· Charlotte Will · webscraping · 4 min read
How to Build a Web Scraper Using Django and BeautifulSoup
Learn how to build a web scraper using Django and BeautifulSoup in this step-by-step guide. Discover practical tips for extracting data from websites, handling changing structures, and best practices for respectful scraping. Perfect for beginners and experienced developers alike.
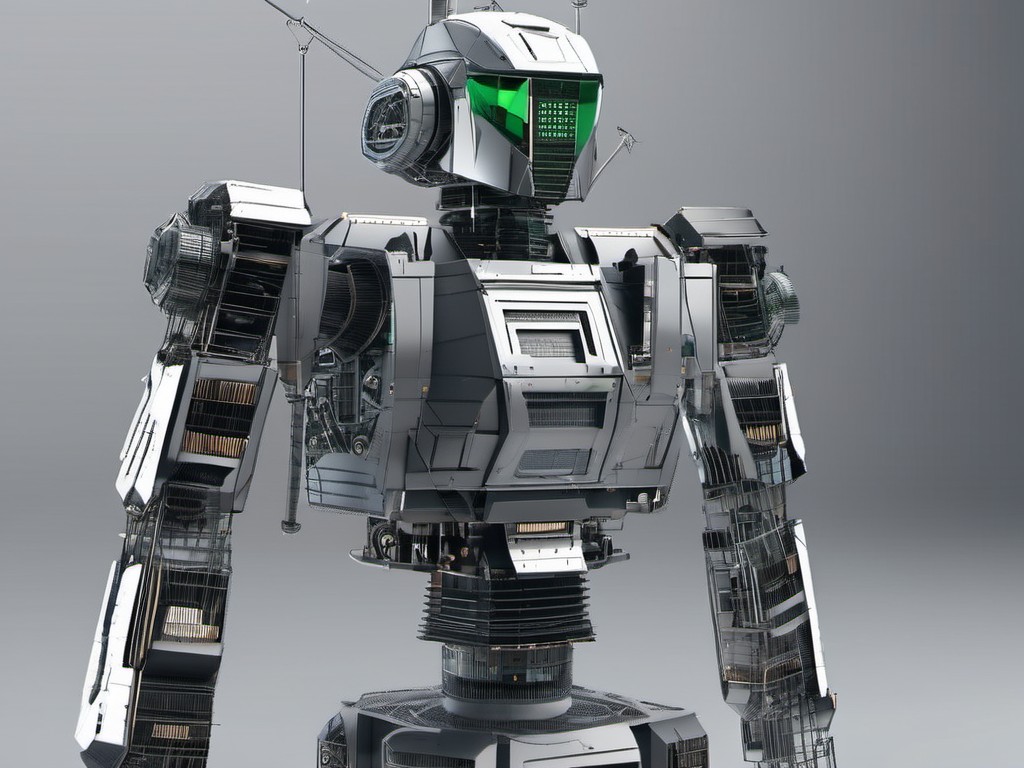
Web scraping is an essential skill in the age of big data, enabling you to extract valuable information from websites. This guide will walk you through building a web scraper using Django and BeautifulSoup, two powerful tools that simplify this process. Whether you’re new to web scraping or looking to enhance your skills, this tutorial offers practical insights for both beginners and experienced developers.
Why Use Django and BeautifulSoup?
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Combined with BeautifulSoup—a Python library for parsing HTML and XML documents—you can create robust web scrapers efficiently.
Prerequisites
Before diving into the tutorial, ensure you have the following:
- Basic understanding of Python
- Django installed on your system
- A text editor or IDE (such as VS Code or PyCharm)
If you haven’t installed Django yet, you can do so with pip:
pip install django beautifulsoup4 requests
Setting Up Your Project
Create a New Django Project:
django-admin startproject myscraper cd myscraper
Start a New App within the Project:
python manage.py startapp scraper
Add the App to
INSTALLED_APPS
insettings.py
:INSTALLED_APPS = [ ... 'scraper', ]
Building the Web Scraper
1. Define Your Model
In Django, models define the structure of your data. For a web scraper, you might want to store scraped content like blog posts or product details.
# scraper/models.py
from django.db import models
class ScrapedData(models.Model):
title = models.CharField(max_length=200)
description = models.TextField()
date = models.DateTimeField()
2. Create Views for Scraping
Views handle the logic for fetching data from websites and processing it with BeautifulSoup.
# scraper/views.py
import requests
from bs4 import BeautifulSoup
from .models import ScrapedData
def scrape_website(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract data
title = soup.find('h1').get_text()
description = soup.find('p').get_text()
date = datetime.now() # Example placeholder
# Save data to database
ScrapedData(title=title, description=description, date=date).save()
3. Define URLs and Routes
You need a way to trigger the scraping process. Let’s create a simple endpoint for this.
# scraper/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('scrape/', views.scrape_website, name='scrape'),
]
Don’t forget to include the app’s URLs in your project’s main urls.py
file:
# myscraper/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('scrape/', include('scraper.urls')),
]
4. Create Templates for Output
While not required, creating templates can make it easier to visualize the scraped data.
<!-- scraper/templates/scrape_result.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Scraped Data</title>
</head>
<body>
<h1>{{ title }}</h1>
<p>{{ description }}</p>
<p><small>{{ date }}</small></p>
</body>
</html>
5. Create Views to Render Templates
You can now render the scraped data in a template.
# scraper/views.py (continued)
from django.shortcuts import render
def show_scraped_data(request):
data = ScrapedData.objects.all()
return render(request, 'scrape_result.html', {'title': data[0].title, 'description': data[0].description, 'date': data[0].date})
Add a URL pattern for this view:
# scraper/urls.py (continued)
urlpatterns = [
path('scrape/', views.scrape_website, name='scrape'),
path('result/', views.show_scraped_data, name='result'),
]
Running the Scraper
Migrate Your Database:
python manage.py makemigrations python manage.py migrate
Run the Development Server:
python manage.py runserver
Navigate to
http://127.0.0.1:8000/scrape/
in your browser to trigger the scraper.View the Scraped Data: Navigate to
http://127.0.0.1:8000/result/
to see the result rendered in a template.
Best Practices for Web Scraping
1. Respect Robots.txt
Always check the website’s robots.txt
file before scraping to ensure you’re not violating any rules.
2. Be Polite with Requests
Add delays between requests and avoid hammering the server too hard. Libraries like time
can help with this.
3. Handle Exceptions Gracefully
Use try-except blocks to manage potential errors, such as network issues or changes in webpage structure.
Conclusion
Congratulations! You’ve built a basic web scraper using Django and BeautifulSoup. This tutorial covered the essentials of setting up your project, defining models, creating views for scraping, and rendering the results. Remember to follow best practices to ensure your scraping activities are legal and respectful.
FAQ
1. Can I Scrape Any Website?
While technically possible, it’s essential to respect the website’s terms of service and the legal implications of web scraping. Always check robots.txt
and consider seeking permission if needed.
2. How Do I Handle Changing Website Structures?
Website structures can change over time, breaking your scraper. Use flexible selectors in BeautifulSoup and implement error handling to manage such scenarios effectively.
3. Can I Scrape Data from Websites That Require Login?
Yes, you can use libraries like requests
with session objects or Selenium for more complex interactions that require login.
4. How Do I Avoid Getting Blocked by the Website?
Use rotating proxies and user agents to mimic human behavior and avoid detection. Additionally, implement delays between requests to reduce the load on the server.
5. What Are Some Advanced Techniques for Web Scraping?
Advanced techniques include using headless browsers like Puppeteer or Selenium for dynamic content, handling CAPTCHAs, and scraping data from APIs when available.