· Charlotte Will · 4 min read
How to Build a Robust Amazon Scrape using GitHub Repositories
Learn how to build a robust Amazon scraper using GitHub repositories with our step-by-step guide. Discover best practices, handle anti-scraping measures, and leverage advanced techniques like rotating proxies and headless browsers for effective web scraping.
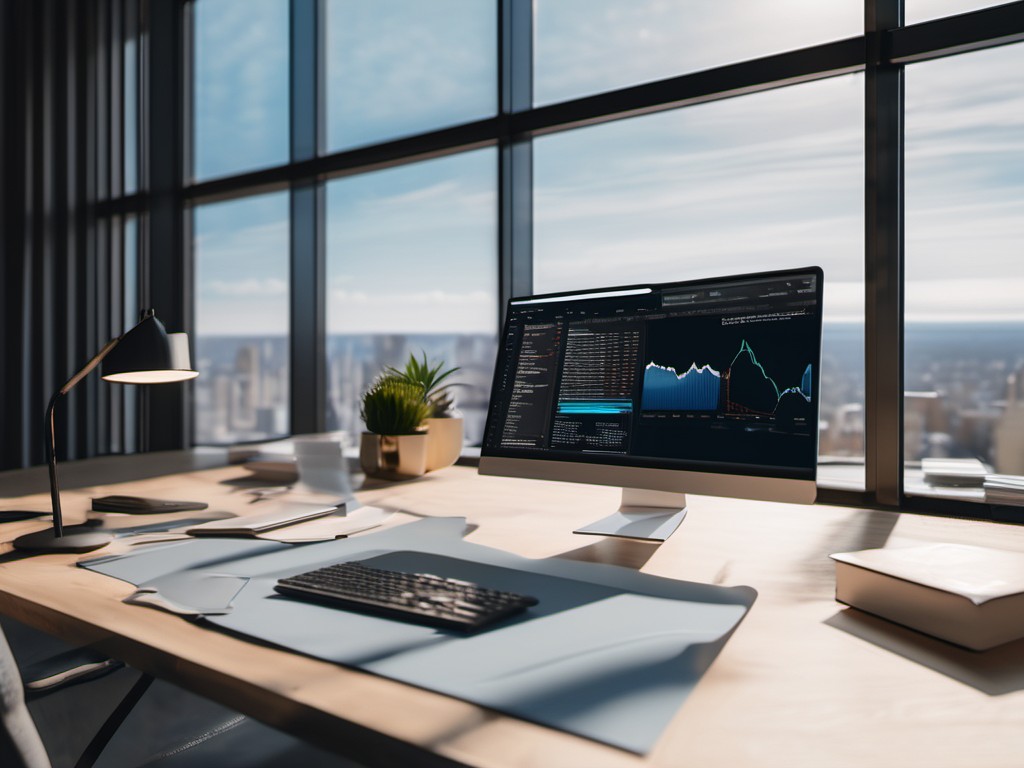
Introduction to Amazon Scraping
Web scraping has become an essential tool in data collection and analysis, especially for e-commerce platforms like Amazon. By extracting valuable information such as product prices, reviews, and ratings, businesses can gain a competitive edge. However, building a robust Amazon scraper requires careful planning and the right tools. GitHub repositories offer a treasure trove of pre-built scripts and libraries that can simplify this process significantly.
Why Choose GitHub Repositories?
GitHub is a goldmine for developers looking to leverage existing code. Here’s why:
- Open Source: Many repositories are open source, meaning you can use, modify, and distribute the code freely.
- Community Support: GitHub has an active community where you can find help, report issues, and share improvements.
- Pre-Built Solutions: Repositories often come with pre-built solutions that save time and effort.
- Learning Opportunities: Reading through others’ code can provide valuable insights into best practices.
Setting Up Your Environment
Before diving into the coding part, you need to set up your environment correctly.
Required Tools and Libraries
- Python: Python is a popular choice for web scraping due to its simplicity and powerful libraries.
- BeautifulSoup: A library that makes it easy to scrape information from web pages.
- Requests: Used to send HTTP requests to fetch the web page content.
- Selenium: For handling dynamic content that requires a browser.
- Pandas: Useful for data manipulation and analysis after scraping.
You can install these using pip:
pip install beautifulsoup4 requests selenium pandas
Step-by-Step Guide to Building an Amazon Scraper
Configuring the Scraper
Import Libraries: Start by importing necessary libraries.
from bs4 import BeautifulSoup import requests import pandas as pd
Fetch the Web Page: Use
requests
to fetch the web page content.url = 'https://www.amazon.com/s?k=laptop' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser')
Parse the Data: Extract relevant data from the parsed HTML.
products = [] for item in soup.select('.s-main-slot .s-result-item'): name = item.select_one('.a-section > h2').text.strip() price = item.select_one('[data-component="corePrice"]').text.strip() products.append({'name': name, 'price': price})
Handling Amazon’s Anti-Scraping Measures
Amazon implements various measures to prevent scraping:
- CAPTCHAs: Use services like 2Captcha or Anti-Captcha to solve CAPTCHAs programmatically.
- IP Blocking: Rotate your IP address using proxies to avoid getting blocked.
- Bot Detection: Mimic human behavior by introducing random delays between requests and using headless browsers like Selenium.
Advanced Techniques for Robust Scraping
Rotating Proxies
Using rotating proxies can help you avoid IP blocking:
import requests
from requests.adapters import HTTPAdapter
proxies = {
'http': 'http://your-proxy',
'https': 'http://your-proxy'
}
session = requests.Session()
session.proxies.update(proxies)
Headless Browsers
Selenium allows you to control a browser through Python scripts:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
options = webdriver.ChromeOptions()
options.add_argument('--headless')
options.add_argument('--disable-gpu')
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()), options=options)
driver.get("https://www.amazon.com")
# Perform your scraping tasks here
Conclusion and Best Practices
Building a robust Amazon scraper requires careful consideration of tools, techniques, and ethical practices. Always ensure you comply with legal and ethical guidelines when scraping data from any website. Utilize GitHub repositories to leverage existing solutions and optimize your development process.
FAQs
What are the legal implications of web scraping?
Web scraping can be legally complex, depending on the terms of service of the target website and local laws. Always review the website’s robots.txt file and terms of service to understand their policies regarding data scraping.
How can I handle CAPTCHAs when scraping Amazon?
You can use CAPTCHA-solving services like 2Captcha or Anti-Captcha to automate the process of solving CAPTCHAs. These services provide APIs that you can integrate into your web scraper.
Are there any ethical considerations for web scraping?
Yes, it’s important to consider ethical implications when web scraping. Ensure you are not overloading the server, respect the website’s terms of service, and do not misuse or sell personal data without consent.
How can I avoid getting blocked by Amazon while scraping?
To avoid getting blocked, use rotating proxies, introduce random delays between requests, and mimic human behavior using headless browsers like Selenium. Additionally, respect the website’s rate limits and terms of service.
Can I use web scraping to gather data for commercial purposes?
While web scraping can be used for commercial purposes, it’s crucial to ensure compliance with legal and ethical standards. Review the target website’s terms of service and consult with a legal expert if necessary.