· Charlotte Will · webscraping · 4 min read
How to Extract Images from a Website with Web Scraping
Discover how to extract images from websites using web scraping techniques with Python, BeautifulSoup, and Selenium. Learn practical steps, ethical considerations, and advanced methods in this detailed guide tailored for both beginners and experienced users.
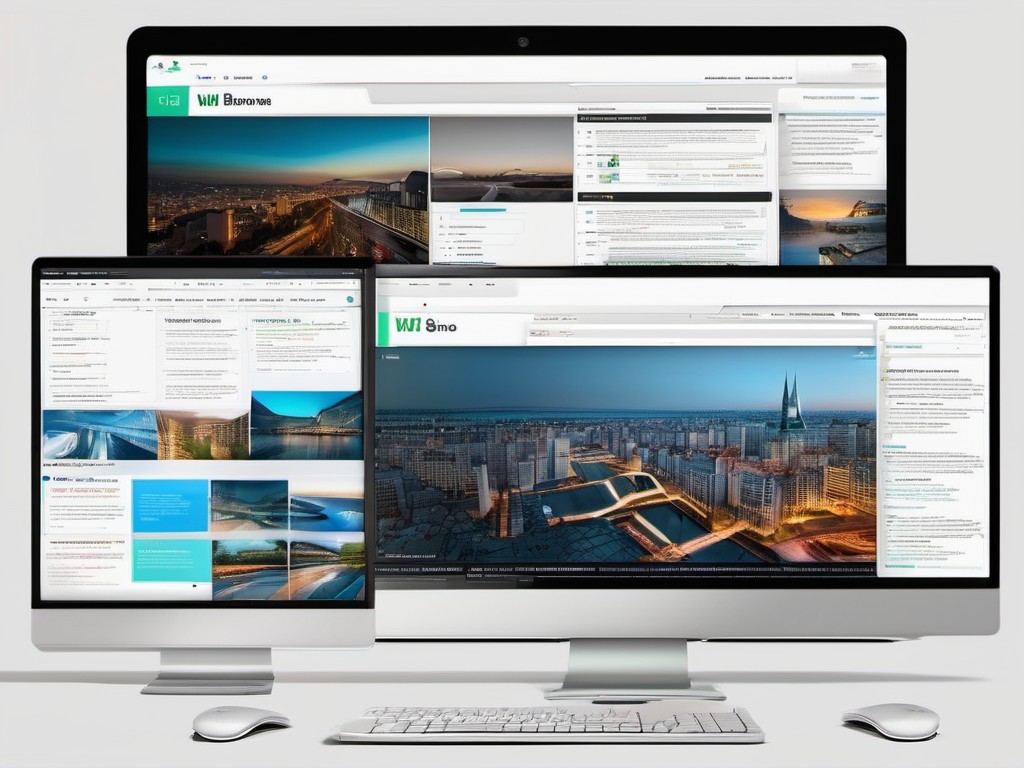
Extracting images from websites can be incredibly useful for various tasks, whether you’re collecting data for research, building an image dataset for machine learning models, or simply archiving visual content. Web scraping is the go-to technique for this task. Let’s dive into how to extract images from a website using web scraping.
Understanding Web Scraping for Images
Web scraping involves automatically collecting data from websites. When it comes to image extraction, web scraping tools can systematically download images based on specified criteria. This process is invaluable for gathering large volumes of visual content efficiently.
Why Extract Images?
- Data Collection: Useful for research projects requiring extensive image datasets.
- Machine Learning: Essential for training models that rely on visual data.
- Market Analysis: Helps in analyzing competitor websites by scraping product images.
Tools Needed for Image Extraction
To extract images from a website, you’ll need some essential tools:
1. Python
Python is the most commonly used programming language for web scraping due to its simplicity and powerful libraries.
2. BeautifulSoup
A library that helps parse HTML and XML documents. It’s great for extracting data from web pages.
3. Requests
An HTTP library that allows you to send requests to a server and receive the response. Perfect for downloading web content.
4. Selenium (Optional)
A tool used for automating web browsers, particularly useful for scraping dynamic websites with JavaScript-rendered content.
Step-by-Step Guide to Scrape Images from a Website
Setting Up Your Environment
- Install Python: Make sure you have Python installed on your computer. You can download it from python.org.
- Install Required Libraries:
pip install requests beautifulsoup4
Writing the Web Scraping Script
Here’s a simple example of how to scrape images using Python, BeautifulSoup, and Requests:
- Import Necessary Libraries:
import requests from bs4 import BeautifulSoup import os
- Define the URL and Request Headers:
url = "https://example.com" # Replace with your target website headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36' }
- Send a Request and Parse the HTML:
response = requests.get(url, headers=headers) soup = BeautifulSoup(response.content, 'html.parser')
- Extract Image URLs:
images = soup.find_all('img') image_urls = [img['src'] for img in images if 'src' in img.attrs]
- Download and Save Images:
os.makedirs("images", exist_ok=True) # Create a directory to save the images for i, image_url in enumerate(image_urls): try: response = requests.get(image_url, headers=headers) with open(f"images/image_{i}.jpg", "wb") as f: f.write(response.content) except Exception as e: print(f"Failed to download {image_url}: {e}")
Running and Debugging the Script
- Run Your Script: Save your script as
scraper.py
and run it using Python.python scraper.py
- Debugging Issues: Common issues include network errors, incorrect URLs, or changes in website structure. Use print statements to debug step-by-step.
Ethical Considerations in Web Scraping
While web scraping can be highly beneficial, it’s essential to consider the ethical and legal aspects:
1. Legalities:
- Always check the website’s
robots.txt
file for restrictions on web crawling. - Ensure you comply with the site’s terms of service.
2. Ethical Guidelines:
- Respect the website’s bandwidth by not sending too many requests in a short time.
- Use scraping responsibly and ethically, avoiding any actions that could be seen as malicious or harmful.
Advanced Techniques for Image Extraction
Handling Dynamic Websites:
Some websites use JavaScript to load content dynamically. For these sites, you’ll need a tool like Selenium:
- Install Selenium and WebDriver:
pip install selenium
- Set Up the Script with Selenium:
from selenium import webdriver from bs4 import BeautifulSoup import os url = "https://example.com" # Replace with your target website driver_path = "/path/to/chromedriver" # Path to your WebDriver options = webdriver.ChromeOptions() options.add_argument('--headless') driver = webdriver.Chrome(executable_path=driver_path, options=options) driver.get(url) soup = BeautifulSoup(driver.page_source, 'html.parser') images = soup.find_all('img') image_urls = [img['src'] for img in images if 'src' in img.attrs] os.makedirs("images", exist_ok=True) for i, image_url in enumerate(image_urls): try: response = requests.get(image_url, headers=headers) with open(f"images/image_{i}.jpg", "wb") as f: f.write(response.content) except Exception as e: print(f"Failed to download {image_url}: {e}")
Dealing with CAPTCHAs:
CAPTCHAs are designed to prevent automated access. Handling them often requires manual intervention or advanced techniques like machine learning models to solve the CAPTCHA automatically.
FAQ Section
Is web scraping legal?
Yes, but it depends on the website’s terms of service and local laws. Always check robots.txt
and consult a legal professional if unsure.
What are the best tools for image extraction?
Python with libraries like BeautifulSoup, Requests, and Selenium are among the best tools for web scraping images.
How do I handle large-scale scraping projects?
For large-scale projects, consider using distributed systems or cloud services to manage computational resources efficiently. Also, implement rate limiting to avoid overloading the target server.
Can I use Selenium for all types of websites?
Selenium is particularly useful for dynamic websites that rely on JavaScript. For simpler sites, BeautifulSoup and Requests might be sufficient.
What should I do if a website blocks my scraping attempts?
If you encounter blocking, try using proxies or rotating your IP address. However, always ensure you are not violating the site’s terms of service.
Conclusion
Extracting images from websites using web scraping can be a powerful tool for various applications. By following this guide, you’ll have the essential skills and knowledge to start your own image extraction projects. Always remember to act ethically and legally when scraping data. Happy scraping!