· Charlotte Will · Amazon API · 6 min read
How to Integrate Amazon MWS API for Order Management
Learn how to integrate Amazon MWS API for efficient order management in this comprehensive guide. Discover step-by-step instructions, best practices, and practical examples to streamline your e-commerce operations and enhance automation.
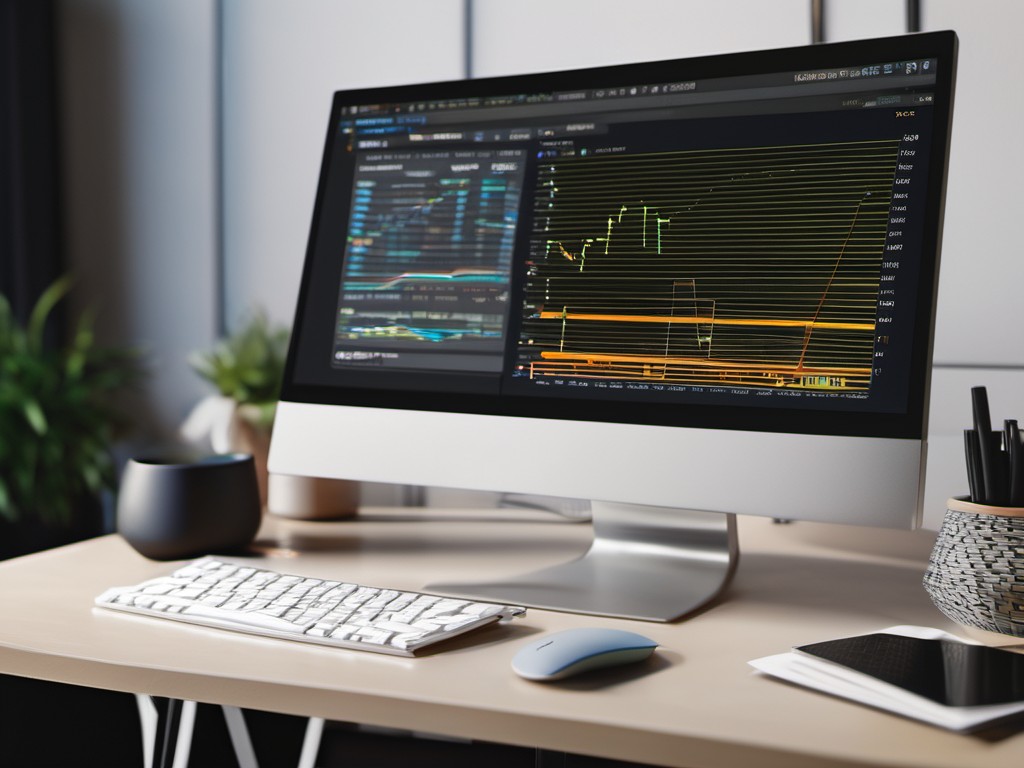
Are you an e-commerce business owner looking to streamline your order management process? Integrating Amazon Marketplace Web Service (MWS) API can revolutionize the way you manage orders, automating processes and saving valuable time. In this comprehensive guide, we’ll walk you through integrating Amazon MWS API for efficient order management, covering everything from API setup to automated order processing.
Understanding Amazon MWS API
Before diving into the integration process, let’s understand what Amazon MWS API is and how it can benefit your e-commerce business.
What is Amazon MWS?
Amazon Marketplace Web Service (MWS) is a set of web services that enables you to programmatically access Amazon seller account information. This includes order details, inventory levels, and more. By using the MWS API, you can automate various aspects of your e-commerce operations, making your business more efficient.
Benefits of Using Amazon MWS API
Integrating Amazon MWS API offers several advantages:
- Automated Order Processing: Streamline your order management system by automatically retrieving and processing orders.
- Inventory Management: Keep track of stock levels in real time to avoid overselling or running out of inventory.
- Customer Service Improvement: Provide faster and more accurate order updates, enhancing customer satisfaction.
Getting Started with Amazon MWS API Integration
Now that you understand the benefits, let’s get started with integrating Amazon MWS API into your e-commerce platform.
Step 1: Create an AWS Account
To use Amazon MWS, you need to have an Amazon Web Services (AWS) account. If you don’t already have one, go to the AWS website and sign up for a free account.
Step 2: Register as an Amazon Developer
Next, register as an Amazon developer by visiting the Amazon MWS Developer Portal. This step is crucial as it provides you with access to the necessary API keys and credentials.
Step 3: Obtain Your MWS Credentials
Once registered, you’ll need to obtain your MWS credentials, including the Seller ID, Marketplace ID, Access Key, and Secret Key. These will be essential for authenticating your API requests.
Setting Up the Amazon MWS API Environment
With your credentials in hand, let’s set up the environment for integrating Amazon MWS API.
Step 4: Choose Your Programming Language
Amazon MWS supports multiple programming languages such as Java, C#, Python, and PHP. Select the language that best suits your development team’s expertise.
Step 5: Install Required Libraries and SDKs
Depending on your chosen programming language, you may need to install specific libraries or SDKs. For example, if you’re using Python, you can use the boto3
library for AWS services.
Integrating Amazon MWS API for Order Management
Now that your environment is set up, let’s dive into the integration process for managing orders with Amazon MWS API.
Step 6: Authenticate Your API Requests
To securely communicate with Amazon MWS, you need to authenticate your requests using your credentials. This involves signing each request with your Access Key and Secret Key.
Example Code Snippet (Python)
import hmac
import hashlib
import base64
from time import gmtime, strftime
def sign_request(params):
# Your Access Key and Secret Key here
access_key = 'your-access-key'
secret_key = 'your-secret-key'
string_to_sign = "GET\n" + params['endpoint'] + "\n" + params['uri'] + "\n" + params['query_string']
signature = hmac.new(secret_key, string_to_sign.encode('utf-8'), hashlib.sha256).digest()
return base64.b64encode(signature).decode('utf-8')
Step 7: Retrieve Orders Using MWS API
To manage orders, you need to retrieve order information from Amazon using the ListOrders
operation of the MWS Orders API. This will provide you with details such as order IDs, customer information, and product details.
Example Code Snippet (Python)
import requests
def list_orders(access_key, secret_key, seller_id):
# Construct the request parameters
params = {
'AWSAccessKeyId': access_key,
'Action': 'ListOrders',
'SellerId': seller_id,
'SignatureMethod': 'HmacSHA256',
'SignatureVersion': '2',
'Timestamp': strftime('%Y-%m-%dT%H:%M:%SZ', gmtime()),
'Version': '2013-09-01'
}
# Sign the request
params['Signature'] = sign_request(params)
response = requests.get('https://mws.amazonservices.com/Orders/2013-09-01', params=params)
return response.text
Step 8: Automate Order Processing
With order information retrieved, you can automate various aspects of your order management system. This includes updating inventory levels, generating invoices, and notifying customers about their order status.
Example Use Cases:
- Inventory Management: Update stock levels in real time to reflect recent orders and avoid overselling.
- Order Fulfillment: Automatically generate shipping labels and notify carriers for faster delivery.
- Customer Communication: Send automated emails or SMS notifications to customers regarding their order status.
Best Practices for Amazon MWS API Integration
To ensure a smooth integration process, follow these best practices:
Error Handling and Logging
Implement robust error handling mechanisms to catch any issues during API requests. Log all errors for easier troubleshooting and monitoring.
Example Code Snippet (Python)
import logging
logging.basicConfig(filename='mws_api.log', level=logging.ERROR)
def handle_error(response):
if response.status_code != 200:
logging.error('Error occurred: {}'.format(response.text))
Rate Limiting and Throttling
Amazon MWS has rate limits for API requests to prevent abuse and ensure fair usage. Make sure your integration respects these limits to avoid being throttled or banned.
Example:
Implement a delay between consecutive API calls to stay within the allowed request quota.
import time
def list_orders(access_key, secret_key, seller_id):
# Existing code...
response = requests.get('https://mws.amazonservices.com/Orders/2013-09-01', params=params)
if response.status_code == 429: # Rate limit exceeded
time.sleep(60) # Wait for a minute before retrying
return response.text
Security Measures
Ensure that your API keys and credentials are stored securely to prevent unauthorized access. Use environment variables or secure vaults instead of hardcoding sensitive information in your code.
Conclusion
Integrating Amazon MWS API for order management can significantly enhance the efficiency of your e-commerce operations. By automating processes such as order retrieval, inventory updates, and customer notifications, you can save time and improve overall business productivity. Following best practices like robust error handling, rate limiting, and secure credential storage will ensure a smooth integration process.
FAQs
1. What is Amazon MWS API used for?
Amazon MWS API is used to programmatically access seller account information on Amazon, enabling automation of various e-commerce operations such as order management, inventory tracking, and customer communication.
2. How do I get started with Amazon MWS API integration?
To get started, you need to create an AWS account, register as an Amazon developer, obtain your MWS credentials, choose a programming language, and install the required libraries or SDKs.
3. What are some benefits of using Amazon MWS API for order management?
Benefits include automated order processing, real-time inventory management, improved customer service through faster order updates, and overall enhanced efficiency in e-commerce operations.
4. How do I handle errors during API requests with Amazon MWS?
Implement robust error handling mechanisms to catch issues during API requests and log all errors for easier troubleshooting. Use libraries like logging
in Python to record error details.
5. What are the rate limits for Amazon MWS API requests?
Amazon MWS has specific rate limits that vary depending on the type of request and the seller’s account status. Ensure your integration respects these limits to avoid being throttled or banned.