· Charlotte Will · Amazon API · 4 min read
How to Fetch Product Information Using Amazon PA-API 5.0
Discover how to fetch product information using Amazon PA-API 5.0 with our comprehensive guide. Learn step-by-step setup, making API requests, handling responses, best practices, and more for seamless integration into your projects. Perfect for developers looking to leverage Amazon's Product Advertising API effectively.
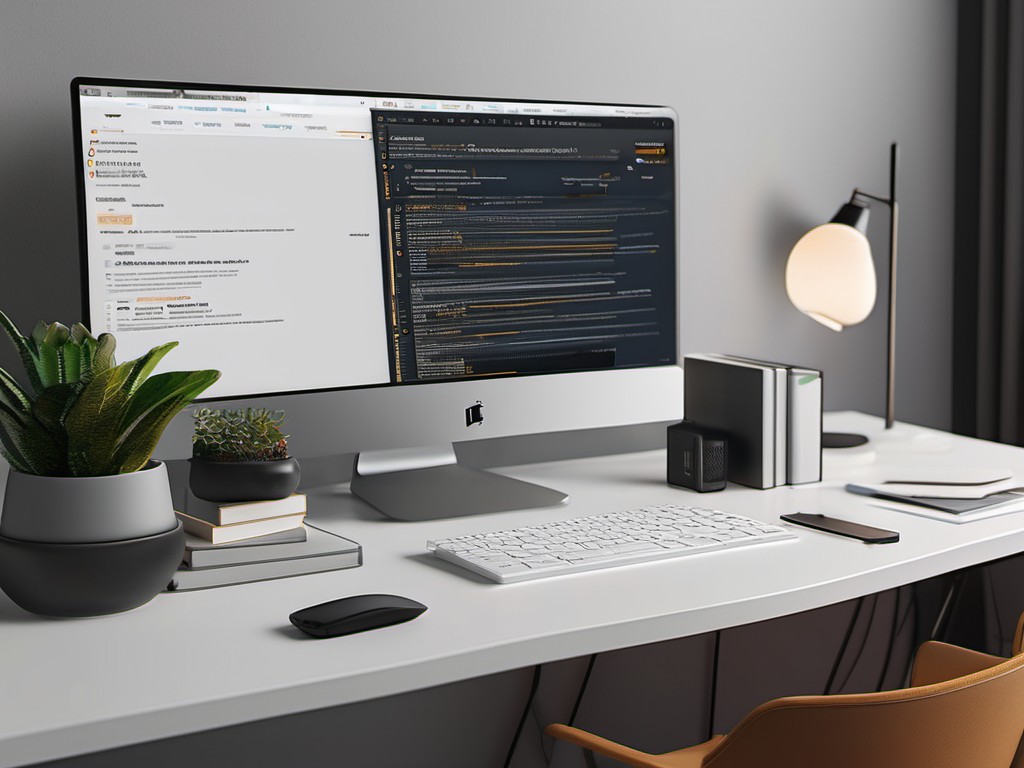
Introduction to Amazon PA-API 5.0
What is Amazon PA-API?
Amazon’s Product Advertising API (PA-API) allows developers to programmatically access product information, including details like price, availability, and customer reviews. This API is invaluable for integrating Amazon products into your website or application, offering a seamless shopping experience for users.
Benefits of Using PA-API 5.0
PA-API 5.0 comes with several enhancements over its predecessors:
- Enhanced Security: Improved authentication methods ensure data integrity and security.
- Rich Data Access: Detailed product information, including high-resolution images and comprehensive descriptions.
- Scalability: Supports large-scale applications with robust performance.
Setting Up Your Environment for PA-API
Prerequisites
Before diving into the setup process, ensure you have:
- An Amazon Associates account.
- AWS credentials (Access Key and Secret Key).
- Basic knowledge of a programming language like Python or JavaScript.
Step-by-Step Setup Guide
1. Register for PA-API
First, sign up for the Amazon Product Advertising API. You’ll need to have an Amazon Associates account to proceed.
2. Get Your Credentials
Once registered, you’ll receive your Access Key and Secret Key. These are crucial for making authenticated API requests.
3. Set Up Your Development Environment
- Python: Install the necessary libraries using pip:
pip install boto3
- JavaScript: Use npm to install AWS SDK:
npm install aws-sdk
Fetching Product Information with PA-API
Making API Requests
To fetch product information, you need to make a signed request to the Amazon PA-API endpoint. Below is an example using Python and Boto3:
import boto3
from botocore.config import Config
# Configure your AWS credentials
AWS_ACCESS_KEY = 'your_access_key'
AWS_SECRET_KEY = 'your_secret_key'
REGION_NAME = 'us-east-1' # Change if necessary
client = boto3.client(
'productadvertising',
aws_access_key_id=AWS_ACCESS_KEY,
aws_secret_access_key=AWS_SECRET_KEY,
region_name=REGION_NAME,
config=Config(signature_version='v4')
)
def fetch_product_info(asin):
try:
response = client.get_items(ItemIds=[asin], Resources=['Images.Primary.Medium', 'Offers.Listings.Price'])
return response
except Exception as e:
print(f"Error fetching product info: {e}")
return None
# Example usage
product_info = fetch_product_info('B08N5WRWNW') # Replace with actual ASIN
print(product_info)
Handling API Responses
Once you receive the response, parse it to extract the required product details. Here’s how you can handle the JSON response in Python:
import json
def display_product_info(response):
if 'ItemsResult' in response and 'Items' in response['ItemsResult']:
for item in response['ItemsResult']['Items']:
print("Product Title:", item.get('Title', 'N/A'))
print("Price:", item.get('Offers', {}).get('Listings', [{}])[0].get('Price', {}).get('DisplayAmount', 'N/A'))
if 'Images' in item:
print("Image URL:", item['Images']['Primary']['Medium']['URL'])
else:
print("No items found.")
# Example usage with the fetched product info
display_product_info(product_info)
Best Practices and Tips
Error Handling
Always implement robust error handling to manage API request failures. Common errors include rate limits, invalid credentials, or service unavailability.
import botocore
def fetch_product_info(asin):
try:
response = client.get_items(ItemIds=[asin], Resources=['Images.Primary.Medium', 'Offers.Listings.Price'])
return response
except botocore.exceptions.ClientError as e:
print(f"Client error occurred: {e}")
return None
except botocore.exceptions.BotoCoreError as e:
print(f"BotoCore error occurred: {e}")
return None
Rate Limiting
Be mindful of the rate limits imposed by Amazon PA-API to avoid being throttled or blocked. Implementing a delay between requests can help mitigate this issue.
import time
def fetch_product_info_with_delay(asins):
results = []
for asin in asins:
product_info = fetch_product_info(asin)
if product_info:
results.append(product_info)
time.sleep(1) # Adding a delay to avoid hitting rate limits
return results
FAQs on Amazon PA-API 5.0
Conclusion
Integrating the Amazon Product Advertising API into your projects can significantly enhance user experience by providing up-to-date product information directly from Amazon. By following this guide, you’ve learned how to set up your environment, make authenticated requests, handle responses, and implement best practices for using PA-API 5.0 effectively.
FAQs
1. How do I get access to the Amazon Product Advertising API? You need an active Amazon Associates account. Once you have that, you can sign up for the API through your Associates dashboard.
2. What are the rate limits for PA-API 5.0? The exact rate limits depend on your usage and may vary. Always refer to the official documentation or contact Amazon support for specific details.
3. Can I use PA-API in any programming language? Yes, you can use PA-API with various languages including Python, JavaScript, Java, PHP, etc., as long as they support HTTP requests and AWS SDKs.
4. How do I handle authentication errors? Ensure your Access Key and Secret Key are correct and have the necessary permissions. Also, verify that your API request is correctly signed.
5. What resources can I fetch using PA-API 5.0? PA-API 5.0 allows you to fetch a wide range of product details including titles, descriptions, images, prices, customer reviews, and more.