· Charlotte Will · 5 min read
How to Automate Data Collection with Python Webscraping
Learn how to automate data collection efficiently using Python web scraping. This comprehensive guide covers setting up your environment, choosing the right tools like BeautifulSoup and Selenium, writing your first web scraper, handling common challenges, and best practices for ethical web scraping. Enhance your data extraction skills today with practical steps and code examples.
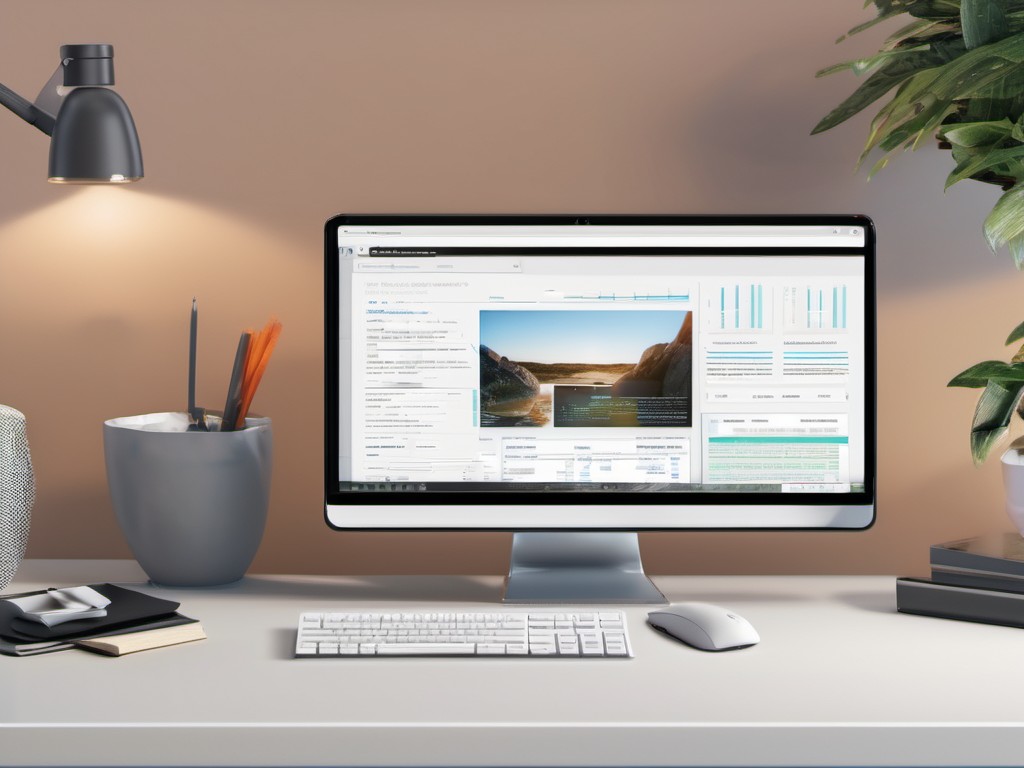
In today’s data-driven world, automating the collection of data is more crucial than ever. With Python web scraping, you can extract valuable information from websites efficiently and effectively. This comprehensive guide will walk you through the process of setting up your environment, choosing the right tools, writing your first web scraper, handling common challenges, and following best practices for ethical web scraping.
Introduction to Web Scraping with Python
Web scraping is a technique used to extract data from websites programmatically. By automating this process, you can gather large amounts of information without manually copying and pasting data. Python is an excellent language for web scraping due to its simplicity and powerful libraries such as BeautifulSoup and Selenium.
Setting Up Your Environment
Before diving into coding, it’s essential to set up your environment. You’ll need Python installed on your computer and a few libraries to get started.
Installing Necessary Libraries
Install Python: Ensure you have the latest version of Python installed on your machine. You can download it from python.org.
Create a Virtual Environment: It’s good practice to create a virtual environment for your project to manage dependencies efficiently.
python -m venv scraping_env source scraping_env/bin/activate # On Windows, use `scraping_env\Scripts\activate`
Install Libraries: Install the necessary libraries using pip.
pip install requests beautifulsoup4 selenium
Choosing the Right Tools
Selecting the right tools depends on the complexity of the website you’re scraping. The two most common tools are BeautifulSoup and Selenium.
BeautifulSoup vs. Selenium
- BeautifulSoup: Ideal for static websites where data is readily available in HTML tags. It parses HTML and XML documents, making it easy to extract data.
- Selenium: Better suited for dynamic websites that load content via JavaScript. Selenium automates web browsers, allowing you to interact with the website as a user would.
Writing Your First Web Scraper
Let’s start by writing a simple web scraper using BeautifulSoup to extract data from a static website.
Step-by-Step Guide
Import Libraries: Import the necessary libraries at the beginning of your script.
import requests from bs4 import BeautifulSoup
Send an HTTP Request: Use the
requests
library to send a GET request to the website.url = "https://example.com" response = requests.get(url)
Parse HTML Content: Create a BeautifulSoup object and parse the HTML content.
soup = BeautifulSoup(response.content, 'html.parser')
Extract Data: Locate the data you want to extract using selectors such as class names or tags.
titles = soup.find_all('h2', class_='title') for title in titles: print(title.get_text())
Save Data: You can save the extracted data to a file, such as a CSV or JSON.
import csv with open('titles.csv', 'w', newline='') as file: writer = csv.writer(file) writer.writerow(['Title']) # Write the header row for title in titles: writer.writerow([title.get_text()])
Handling Common Challenges
Web scraping can sometimes be tricky due to various challenges. Here’s how you can handle some common ones.
Dealing with CAPTCHAs
CAPTCHAs are designed to prevent automated access to websites. While there’s no foolproof way to bypass CAPTCHAs, here are a few strategies:
- Use Proxies: Rotate proxies to change your IP address frequently.
- Respect Robots.txt: Ensure you’re not violating the website’s terms of service.
- Consider Alternatives: Check if the data is available through APIs or paid services.
Best Practices for Ethical Web Scraping
Ethical web scraping involves respecting the website’s policies and minimizing the impact on its servers. Here are some best practices:
- Respect Robots.txt: Always check the
robots.txt
file of the website to understand which pages you can scrape. - Rate Limiting: Implement rate limiting to avoid sending too many requests in a short period, which can overload the server.
- Use Headers: Mimic a real browser by setting appropriate HTTP headers in your requests.
- Handle Exceptions Gracefully: Use try-except blocks to handle errors and prevent your scraper from crashing unexpectedly.
- Rotate Proxies: Rotate proxies to distribute the load across multiple IP addresses.
Conclusion
Web scraping with Python is a powerful skill that can significantly enhance your ability to collect data efficiently. By following this guide, you’ll be able to set up your environment, choose the right tools, write your first web scraper, handle common challenges, and follow best practices for ethical web scraping. As you become more proficient, explore advanced techniques such as using Selenium for dynamic content and handling CAPTCHAs effectively.
FAQs
1. Is Web Scraping Legal?
The legality of web scraping depends on the jurisdiction and the website’s terms of service. Generally, scraping public data is legal, but you should always respect the website’s robots.txt
file and terms of use.
2. How Do I Handle Dynamic Content?
For websites that load content via JavaScript, use Selenium or other browser automation tools to render the page and extract data after it has been fully loaded.
3. What is Rate Limiting?
Rate limiting involves controlling how frequently your scraper sends requests to a website. This helps prevent overloading the server and getting blocked.
4. Can I Use Web Scraping for Commercial Purposes?
While web scraping can be used for commercial purposes, it’s essential to ensure you comply with all relevant laws and the website’s terms of service. Consider consulting a legal expert if you’re unsure.
5. How Do I Handle Blocked IP Addresses?
If your IP address is blocked, consider using proxies or VPNs to change your IP address. Rotating proxies can help distribute the load and reduce the likelihood of getting blocked.