· Charlotte Will · 4 min read
How to Make an Amazon Scrape: A Step-by-Step Guide
Learn how to scrape data from Amazon using Python, Selenium, BeautifulSoup, and other tools. Follow our step-by-step guide to extract product information ethically and effectively. Boost your business insights with competitive intelligence and real-time data.
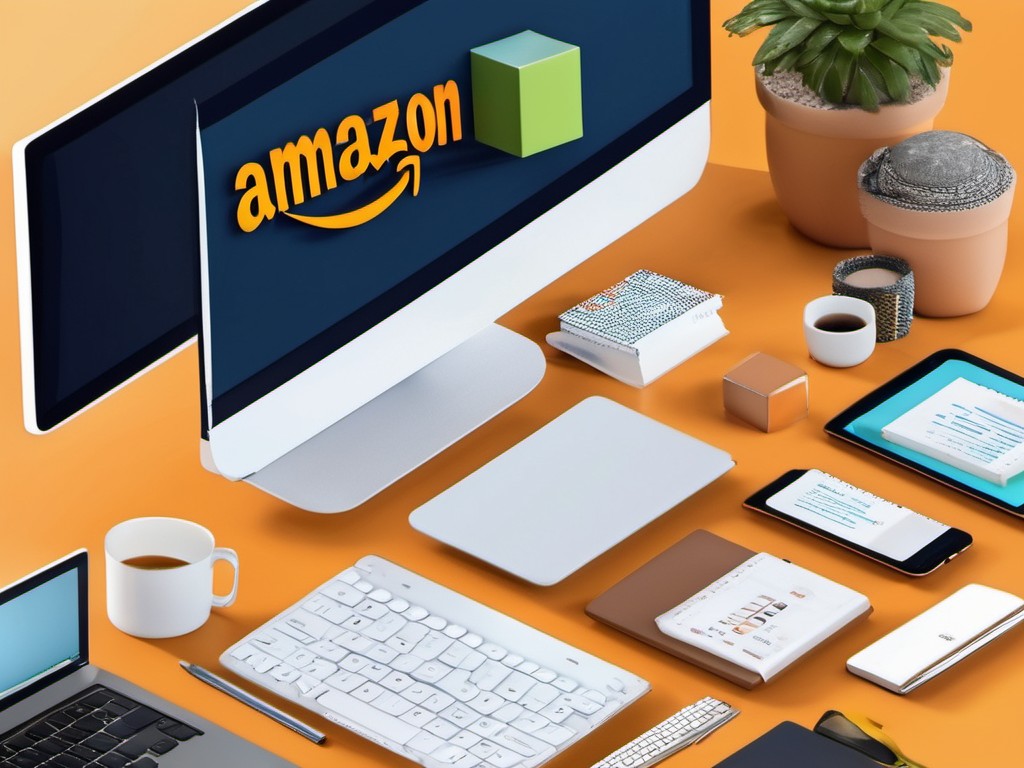
Understanding Web Scraping and Its Benefits
What is Web Scraping?
Web scraping, also known as web harvesting or data extraction, involves using software tools or scripts to extract information from websites. It’s like a digital treasure hunt where you collect valuable data hidden within the vast expanse of the internet. Amazon, with its wealth of product information, is one such treasure trove.
Why Scrape Amazon?
Amazon is an e-commerce behemoth that hosts millions of products and their corresponding data—prices, reviews, descriptions, and more. For businesses, scraping Amazon can provide competitive intelligence, help in price optimization, monitor product trends, and even gather consumer sentiment.
Tools You’ll Need for Amazon Scraping
Before diving into the technicalities, let’s discuss some essential tools you’ll need:
- Programming Language: Python is highly recommended due to its simplicity and powerful libraries such as BeautifulSoup and Scrapy.
- Web Browser: Tools like Selenium can automate browser actions, making it easier to scrape dynamic content.
- IP Rotation Service: Services like Luminati or ScraperAPI help in rotating IP addresses to prevent getting blocked by Amazon.
- Proxy Server: A proxy server can mask your real IP address and distribute your requests across multiple IPs.
Step-by-Step Guide to Scraping Amazon
Setting Up Your Environment
- Install Python: Ensure you have the latest version of Python installed. You can download it from python.org.
- Set Up a Virtual Environment: This isolates your project dependencies from your global site-packages, preventing conflicts.
python -m venv amazon_scraper source amazon_scraper/bin/activate # On Windows use `amazon_scraper\Scripts\activate`
- Install Required Libraries: Use pip to install essential libraries like BeautifulSoup, Requests, and Selenium.
pip install beautifulsoup4 requests selenium
Writing the Script
Import Libraries: Start by importing the necessary libraries in your script.
from bs4 import BeautifulSoup import requests from selenium import webdriver from selenium.webdriver.common.keys import Keys
Initialize Selenium WebDriver: Set up the Selenium WebDriver to interact with Amazon’s website.
driver = webdriver.Chrome() # Ensure you have the ChromeDriver installed
Search for Products: Automate a search query on Amazon using Selenium.
driver.get('https://www.amazon.com') search_box = driver.find_element_by_id('twotabsearchtextbox') search_box.send_keys('laptop') search_box.send_keys(Keys.RETURN)
Extract Data: Once the results page loads, use BeautifulSoup to parse and extract relevant data.
soup = BeautifulSoup(driver.page_source, 'html.parser') items = soup.find_all('div', {'data-component-type': 's-search-result'}) for item in items: title = item.find('span', {'class': 'a-size-medium a-color-base a-text-normal'}).get_text(strip=True) price = item.find('span', {'class': 'a-price-whole'}).get_text(strip=True) print(f"Title: {title}, Price: ${price}")
Running and Testing Your Scraper
- Run the Script: Execute your script to see if it works as expected. Make sure you handle exceptions and errors gracefully.
- Test with Different Queries: Try searching for different products to ensure your scraper is versatile.
- Rotate IP Addresses: Use an IP rotation service or proxy server to avoid getting blocked by Amazon.
proxies = { 'http': 'your_proxy_here', 'https': 'your_proxy_here' } response = requests.get('https://www.amazon.com/s?k=laptop', proxies=proxies)
- Check for Updates: Amazon frequently changes its website structure, so ensure your scraper is up to date by periodically checking and modifying your code as needed.
Best Practices for Ethical Web Scraping
- Respect Robots.txt: Always check the
robots.txt
file of a website to understand what’s allowed and what’s not. - Rate Limiting: Implement rate limiting to avoid overwhelming the server with too many requests at once.
- Use Headers: Mimic a real browser by including headers in your HTTP requests.
- Handle Exceptions: Gracefully handle exceptions such as network errors or changes in the website layout.
- Store Data Responsibly: Ensure that any data you scrape is stored securely and used ethically.
FAQ Section
Is web scraping legal?
Web scraping can be legally complex, depending on your jurisdiction and the terms of service of the website you’re scraping. Always ensure you’re complying with local laws and respecting the website’s robots.txt file.
What tools are best for Amazon scraping?
For Amazon scraping, a combination of Python (with libraries like BeautifulSoup and Scrapy), Selenium for automation, and an IP rotation service or proxy server works best.
How do I avoid getting blocked by Amazon?
To avoid getting blocked, use an IP rotation service, set up rate limiting in your requests, mimic real browser behavior with headers, and respect the website’s robots.txt file.
Can I scrape Amazon without using Selenium?
Yes, you can scrape Amazon without Selenium by using libraries like BeautifulSoup or Scrapy. However, Selenium is particularly useful for handling dynamic content that relies on JavaScript to load.
Is it safe to share the scripts I create for web scraping?
Sharing your scraping scripts can be risky as others might use them irresponsibly, leading to IP blacklisting or legal issues. If you must share, ensure you anonymize any sensitive information and clearly outline ethical usage guidelines.
By following this comprehensive guide, you should now have a solid foundation for scraping Amazon effectively and ethically. Happy scraping!