- Synchronization: Use locks or semaphores to synchronize access to shared resources and prevent data corruption.
Enhancing Web Scraping with AsyncIO and Aiohttp
AsyncIO is a Python library for writing single-threaded concurrent code using coroutines, multiplexing I/O access over sockets and other resources, running network clients and servers, and other related primitives. When combined with aiohttp for making HTTP requests, it can significantly boost your web scraper’s performance.
Setting Up AsyncIO and Aiohttp
import asyncio
import aiohttp
async def fetch_data(session, url):
async with session.get(url) as response:
return await response.text()
urls = ['http://example.com', 'http://example2.com']
async def main():
async with aiohttp.ClientSession() as session:
tasks = [fetch_data(session, url) for url in urls]
results = await asyncio.gather(*tasks)
return dict(zip(urls, results))
results = asyncio.run(main())
print(results)
Best Practices for AsyncIO and Aiohttp
- Semaphore for Rate Limiting: Use a semaphore to limit the number of concurrent requests and handle rate limits more gracefully.
- Error Handling: Implement error handling within async functions to ensure that exceptions in one task do not stop others from completing.
- Session Management: Reuse HTTP sessions to improve performance and reduce overhead.
Scaling Web Scrapers
When dealing with large-scale web scraping projects, it’s essential to consider scalability. Distributing tasks across multiple machines or using cloud services can help scale your web scraper efficiently.
Distributed Web Scraping
- Task Queues: Use task queues like RabbitMQ or Celery to distribute scraping tasks among multiple workers.
- Cloud Services: Leverage cloud platforms such as AWS Lambda, Google Cloud Functions, or Azure Functions for scalable and serverless web scraping.
- Load Balancing: Implement load balancers to evenly distribute network requests across multiple servers.
Efficient Web Scraping with Python
Python offers a rich ecosystem of libraries and tools that can enhance your web scraping performance. Here are some additional techniques and libraries to consider:
- BeautifulSoup: For parsing HTML and XML documents efficiently.
- Scrapy: An open-source and collaborative web crawling framework for Python.
- Lxml: A library for processing XML and HTML, known for its performance and efficiency.
Handling Rate Limits in Web Scraping
Rate limits are a common challenge in web scraping. Here’s how you can handle them effectively:
Strategies to Manage Rate Limits
- Exponential Backoff: Increase the delay between requests exponentially after receiving a rate limit error.
- Rotating Proxies: Use rotating proxies to distribute your requests across multiple IP addresses.
- User-Agent Rotation: Rotate user agents to mimic different browsers and avoid detection.
Conclusion
Optimizing web scraping performance with parallel processing can significantly improve the efficiency and speed of your data extraction projects. By leveraging multi-threading, asyncio, and aiohttp, you can address common bottlenecks and handle rate limits more effectively. Additionally, scaling your web scrapers and using Python’s rich ecosystem of libraries can further enhance your performance.
FAQ
1. What is the best way to handle rate limits in web scraping?
Handling rate limits involves strategies like exponential backoff, rotating proxies, and user-agent rotation. Implementing a combination of these techniques can help manage rate limits more effectively.
2. How does multi-threading improve web scraping performance?
Multi-threading allows for concurrent execution of tasks within the same program. This means multiple HTTP requests can be sent simultaneously, reducing the overall time taken to scrape data.
3. What is asyncio, and how can it enhance web scraping?
AsyncIO is a Python library for writing single-threaded concurrent code using coroutines. When combined with aiohttp for making HTTP requests, it enables non-blocking I/O operations, significantly improving the performance of your web scraper.
4. How can I scale my web scrapers?
Scaling web scrapers involves distributing tasks across multiple machines or using cloud services. Techniques like task queues, cloud platforms, and load balancers can help achieve scalability efficiently.
5. What are some best practices for error handling in parallel processing?
Best practices for error handling include implementing robust error handling within each thread or coroutine, using locks or semaphores to synchronize access to shared resources, and ensuring that failures in one task do not affect others.