· Charlotte Will · webscraping · 5 min read
How to Handle IP Blocking and Rate Limiting in Web Scraping
Discover practical strategies and best practices for handling IP blocking and rate limiting in web scraping. Learn how to implement proxy rotation, retry logic, and other advanced techniques to improve your data extraction efforts efficiently and reliably.
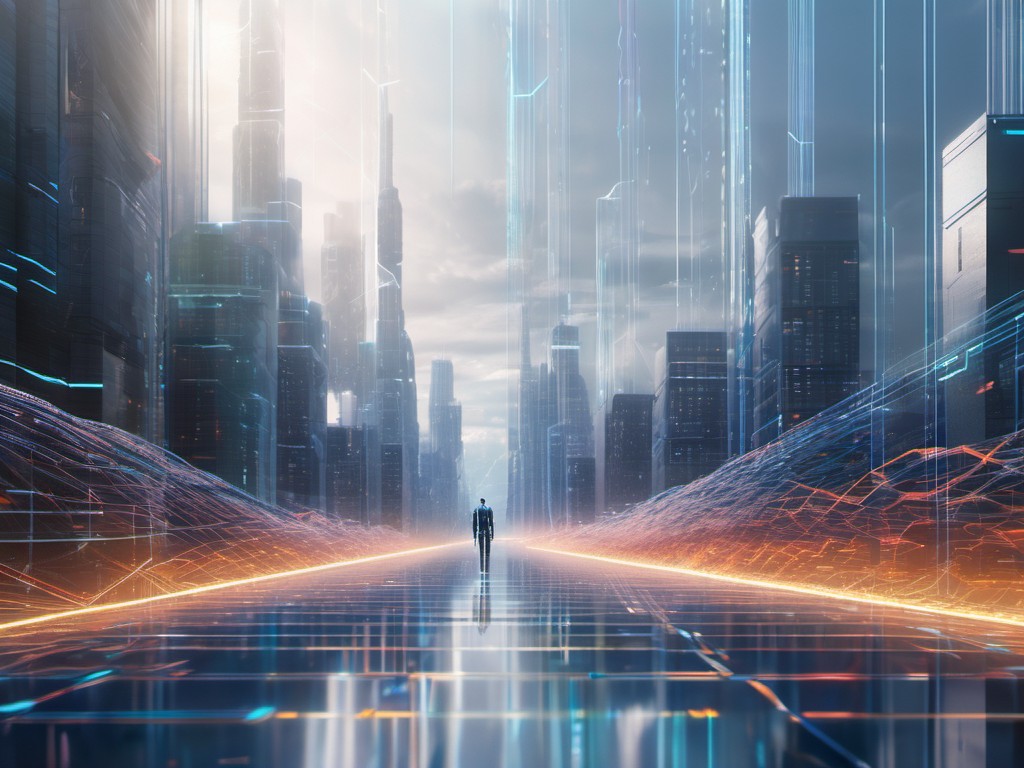
Web scraping is an essential technique for extracting data from websites, but it comes with challenges like IP blocking and rate limiting. Understanding how to handle these issues can significantly improve the efficiency and reliability of your web scraping projects. Let’s dive into practical strategies and best practices for managing IP blocking and rate limiting.
Understanding IP Blocking in Web Scraping
IP blocking occurs when a website detects repeated requests from the same IP address within a short period. To protect against malicious activities, websites may block your IP temporarily or permanently. Handling IP blocking effectively is crucial for maintaining access to the data you need.
Common Reasons for IP Blocking
- Frequent Requests: Making too many requests in a short time can trigger anti-scraping mechanisms.
- Suspicious Activities: Behaviors like rapid page changes, form submissions, or JavaScript execution can raise red flags.
- Violation of Terms of Service: Some websites explicitly prohibit scraping and may block IPs associated with such activities.
Best Practices to Avoid IP Blocking
Use Proxy Rotation
Rotating proxies is one of the most effective ways to avoid IP blocking. By using a pool of different IP addresses, you can distribute your requests, making them less likely to be detected and blocked.
Implementing Proxy Rotation in Python
import requests
from fake_useragent import UserAgent
proxies = ['http://proxy1:port', 'http://proxy2:port']
headers = {'User-Agent': UserAgent().random}
def fetch(url):
for proxy in proxies:
try:
response = requests.get(url, headers=headers, proxies={'http': proxy, 'https': proxy})
if response.status_code == 200:
return response.text
except Exception as e:
print(f"Error with proxy {proxy}: {e}")
return None
Retry Logic
Implementing retry logic helps in handling temporary IP blocks or network issues gracefully. By reattempting requests after a delay, you can increase the chances of success without overwhelming the server.
Example with Retry Logic
import time
from requests.exceptions import RequestException
def fetch_with_retry(url):
attempts = 3
for attempt in range(attempts):
try:
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.text
except RequestException as e:
print(f"Request failed with error {e}. Retrying ({attempt + 1}/{attempts})...")
time.sleep(5)
return None
User-Agent Rotation
Websites often track and block requests based on the User-Agent string. Rotating User-Agents can help distribute your scraping activities more evenly, making it harder to detect and block your requests.
Rotating User-Agents with fake_useragent
Library
from fake_useragent import UserAgent
ua = UserAgent()
headers = {'User-Agent': ua.random}
def fetch(url):
try:
response = requests.get(url, headers=headers)
return response.text if response.status_code == 200 else None
except Exception as e:
print(f"Error fetching {url}: {e}")
return None
Handling Rate Limiting in Web Scraping
Rate limiting is a technique used by websites to control the number of requests made from a single IP address over a specified period. Respecting rate limits is essential for maintaining access and avoiding IP blocks.
Understanding API Rate Limits
API rate limits are typically defined in terms of request quotas per time interval (e.g., 1000 requests per hour). Exceeding these limits can result in temporary or permanent bans.
Best Practices for Handling API Rate Limits
Check Rate Limit Headers
Many APIs include rate limit information in HTTP response headers. By parsing these headers, you can monitor your usage and adjust your scraping rate accordingly.
response = requests.get(url)
rate_limit_remaining = int(response.headers.get('X-RateLimit-Remaining', 0))
rate_limit_reset = int(response.headers.get('X-RateLimit-Reset', 0))
Implement Backoff Strategies
Backoff strategies involve pausing your requests when you approach the rate limit. This can help prevent exceeding limits and potential IP blocks.
import time
def fetch_with_backoff(url):
attempts = 3
for attempt in range(attempts):
response = requests.get(url)
if response.status_code == 429: # HTTP status code for rate limiting
retry_after = int(response.headers.get('Retry-After', 60))
print(f"Rate limit exceeded, retrying after {retry_after} seconds...")
time.sleep(retry_after)
else:
return response.text
return None
Advanced Techniques for Handling IP Blocking and Rate Limiting
Using Headless Browsers
Headless browsers like Selenium can mimic human behavior, making it harder for websites to detect and block your requests. They also handle JavaScript rendering, which is crucial for scraping dynamic content.
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
def fetch_with_selenium(url):
options = Options()
options.headless = True
driver = webdriver.Chrome(options=options)
driver.get(url)
return driver.page_source
Integrating Retry Logic with Proxy Rotation
Combining retry logic and proxy rotation can provide a robust solution for handling IP blocks and rate limits effectively. This approach ensures that your scraping activities are distributed across multiple IP addresses, reducing the likelihood of detection and blocking.
import time
from requests.exceptions import RequestException
def fetch_with_retry_and_proxies(url):
attempts = 3
proxies = ['http://proxy1:port', 'http://proxy2:port']
for attempt in range(attempts):
for proxy in proxies:
try:
response = requests.get(url, headers=headers, proxies={'http': proxy, 'https': proxy})
if response.status_code == 200:
return response.text
except RequestException as e:
print(f"Request failed with error {e}. Retrying ({attempt + 1}/{attempts})...")
time.sleep(5)
return None
Conclusion
Handling IP blocking and rate limiting is essential for successful web scraping projects. By implementing proxy rotation, retry logic, User-Agent rotation, and backoff strategies, you can significantly improve the efficiency and reliability of your data extraction efforts. Additionally, consider using headless browsers and integrating advanced techniques to stay ahead in the dynamic landscape of web scraping.
FAQs
What is IP blocking, and why does it happen?
- IP blocking occurs when a website detects repeated requests from the same IP address within a short period, often leading to temporary or permanent blocks. This happens to protect against malicious activities and excessive resource usage.
How can proxy rotation help in web scraping?
- Proxy rotation distributes your requests across multiple IP addresses, making them less likely to be detected and blocked by the target website.
What is rate limiting, and how do APIs enforce it?
- Rate limiting controls the number of requests made from a single IP address over a specified period. APIs typically enforce this through HTTP headers that define quotas and reset times for request limits.
How can I implement retry logic in my web scraping project?
- Retry logic involves reattempting requests after a delay, helping to handle temporary IP blocks or network issues gracefully. You can implement this by wrapping your request code in a loop with a sleep interval between retries.
What are the benefits of using headless browsers for web scraping?
- Headless browsers like Selenium mimic human behavior, making it harder for websites to detect and block your requests. They also handle JavaScript rendering, which is crucial for scraping dynamic content.