· Charlotte Will · webscraping · 5 min read
How to Optimize Web Scraper Performance with Multi-Threading
Discover how to optimize web scraper performance with multi-threading techniques, improving speed and efficiency. Learn practical tips and advanced methods for concurrent processing in Python, ensuring faster data extraction without compromising ethical considerations.
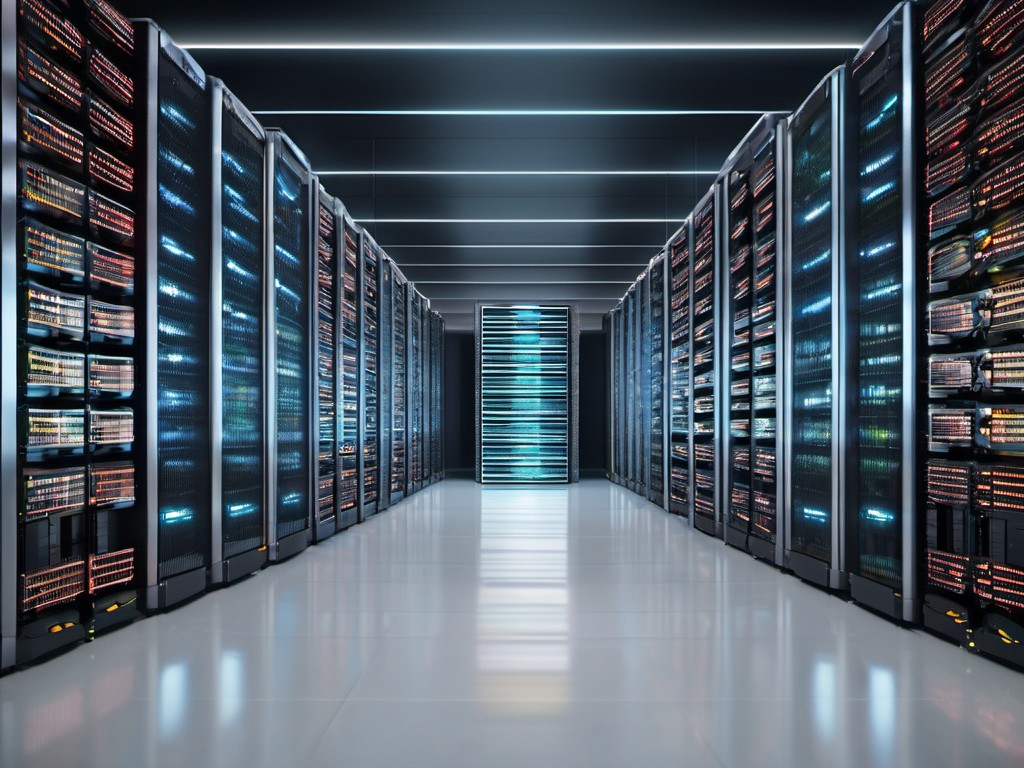
Web scraping is an essential tool for data extraction, but its performance can often leave much to be desired. Whether you’re scraping large datasets or dealing with complex web structures, slow speeds and inefficiencies can seriously hamper your workflow. That’s where multi-threading comes into play. By leveraging the power of concurrent processing, you can significantly boost your web scraper’s performance. In this article, we’ll delve into the intricacies of optimizing web scraper performance with multi-threading, providing practical tips and techniques to enhance your data extraction capabilities.
Understanding Multi-Threading in Web Scraping
Multi-threading is a technique that allows multiple threads within a single process to run concurrently. In the context of web scraping, this means you can send multiple requests simultaneously rather than waiting for one request to complete before sending another. This parallelization can drastically reduce the overall time taken to scrape data from websites.
Why Multi-Threading Matters
- Speed: By making multiple requests concurrently, you reduce the total time spent on data extraction.
- Efficiency: Better utilization of system resources leads to more efficient processing.
- Scalability: Multi-threading allows your web scraper to handle larger datasets and more complex tasks without significant performance degradation.
Getting Started with Multi-Threading
Before diving into the specifics, let’s ensure you have a basic understanding of how multi-threading works in Python, one of the most popular languages for web scraping.
Basic Setup with ThreadPoolExecutor
Python’s concurrent.futures
module provides a high-level interface for asynchronously executing callables using threads or processes. Here’s a basic example:
from concurrent.futures import ThreadPoolExecutor
import requests
def fetch_data(url):
response = requests.get(url)
return response.text
urls = [
'https://example.com/page1',
'https://example.com/page2',
# Add more URLs as needed
]
with ThreadPoolExecutor() as executor:
results = list(executor.map(fetch_data, urls))
In this example, ThreadPoolExecutor
manages a pool of threads and distributes the tasks among them. This allows your web scraper to fetch data from multiple URLs concurrently.
Advanced Techniques for Multi-Threading
Customizing Thread Pool Size
The number of threads in your thread pool can significantly impact performance. Too few threads may not fully utilize system resources, while too many can lead to overhead and decreased efficiency due to context switching. Experimenting with different numbers of threads is crucial for finding the optimal configuration:
with ThreadPoolExecutor(max_workers=10) as executor:
results = list(executor.map(fetch_data, urls))
Handling Rate Limiting
When scraping websites that enforce rate limits, it’s essential to respect these limits to avoid getting banned. You can manage this by introducing delays between requests:
import time
def fetch_data(url):
time.sleep(1) # Simulate delay to respect rate limiting
response = requests.get(url)
return response.text
Error Handling and Retries
Real-world web scraping often involves dealing with unreliable networks and servers. Implementing robust error handling and retries is key:
import requests
from concurrent.futures import ThreadPoolExecutor, as_completed
def fetch_data(url):
attempts = 0
while attempts < 3:
try:
response = requests.get(url)
return response.text
except requests.RequestException:
attempts += 1
time.sleep(2 ** attempts) # Exponential backoff
return None
with ThreadPoolExecutor() as executor:
future_to_url = {executor.submit(fetch_data, url): url for url in urls}
for future in as_completed(future_to_url):
url = future_to_url[future]
try:
data = future.result()
if data is not None:
print(f"Data from {url}: {data}")
except Exception as exc:
print(f'{url} generated an exception: {exc}')
Optimizing Your Web Scraper
Efficient Data Processing
Multi-threading is not just about sending requests; it’s also about processing the data efficiently. Use libraries like pandas
to handle large datasets effectively:
import pandas as pd
def process_data(data):
# Your data processing logic here
return data
with ThreadPoolExecutor() as executor:
processed_data = list(executor.map(process_data, results))
df = pd.DataFrame(processed_data)
Resource Management
Multi-threading can be resource-intensive. Monitor your system’s CPU and memory usage to ensure you’re not overloading it:
import psutil
def check_resources():
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
return cpu_usage, memory_info
with ThreadPoolExecutor() as executor:
results = list(executor.map(fetch_data, urls))
cpu_usage, memory_info = check_resources()
print(f"CPU Usage: {cpu_usage}%")
print(f"Memory Info: {memory_info}")
Best Practices for Multi-Threaded Web Scraping
Respect Robots.txt
Always respect the robots.txt
file of websites you are scraping to ensure compliance with their policies and avoid legal issues.
Use Proxies
Using proxies can help distribute your requests across different IP addresses, reducing the likelihood of being blocked.
Monitor and Log
Implement comprehensive logging and monitoring to track your web scraper’s performance and identify bottlenecks or errors quickly.
Conclusion
Optimizing web scraper performance with multi-threading is a powerful way to enhance data extraction efficiency. By leveraging concurrent processing, you can significantly reduce the time taken for tasks, handle larger datasets, and manage complex structures more effectively. However, it’s essential to balance speed with resource management and ethical considerations.
FAQ
1. What is the optimal number of threads for web scraping?
The optimal number of threads depends on your system’s capabilities and the specific requirements of your task. Experimentation is key, but generally, starting with a thread pool size similar to the number of CPU cores is a good strategy.
2. How can I handle rate limits while using multi-threading?
Introduce delays between requests to respect rate limits. You can use exponential backoff strategies to retry failed requests, gradually increasing the delay between attempts.
3. What libraries are best for multi-threaded web scraping in Python?
concurrent.futures
, requests
, and pandas
are excellent choices for multi-threaded web scraping in Python. These libraries offer robust features for concurrent processing, making HTTP requests, and handling large datasets.
4. How can I avoid being banned while scraping?
Respect the website’s robots.txt
file, use proxies to distribute requests across different IP addresses, and implement rate limiting to avoid overwhelming the server. Also, consider adding delays between requests and handling retries gracefully.
5. What are some common pitfalls in multi-threaded web scraping?
Common pitfalls include overloading system resources, ignoring error handling and retries, not respecting rate limits, and failing to monitor and log performance metrics effectively. Always balance speed with resource management and ethical considerations.