· Charlotte Will · 6 min read
How to Create a Custom Amazon Scraper Tool Using Python
Learn how to create a custom Amazon scraper tool using Python in this comprehensive guide. Master web scraping techniques, handle errors effectively, and store data efficiently using popular libraries like Requests, BeautifulSoup, and Pandas. Perfect for businesses seeking competitive intelligence or price monitoring from the world's largest e-commerce platform.
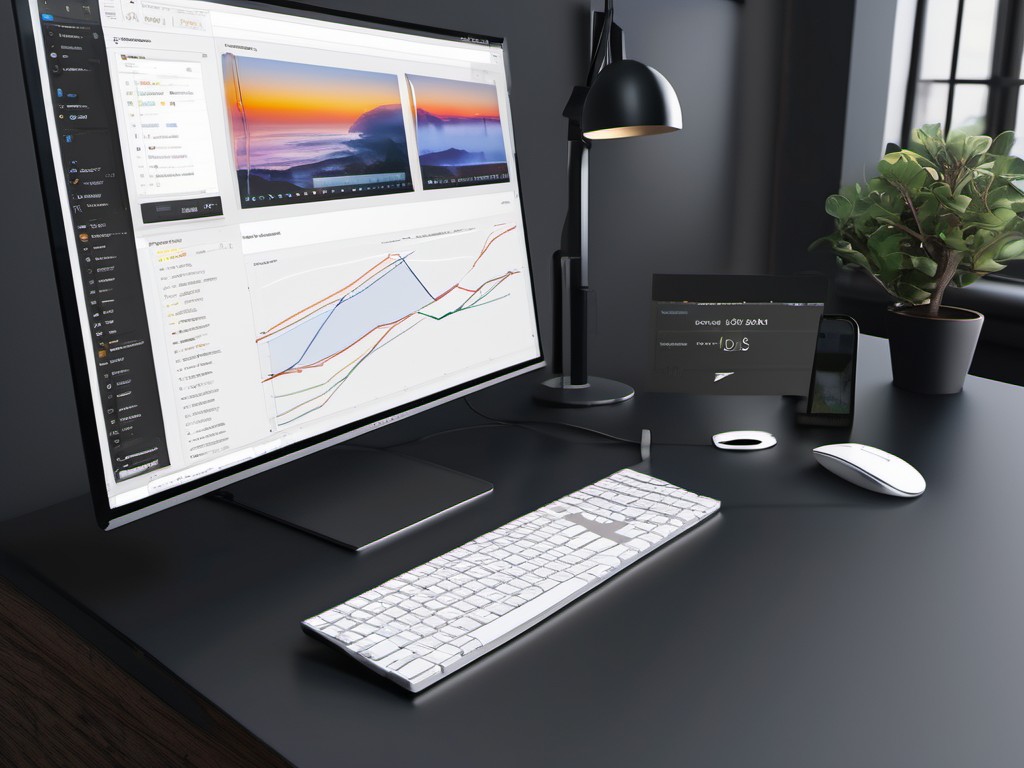
Welcome to our comprehensive guide on how to create a custom Amazon scraper tool using Python. Web scraping is an essential skill for extracting valuable data from websites, and Amazon, with its vast repository of product information, makes it an ideal target for this technique. Let’s dive into the world of web scraping and learn how to build a powerful tool that can fetch and parse product details from Amazon.
Introduction to Web Scraping
Web scraping involves extracting data from websites programmatically. This process is invaluable for businesses seeking to gather competitive intelligence, monitor prices, or collect product information. When it comes to e-commerce giants like Amazon, web scraping can provide a wealth of insights that are otherwise difficult or time-consuming to obtain manually.
Setting Up Your Environment
Before we get into the nitty-gritty of building our Amazon scraper, let’s set up our development environment. We’ll need Python installed on our system, along with several essential libraries for web scraping and data manipulation.
Installing Necessary Libraries
First, ensure you have Python installed. You can download it from the official website. Once Python is set up, we’ll use pip
to install our required libraries:
pip install requests beautifulsoup4 pandas
- Requests: For making HTTP requests to fetch web pages.
- BeautifulSoup4: For parsing HTML and extracting data.
- Pandas: For data manipulation and storage in a structured format like CSV or Excel.
Writing the Scraper Code
Now that our environment is ready, let’s write some code to build our Amazon scraper. We’ll start by creating a script that fetches product pages from Amazon and extracts relevant details.
Fetching Product Pages
We’ll use the requests
library to fetch product pages. Here’s a basic example of how to do this:
import requests
from bs4 import BeautifulSoup
# URL of the Amazon product page
url = 'https://www.amazon.com/dp/B08N5WRWB9'
# Send a GET request to the webpage
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
page_content = response.text
else:
print("Failed to retrieve the webpage")
Extracting Data from HTML
Next, we’ll use BeautifulSoup to parse the HTML content and extract relevant data. Let’s assume we want to scrape the product title, price, and customer rating.
soup = BeautifulSoup(page_content, 'html.parser')
# Extract product title
product_title = soup.find('span', id='productTitle').get_text()
# Extract product price
price_container = soup.find('span', class_='a-price-whole')
if price_container:
product_price = price_container.get_text().strip()
else:
product_price = "Price not available"
# Extract customer rating
rating_container = soup.find('span', class_='a-icon-alt')
if rating_container:
product_rating = rating_container.get_text().strip()
else:
product_rating = "Rating not available"
print(f'Product Title: {product_title}')
print(f'Product Price: ${product_price}')
print(f'Customer Rating: {product_rating}')
Handling Multiple Pages and Products
To scrape data from multiple pages or products, we can use a loop. Let’s say you have a list of product URLs:
import requests
from bs4 import BeautifulSoup
# List of product URLs
product_urls = [
'https://www.amazon.com/dp/B08N5WRWB9',
'https://www.amazon.com/dp/B01LTHP3YQ',
# Add more URLs as needed
]
# Function to scrape product details
def scrape_product(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
product_title = soup.find('span', id='productTitle').get_text()
price_container = soup.find('span', class_='a-price-whole')
product_price = price_container.get_text().strip() if price_container else "Price not available"
rating_container = soup.find('span', class_='a-icon-alt')
product_rating = rating_container.get_text().strip() if rating_container else "Rating not available"
return {
'title': product_title,
'price': product_price,
'rating': product_rating
}
else:
return None
# Scrape all products and store the results in a list
products = []
for url in product_urls:
product_data = scrape_product(url)
if product_data:
products.append(product_data)
Handling Errors and Exceptions
Scraping websites often involves dealing with unpredictable changes to the site’s structure or temporary issues like network errors. We can handle these situations using try-except blocks:
def scrape_product(url):
try:
response = requests.get(url)
response.raise_for_status() # Raise an HTTPError for bad responses
soup = BeautifulSoup(response.text, 'html.parser')
product_title = soup.find('span', id='productTitle').get_text()
price_container = soup.find('span', class_='a-price-whole')
product_price = price_container.get_text().strip() if price_container else "Price not available"
rating_container = soup.find('span', class_='a-icon-alt')
product_rating = rating_container.get_text().strip() if rating_container else "Rating not available"
return {
'title': product_title,
'price': product_price,
'rating': product_rating
}
except requests.exceptions.RequestException as e:
print(f"Error fetching {url}: {e}")
return None
Storing Data in a Structured Format
Finally, let’s store the scraped data in a CSV file using Pandas for easy analysis and storage.
import pandas as pd
# Convert list of products to a DataFrame
df = pd.DataFrame(products)
# Save DataFrame to a CSV file
df.to_csv('amazon_products.csv', index=False)
Best Practices for Amazon Web Scraping
While web scraping can be incredibly powerful, it’s essential to follow best practices to avoid legal issues and ensure the sustainability of your scraper:
Respect Robots.txt
Always check a website’s robots.txt
file to understand its crawling policies. Amazon typically allows crawling but may restrict certain areas of their site.
Use Headers and User-Agent Strings
Mimic a real browser by setting appropriate headers and user-agent strings in your requests to avoid being blocked.
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
Handle Rotating Proxies and Captchas
To bypass IP bans or captcha challenges, consider using rotating proxies or services that can handle captchas for you.
Conclusion
Creating a custom Amazon scraper tool using Python is an excellent way to extract valuable product data from one of the largest e-commerce platforms in the world. By following the steps outlined above and adhering to best practices, you can build a powerful web scraper that fetches and parses product details efficiently.
Remember to always respect the legal and ethical guidelines surrounding web scraping. With careful consideration and responsible use, your Amazon scraper can become an invaluable tool for gathering competitive intelligence or monitoring market trends.
FAQs
Is web scraping legal?
Web scraping itself is not inherently illegal, but the legality depends on how you use it. Always respect the website’s terms of service and robots.txt file. Avoid scraping sensitive information or causing harm to the website by overloading its servers.
How do I handle captchas on Amazon?
Handling captchas can be challenging. Some services provide captcha-solving capabilities, which you can integrate into your scraper. Alternatively, using rotating proxies and user-agent strings can help reduce the likelihood of encountering captchas.
What are some best practices for data storage after scraping?
After scraping, store your data in a structured format like CSV or a database for easy retrieval and analysis. Regularly clean and update your data to ensure its accuracy and relevance.
Can I scrape Amazon without getting blocked?
While it’s difficult to guarantee you won’t get blocked, following best practices such as respecting the site’s policies, using realistic headers, and implementing rate limits can help minimize the risk. Using rotating proxies is also an effective strategy.
How often should I update my Amazon scraper?
Website structures change frequently, so it’s essential to periodically update your scraper to adapt to these changes. Regularly monitor your scraper’s performance and adjust the code as needed to ensure continued success.