· Charlotte Will · webscraping · 6 min read
How to Implement Retry Logic for Reliable Web Scraping
Discover how to enhance your web scraping projects by implementing retry logic. This comprehensive guide covers practical techniques, best practices, and common pitfalls, ensuring your data extraction remains reliable and efficient. Perfect for beginners and intermediate users looking to improve their web scraping skills.
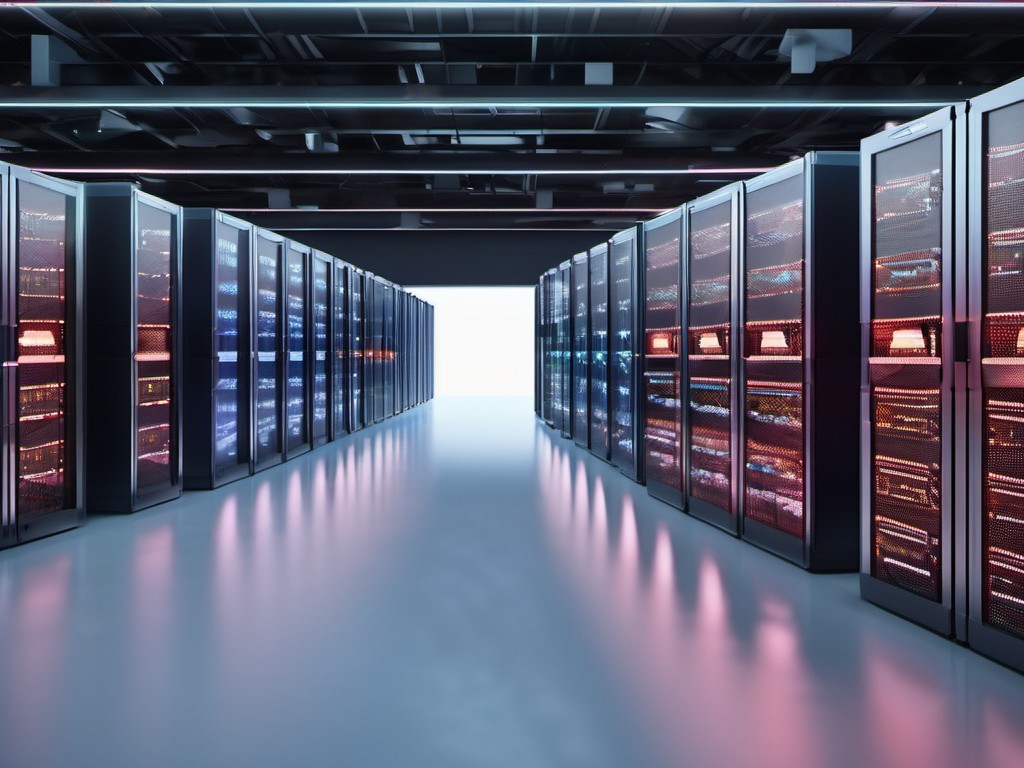
Web scraping can be an invaluable tool for extracting data from websites, but it’s not without its challenges. One of the most common issues you’ll encounter is the occasional failure of requests due to network instability, server overloads, or rate limiting. To ensure your web scraping project remains reliable and efficient, implementing retry logic is essential. In this comprehensive guide, we’ll walk you through the process of adding retry logic to your web scraper, providing practical advice and actionable tips along the way.
Understanding Retry Logic
Retry logic is a technique used in programming to handle transient errors that may occur during network requests. By implementing retry logic, you can reattempt failed requests after a specified delay or under certain conditions, thereby increasing the chances of successful data extraction.
Why Use Retry Logic?
- Network Instability: Temporary network issues can cause requests to fail. Retrying these requests can often resolve the problem.
- Server Overloads: Sometimes servers are overwhelmed with too many requests at once, leading to temporary failures. Retrying helps mitigate this issue.
- Rate Limiting: Many websites impose rate limits on requests to prevent abuse. Retry logic can help you stay within these limits and avoid getting blocked.
- Improved Reliability: By implementing retry logic, your web scraper becomes more resilient and less prone to failure.
Basic Principles of Retry Logic
Before diving into the implementation details, let’s outline some basic principles for effective retry logic:
- Exponential Backoff: Increase the delay between retries exponentially. This helps in avoiding overwhelming the server with rapid retries.
- Jitter: Introduce randomness to the delay between retries to avoid synchronization issues when multiple clients are making requests.
- Maximum Retries: Set a limit on the number of retries to prevent endless loops and resource wastage.
- Retry Conditions: Only retry certain types of errors, such as network-related or rate limiting errors. Avoid retrying permanent errors like 404 Not Found.
- Backoff Factor: Choose a backoff factor that balances between quick retries and preventing server overload.
Implementing Retry Logic in Python
We’ll use Python for this guide, given its popularity in the web scraping community. We’ll also utilize the requests
library, which is widely used for making HTTP requests.
Step 1: Install Required Libraries
First, ensure you have the requests
library installed. You can install it using pip:
pip install requests
Step 2: Define a Retry Function
Create a function that handles retries based on the principles outlined above. Here’s an example implementation:
import time
import random
from requests.exceptions import RequestException
def make_request(url, max_retries=5):
attempt = 0
backoff = 0.1 # Starting backoff factor
while attempt < max_retries:
try:
response = requests.get(url)
response.raise_for_status() # Raise an error for bad status codes
return response
except RequestException as e:
if 'timeout' in str(e):
print("Request timed out, retrying...")
elif 'too many requests' in str(e):
print("Rate limit exceeded, retrying with exponential backoff...")
else:
# Log the error and break if it's not a transient error
print(f"Request failed with error: {e}")
break
attempt += 1
delay = backoff * (2 ** attempt) + random.uniform(0, 1)
print(f"Retrying in {delay:.2f} seconds...")
time.sleep(delay)
backoff = min(backoff * 2, 64) # Cap the backoff factor at 64 seconds
return None
Step 3: Integrate Retry Logic into Your Web Scraper
Here’s an example of how to integrate the retry logic function into a simple web scraping script:
import requests
from bs4 import BeautifulSoup
def scrape_website(url):
response = make_request(url)
if not response:
print("Failed to retrieve data after multiple attempts.")
return
soup = BeautifulSoup(response.content, 'html.parser')
# Extract the required data from the page
title = soup.title.string
print(f"Scraped title: {title}")
if __name__ == "__main__":
url = "https://example.com"
scrape_website(url)
Advanced Retry Logic Techniques
For more advanced scenarios, consider the following techniques to enhance your retry logic:
Adaptive Backoff
Adaptive backoff algorithms adjust the delay between retries based on the specific error type and server behavior. This can be particularly useful for handling rate-limited APIs or unstable networks.
Circuit Breaker Pattern
The circuit breaker pattern helps prevent your application from repeatedly attempting to execute a failing operation, thus saving resources. When the number of consecutive failures reaches a threshold, the circuit opens, and requests are no longer made. After a cool-down period, the circuit closes, allowing retries to resume.
Retrying with Different Strategies
Depending on the error type, you can implement different retry strategies. For instance:
- Network errors: Use exponential backoff with jitter.
- Rate limiting errors: Implement a more aggressive backoff and consider rotating proxies.
- Server errors: Retry immediately but limit the number of retries to avoid infinite loops.
Common Pitfalls and Best Practices
Pitfalls
- Infinite Loops: Ensure you have a maximum retry limit to prevent endless loops.
- Ignoring Error Codes: Not all errors are transient. Ignore permanent errors like 404 or 500.
- Server Overload: Be cautious with aggressive retries, as they can overwhelm the server and get your IP blocked.
Best Practices
- Log Errors: Keep a log of retry attempts and failed requests for debugging and analysis.
- Monitor Retry Rates: Regularly monitor the number of retries to ensure your application isn’t making too many requests.
- Rate Limiting Compliance: Ensure your retry logic adheres to the rate limits set by the target website.
- Use Proxies Wisely: Rotating proxies can help distribute the load and avoid IP blocking, but use them responsibly.
Conclusion
Implementing retry logic is crucial for creating reliable web scrapers that can handle transient errors gracefully. By following the principles outlined in this guide and utilizing the provided examples, you’ll be well on your way to building a robust web scraper capable of extracting data efficiently even in the face of network instability or server overloads.
FAQs
1. What is retry logic, and why is it important for web scraping?
Retry logic is a technique used to handle transient errors during HTTP requests by attempting them again after a specified delay. It’s crucial for web scraping because network issues, server overloads, and rate limiting can cause requests to fail temporarily. Retry logic ensures that these failed requests are retried, increasing the chances of successful data extraction.
2. How does exponential backoff work in retry logic?
Exponential backoff increases the delay between retries exponentially with each attempt. This helps prevent overwhelming the server with rapid retries and provides a balanced approach to handling transient errors without causing excessive load on the target website.
3. What is the circuit breaker pattern, and how does it help in retry logic?
The circuit breaker pattern prevents an application from repeatedly attempting to execute a failing operation by opening a “circuit” after a specified number of consecutive failures. This saves resources and prevents further retries until the circuit closes after a cool-down period. It’s particularly useful for handling persistent errors that are unlikely to resolve with additional retries.
4. How can I handle rate limiting in web scraping using retry logic?
To handle rate limiting, implement more aggressive backoff strategies and consider rotating proxies. Additionally, ensure your retry logic adheres to the rate limits set by the target website to avoid getting your IP blocked. Monitoring the number of retries can also help you stay within these limits.
5. What are some common pitfalls to avoid when implementing retry logic in web scrapers?
Some common pitfalls include:
- Creating infinite loops without a maximum retry limit.
- Ignoring error codes and retrying permanent errors like 404 Not Found.
- Using overly aggressive retries that can overwhelm the server and lead to IP blocking.
By being mindful of these pitfalls and following best practices, you can implement effective retry logic for reliable web scraping.