· Charlotte Will · 16 min read
How to Implement Custom Business Logic with Amazon Data API and AWS Lambda
Learn how to implement custom business logic using Amazon Data API and AWS Lambda for efficient data processing and automation. Discover best practices, real-world examples, and step-by-step guides to enhance your applications.
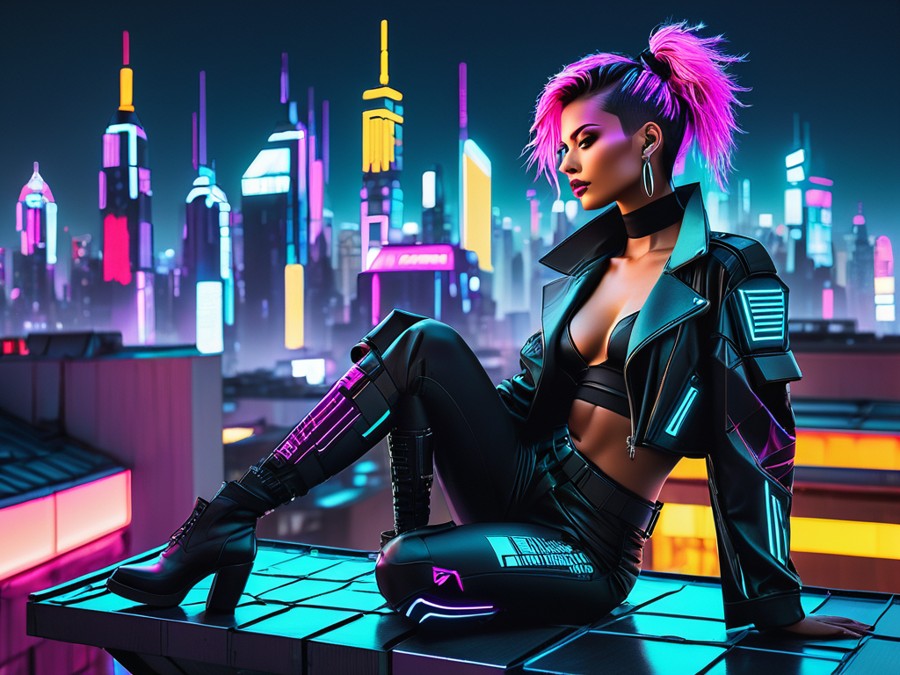
Introduction
Navigating the complexities of modern business operations often requires seamless integration of custom rules and data processing. This is where Amazon Data API and AWS Lambda come into play, offering a powerful combination to streamline your business logic. Whether you’re automating workflows, processing data in real-time, or enhancing user experiences, these tools can significantly elevate your operations.
In this article, we’ll guide you through the process of implementing custom business logic using Amazon Data API and AWS Lambda. We’ll cover everything from setting up your environment to writing efficient Lambda functions, integrating with Amazon Data API, and monitoring performance. You’ll also learn best practices for security, scalability, and optimization. By the end, you’ll have a clear roadmap to leverage these AWS services for your custom business needs. Let’s dive in and unlock the potential of serverless computing with AWS Lambda and Amazon Data API.
Understanding Custom Business Logic
What is Custom Business Logic?
Custom business logic refers to the unique set of rules and processes that govern your specific business operations. This can include anything from complex data transformations to specialized decision-making algorithms tailored to your company’s needs.
For example, a retail business might need to apply dynamic pricing based on inventory levels, time of day, and other factors. Another example could be a finance company that needs to automatically trigger alerts based on transaction patterns indicative of fraud.
Implementing custom business logic effectively can significantly improve operational efficiency, reduce human error, and enhance decision-making processes. To do this with Amazon Data API and AWS Lambda, we need to understand how these tools can help us execute our custom rules seamlessly.
Examples of Custom Business Rules
Let’s consider a few practical examples:
Inventory Management: Suppose you want to automatically update your inventory levels and trigger restocking processes when stock falls below a certain threshold. AWS Lambda can execute this business rule in response to data changes from Amazon Data API.
Real-Time Analytics: If you’re looking to perform real-time analytics on incoming data, AWS Lambda can process this data and invoke custom business rules instantly. For instance, you could use Amazon Kinesis to stream real-time data and AWS Lambda to process it on the fly (see our article How to Implement Real-Time Analytics with Amazon Kinesis and API Data).
User Authentication: Custom business logic can also handle user authentication processes, ensuring that only authorized users access your data. You could use Amazon Cognito for this purpose along with AWS Lambda (see How to Implement User Authentication in Your App with Amazon Cognito and API Data).
Integration with AWS Lambda
AWS Lambda is a serverless computing service that lets you run code without provisioning or managing servers. By leveraging AWS Lambda, you can execute custom business logic in response to events like changes in data stored in Amazon Data API.
The key advantage here is that AWS Lambda scales automatically, handling each trigger individually and charging you based on actual usage. This means you can focus on writing the logic for your business rules without worrying about infrastructure management.
In the next section, we’ll dive deeper into how to set up and configure AWS Lambda for your use case.
Introduction to Amazon Data API
Key Features of Amazon Data API
Amazon Data API is a set of APIs designed to help you integrate and manage data within Amazon services. These APIs provide programmatic access to various AWS resources, enabling you to build robust and scalable applications that can interact with Amazon services seamlessly.
Key features of Amazon Data API include:
- Data Access and Manipulation: You can retrieve, create, update, and delete data stored in Amazon services.
- Secure Data Interactions: With built-in security features, you can ensure that your data interactions are secure and compliant with industry standards.
- Integration with AWS Services: Amazon Data API integrates seamlessly with other AWS services, making it easy to build complex workflows and automate data processing tasks.
Integration with Other AWS Services
Amazon Data API can be integrated with a wide range of AWS services, enabling you to create powerful and dynamic applications. For instance, you can use Amazon Data API with Amazon S3 for data storage, Amazon DynamoDB for fast and flexible database operations, and AWS Lambda for serverless computing.
By combining these services, you can create robust data pipelines that handle complex business logic efficiently. For example, you might use Amazon S3 to store raw data and AWS Lambda functions to process this data in real-time using custom business rules. This combination can help you achieve better performance and scalability.
Example Use Case
Imagine a scenario where you are building a custom data warehouse to enhance business insights. You can leverage Amazon Data API and AWS Lambda to automate the process of collecting, transforming, and storing data in your warehouse. Our article Building a Custom Data Warehouse for Enhanced Business Insights Using Amazon SP-API provides a detailed guide on how to achieve this.
In the next section, we will delve into configuring AWS Lambda and setting up your environment to work with Amazon Data API.
Introduction to AWS Lambda
What is AWS Lambda?
AWS Lambda is a serverless computing service that lets you run code without provisioning or managing servers. Essentially, it allows you to execute custom business logic in response to various events and integrates seamlessly with other AWS services.
Benefits of Using AWS Lambda
- Automatic Scaling: AWS Lambda automatically scales your application based on the number of incoming requests, ensuring that you can handle spikes in traffic without manually scaling resources.
- Cost Efficiency: You pay only for the compute time you consume, with no charges when your code isn’t running. This makes it a cost-effective solution for businesses.
- Event-driven Architecture: AWS Lambda can be triggered by various events, such as changes in data stored in Amazon Data API or HTTP requests from an API Gateway.
Use Cases for AWS Lambda
- Data Processing: Automatically process and transform data as it is ingested.
- Real-time Analytics: Perform real-time analytics on streaming data.
- Serverless Applications: Build and deploy applications without managing servers.
Setting Up AWS Lambda
Before diving into writing your custom business logic, you need to set up an environment in AWS. Here’s a step-by-step guide:
- Create an AWS Account: Sign up for Amazon Web Services if you haven’t already.
- Navigate to the AWS Lambda Console: Access the AWS Management Console and go to the AWS Lambda service.
- Create a New Function: Click on “Create function” and choose a blueprint or start from scratch.
- Configure Function Settings: Set the runtime environment, define the function name, and configure other settings like memory and timeout.
Example Lambda Function
Let’s take a look at a simple example of an AWS Lambda function that processes data from Amazon Data API:
import json
def lambda_handler(event, context):
# Retrieve data from Amazon Data API
data = event['data']
# Apply custom business logic (example: calculate total)
total = sum(data['items'])
# Return the processed data
return {
'statusCode': 200,
'body': json.dumps({'total': total})
}
This function retrieves data from an event, processes it according to a custom business rule (in this case, summing the items), and returns the processed data.
In our next section, we will explore the step-by-step process of integrating Amazon Data API with AWS Lambda to execute custom business logic.
Integrating Custom Business Logic with AWS Lambda
Step-by-Step Guide to Implementation
To implement custom business logic using Amazon Data API and AWS Lambda, follow these steps:
- Set Up Your Environment: Ensure you have an AWS account and appropriate IAM roles configured.
- Create a Lambda Function: Write your custom business logic in the chosen programming language and deploy it to AWS Lambda.
- Connect Amazon Data API: Set up triggers or event sources that will invoke your Lambda function based on data changes.
- Test and Debug: Validate the functionality with test data to ensure everything works as expected.
Preparing Your Environment
Before you start, make sure you have the necessary tools and SDKs installed on your development machine. You should also set up IAM roles with permissions to access AWS Lambda and Amazon Data API.
aws configure
This command will set up your AWS credentials and default region, making it easier to interact with AWS services.
Writing Lambda Functions in Different Languages
AWS Lambda supports several languages, including Python, Node.js, and Java. Choose the language that best fits your team’s expertise.
# Python example
def lambda_handler(event, context):
data = event['data']
processed_data = process_custom_logic(data)
return {
'statusCode': 200,
'body': json.dumps({'result': processed_data})
}
def process_custom_logic(data):
# Custom business logic
return data['value'] * 2
// Node.js example
exports.handler = async (event) => {
const data = event.data;
const processedData = await processCustomLogic(data);
return {
statusCode: 200,
body: JSON.stringify({ result: processedData })
};
};
async function processCustomLogic(data) {
// Custom business logic
return data.value * 2;
}
Example Lambda Function
Consider a scenario where you need to calculate the total sales for each product category based on incoming data from Amazon Data API. The Lambda function can process this data and return the results.
import json
def lambda_handler(event, context):
sales_data = event['sales']
total_sales = calculate_total_sales(sales_data)
return {
'statusCode': 200,
'body': json.dumps({'total_sales': total_sales})
}
def calculate_total_sales(sales_data):
totals = {}
for sale in sales_data:
category = sale['category']
amount = sale['amount']
if category not in totals:
totals[category] = 0
totals[category] += amount
return totals
Data Handling and Transformation
To ensure your Lambda function handles data efficiently, consider these best practices:
- Data Validation: Validate incoming data to ensure it meets your expected format.
- Error Handling: Implement error handling to manage exceptions and maintain a robust system.
- Data Transformation: Apply necessary transformations to the data before processing it.
In our next section, we will explore how to integrate AWS Lambda and Amazon Data API to create event-driven workflows.
Creating Custom Business Logic Functions
Writing Efficient Lambda Functions
Writing efficient Lambda functions is crucial for the performance and cost-effectiveness of your application. Here are some tips:
- Minimize External Dependencies: Avoid using large external libraries or dependencies that increase the deployment package size.
- Optimize Code Execution: Ensure your code is optimized for performance and avoids unnecessary operations.
- Use Environment Variables: Store configuration settings in environment variables to avoid hardcoding them in your code.
Best Practices for Writing Lambda Functions
- Modularity: Write modular and reusable functions to avoid code duplication.
- Concurrency Management: Consider concurrency limits and how they affect the performance of your functions.
- Logging and Monitoring: Use CloudWatch to log and monitor the execution of your Lambda functions.
Example Function with Best Practices
Let’s look at an example function that follows best practices:
import json
def lambda_handler(event, context):
# Retrieve data from Amazon Data API
sales_data = event['sales']
# Calculate total sales by category
total_sales = calculate_total_sales(sales_data)
return {
'statusCode': 200,
'body': json.dumps({'total_sales': total_sales})
}
def calculate_total_sales(sales_data):
totals = {}
for sale in sales_data:
category = sale['category']
amount = sale['amount']
if category not in totals:
totals[category] = 0
totals[category] += amount
return totals
Logging and Monitoring
To ensure that your Lambda function runs smoothly, you should set up logging and monitoring. This helps in quickly identifying and resolving issues.
aws logs create-log-group --log-group-name /aws/lambda/my-function
This command creates a log group for your Lambda function, allowing you to view and analyze logs in CloudWatch.
Integrating Amazon Data API with AWS Lambda
Connecting the Dots: Event-Driven Architecture
To integrate Amazon Data API with AWS Lambda, you can use an event-driven architecture where changes in data stored in Amazon Data API trigger your Lambda functions. This approach ensures that your custom business logic is executed automatically whenever relevant data changes.
Setting Up API Endpoints
You can use AWS API Gateway to create REST or WebSocket APIs that trigger your Lambda functions based on HTTP requests.
aws apigateway create-rest-api --name my-data-api
This command creates a new REST API that you can use to handle requests and trigger Lambda functions.
Triggering Lambda Functions
Once your API Gateway is set up, you can configure it to trigger specific Lambda functions based on different HTTP methods and routes.
aws lambda add-permission --function-name my-function --statement-id my-apigateway-statement --action 'lambda:InvokeFunction' --principal apigateway.amazonaws.com
This command grants API Gateway permission to invoke your Lambda function.
Example Integration Setup
Let’s set up an example where a POST request to /sales
triggers a Lambda function that processes the sales data.
Create API Gateway Endpoint:
aws apigateway create-resource --rest-api-id my-rest-api-id --parent-id root-resource-id --path-part sales aws apigateway put-method --rest-api-id my-rest-api-id --resource-id sales-resource-id --http-method POST --authorization-type NONE
Integrate with Lambda:
aws apigateway put-integration --rest-api-id my-rest-api-id --resource-id sales-resource-id --http-method POST --type AWS_PROXY --integration-http-method POST --uri arn:aws:apigateway:us-east-1:lambda:path/2015-03-31/functions/arn:aws:lambda:us-east-1:account-id:function:my-function/invocations
Deploy API:
aws apigateway create-deployment --rest-api-id my-rest-api-id --stage-name prod
Data Handling and Processing
To ensure efficient data handling, consider these best practices:
- Data Validation: Always validate incoming data to prevent errors and enhance security.
- Error Handling: Implement error handling mechanisms to manage exceptions gracefully.
Implementing Event-Driven Architecture
Using AWS Lambda and API Gateway Together
Event-driven architecture allows you to build highly responsive systems that automatically execute code in response to events. AWS Lambda and API Gateway work seamlessly together to create this architecture.
Setting Up Triggers
You can configure AWS Lambda functions to be triggered by various events, including API Gateway requests.
aws lambda add-permission --function-name my-function --statement-id my-apigateway-statement --action 'lambda:InvokeFunction' --principal apigateway.amazonaws.com
This command grants API Gateway permission to invoke your Lambda function.
Real-time Data Processing Workflows
Consider a scenario where you want to process real-time data as it is received by your application. AWS Lambda and API Gateway can help you set up a pipeline where incoming data triggers your custom business logic.
For example, you might use Amazon Kinesis to stream real-time data and AWS Lambda to process it on the fly. Our article How to Implement Real-Time Analytics with Amazon Kinesis and API Data provides a detailed guide on how to achieve this.
Monitoring and Logging
Monitoring the performance of your Lambda functions is crucial for maintaining a robust system. AWS CloudWatch provides comprehensive logging and monitoring capabilities.
aws logs create-log-group --log-group-name /aws/lambda/my-function
This command creates a log group for your Lambda function, allowing you to view and analyze logs in CloudWatch.
Testing and Debugging
Ensuring Your Functions Work as Expected
Testing and debugging are essential to ensure that your Lambda functions work correctly. Here are some strategies:
- Unit Testing: Write unit tests for your custom business logic to validate individual components.
- Integration Testing: Test the integration between AWS Lambda and Amazon Data API to ensure that everything works together seamlessly.
- Logging: Use CloudWatch logs to track and diagnose issues.
Common Pitfalls
Common pitfalls include:
- Incorrect Permissions: Ensure that your Lambda function has the necessary permissions to access other AWS services.
- Resource Limits: Be aware of limits such as execution time and memory allocation, which can affect performance.
Debugging Techniques
- Enable X-Ray Tracing: Use AWS X-Ray to trace and debug Lambda functions.
- Use Local Development Tools: Leverage tools like the AWS SAM CLI to test and debug your Lambda functions locally.
Scaling and Performance Optimization
Optimizing Your AWS Lambda Setup
To ensure that your application scales efficiently, consider the following strategies:
- Auto Scaling: Enable auto-scaling to automatically adjust resources based on demand.
- Cold Start Optimization: Minimize cold start times by keeping functions warm or using provisioned concurrency.
- Concurrency Limits: Manage concurrency limits to avoid unexpected costs and performance issues.
Best Practices for High-Performance Applications
- Optimize Deployment Packages: Keep your deployment packages small by removing unnecessary dependencies.
- Use Environment Variables: Store configuration settings in environment variables to avoid hardcoding them.
Real-world Use Cases
Case Studies and Examples
Real-world use cases highlight how organizations are leveraging AWS Lambda and Amazon Data API to streamline their operations.
Retail Business: Inventory Management
A retail business might use AWS Lambda and Amazon Data API to automate inventory management. When stock levels fall below a certain threshold, a Lambda function automatically triggers restocking processes.
Finance Company: Fraud Detection
A finance company could use AWS Lambda and Amazon Data API to detect fraudulent transactions in real-time. The system automatically triggers alerts based on transaction patterns indicative of fraud.
Success Stories and Best Practices
- Modularity: Break down complex business logic into smaller, reusable functions.
- Event-driven Architecture: Leverage event-driven architecture to create responsive and efficient systems.
Conclusion
Implementing custom business logic with Amazon Data API and AWS Lambda can significantly enhance your operational efficiency. By leveraging these powerful tools, you can automate complex workflows, process data in real-time, and build robust applications that meet your business needs.
Whether you are a software engineer, developer, or project manager, this guide provides practical insights and best practices to help you get started. For more detailed guides on related topics, check out our articles on Building a Custom Data Warehouse for Enhanced Business Insights Using Amazon SP-API and How to Implement Real-Time Analytics with Amazon Kinesis and API Data.
FAQs
What are the key benefits of using AWS Lambda for custom business logic?
- Key benefits include automatic scaling, cost efficiency, and the ability to execute code without managing servers. AWS Lambda also integrates seamlessly with other AWS services like Amazon Data API, making it ideal for implementing complex business rules.
How does Amazon Data API integrate with other AWS services?
- Amazon Data API integrates seamlessly with various AWS services, enabling you to build robust workflows. For instance, you can use it with Amazon S3 for data storage, DynamoDB for database operations, and AWS Lambda to execute custom business logic.
Can I use multiple languages to write Lambda functions for custom business logic?
- Yes, AWS Lambda supports several languages including Python, Node.js, and Java. This flexibility allows you to choose the language that best suits your team’s expertise.
What are the best practices for securing data when using AWS Lambda and Data API?
- Best practices include using IAM roles with minimal permissions, enabling encryption for data at rest and in transit, and leveraging AWS CloudWatch logs to monitor and audit access.
How can I monitor the performance of my Lambda functions in a production environment?
- You can use AWS CloudWatch to monitor and log the performance of your Lambda functions. Set up alerts for critical metrics, and use X-Ray to trace and debug complex workflows.
Quick Takeaways
Serverless Computing: AWS Lambda enables you to run code without managing servers, making it ideal for implementing custom business logic efficiently.
Amazon Data API Integration: Integrate Amazon Data API with AWS Lambda to handle data retrieval, processing, and transformation seamlessly, enhancing your business operations.
Event-Driven Architecture: Use AWS Lambda and API Gateway to create an event-driven architecture that triggers custom business logic in response to data changes or HTTP requests.
Scalability and Cost Efficiency: AWS Lambda automatically scales based on demand, ensuring that you can handle spikes in traffic without manual intervention and only pay for the compute time you use.
Real-World Applications: Implement real-world scenarios like inventory management, fraud detection, and real-time analytics using AWS Lambda and Amazon Data API to streamline operational processes.
Testing and Debugging: Ensure your functions work as expected by leveraging unit testing, integration testing, and CloudWatch logs for diagnostics and troubleshooting.
Security Best Practices: Secure your data by using IAM roles with minimal permissions, enabling encryption, and monitoring access logs to maintain compliance and protect sensitive information.
We’d Love to Hear from You!
We hope this guide has provided you with valuable insights into implementing custom business logic using Amazon Data API and AWS Lambda. If you have any questions, comments, or success stories to share, please leave them in the comments below. Your feedback helps us improve and create more content that meets your needs.
Don’t forget to share this article with your colleagues and friends on social media if you found it helpful. Your shares can help others learn about the power of AWS Lambda and Amazon Data API.
Engagement Question: Have you already implemented custom business logic using AWS Lambda and Amazon Data API? What challenges did you face, and how did you overcome them? Share your experiences in the comments!