· Charlotte Will · 5 min read
How Does Python Webscraping Work in Real-World Scenarios
Discover how Python web scraping works in real-world scenarios with this comprehensive guide. Learn about popular libraries like Beautiful Soup and Scrapy, practical applications such as market research and competitor analysis, and best practices for effective and ethical web scraping.
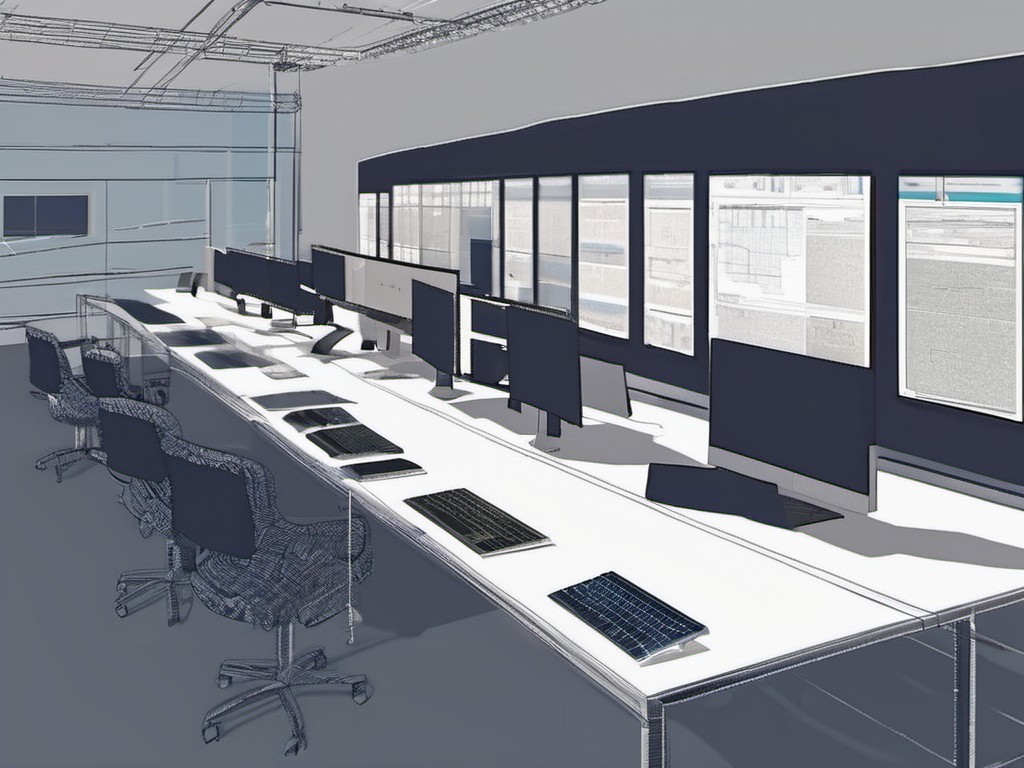
In today’s digital age, information is power—and web scraping has become an essential tool for extracting that power. Python, with its robust libraries and ease of use, stands out as a preferred language for web scraping tasks. But how does it all work in real-world scenarios? Let’s dive into the world of Python web scraping, exploring its basics, popular tools, and practical applications.
Understanding Web Scraping Basics
What is Web Scraping?
Web scraping is a technique used to extract data from websites programmatically. It involves automating the process of visiting web pages, collecting relevant information, and then storing it in a structured format like databases or spreadsheets. The data extracted can range from simple text to complex data structures such as tables and graphs.
Why Use Python for Web Scraping?
Python’s simplicity and extensive library support make it an ideal choice for web scraping:
- Ease of Learning: Python’s syntax is easy to learn, even for beginners.
- Rich Ecosystem: Libraries like Beautiful Soup, Scrapy, and Selenium simplify the scraping process.
- Versatility: Python can handle various tasks beyond web scraping, such as data analysis and visualization.
Popular Python Libraries for Web Scraping
Beautiful Soup Overview
Beautiful Soup is a popular library that parses HTML and XML documents. It creates parse trees from page source code that can be used to extract data easily.
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Example extraction
title = soup.find('h1').text
print(title)
Introduction to Scrapy
Scrapy is a powerful, open-source web crawling framework that simplifies the process of extracting data from websites. It’s particularly useful for large-scale projects due to its ability to handle concurrent requests and manage data pipelines efficiently.
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
title = response.css('h1::text').get()
yield {'title': title}
Real-World Applications of Python Web Scraping
Case Study 1: Market Research
Imagine you’re a marketer looking to understand the pricing strategies of your competitors. You can use web scraping to gather data from various e-commerce websites, extract product prices, and analyze trends.
import requests
from bs4 import BeautifulSoup
def get_price(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
price = soup.find('span', class_='price').text
return price
competitor_urls = ['https://example1.com/product', 'https://example2.com/product']
for url in competitor_urls:
print(f'Price on {url}: {get_price(url)}')
Case Study 2: Competitor Analysis
Web scraping can help you monitor your competitors’ websites for updates, new products, or changes in their services. This data can be crucial for staying ahead of the competition.
class CompetitorSpider(scrapy.Spider):
name = 'competitor'
start_urls = ['https://competitor1.com', 'https://competitor2.com']
def parse(self, response):
# Example extraction logic here
pass
Setting Up Your Web Scraper in Python
Prerequisites
Before diving into coding, ensure you have the necessary libraries installed:
pip install requests beautifulsoup4 scrapy selenium
Basic Workflow
- Identify Target Data: Determine what data you need to extract (e.g., product names, prices).
- Inspect the Website Structure: Use browser developer tools to understand the HTML structure.
- Write Scraping Code: Use libraries like Beautiful Soup or Scrapy to write your scraper.
- Handle Dynamic Content: For websites using JavaScript to load content, consider Selenium.
- Store and Analyze Data: Save extracted data into databases or spreadsheets for further analysis.
Best Practices for Web Scraping
Respect Robots.txt
Always check the robots.txt
file of a website to understand its scraping policies. This file specifies which parts of the site can be crawled and indexed.
https://example.com/robots.txt
Handle Rate Limiting
To avoid overloading servers, implement rate limiting in your scraper. Scrapy has built-in features for this:
import scrapy
from scrapy.utils import request_fingerprint
class MySpider(scrapy.Spider):
custom_settings = {
'DOWNLOAD_DELAY': 1,
'CONCURRENT_REQUESTS_PER_DOMAIN': 2,
}
Manage Proxies
Using proxies can help you bypass IP bans and distribute your requests across multiple IP addresses.
import scrapy
class ProxySpider(scrapy.Spider):
custom_settings = {
'PROXY_POOL_ENABLED': True,
'PROXY_POOL_PAGE_RETRY_TIMES': 5,
}
Advanced Web Scraping Techniques
Handling JavaScript with Selenium
For websites that rely heavily on JavaScript to load content, Selenium can be a lifesaver.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
# Example extraction
title = driver.find_element_by_tag_name('h1').text
print(title)
Data Extraction Techniques
- Regular Expressions: Use regex for pattern matching and data extraction.
- XPath Queries: Precisely locate elements within the HTML structure using XPath.
Legal Considerations in Web Scraping
Before you start scraping, it’s crucial to be aware of legal implications:
- Terms of Service: Ensure your actions comply with the website’s terms of service.
- Copyright Laws: Respect copyright and avoid scraping protected content without permission.
Conclusion
Python web scraping is a powerful tool that can revolutionize data collection and analysis in real-world scenarios. Whether you’re conducting market research, analyzing competitors, or extracting specific data sets, the right approach to web scraping can yield valuable insights. By adhering to best practices and considering legal implications, you can harness the full potential of web scraping while staying on the right side of the law.
FAQs
What are the legal implications of web scraping?
Web scraping must comply with website terms of service and copyright laws. Always check robots.txt
and consider obtaining permission for large-scale or sensitive data extraction.
How do I handle dynamic content while web scraping?
For handling dynamic content, you can use Selenium, which automates browser interactions to render JavaScript-heavy pages before extracting the required data.
Can I use Python for large-scale web scraping projects?
Yes, Python is highly suitable for large-scale web scraping with libraries like Scrapy, which can handle concurrent requests and manage data pipelines efficiently.
What are some ethical considerations in web scraping?
Ethical considerations include respecting privacy, avoiding overloading servers, complying with legal requirements, and being transparent about your intentions.
How can I store and analyze the data extracted through web scraping?
Extracted data can be stored in databases or spreadsheets for further analysis. Tools like pandas and NumPy are popular for data manipulation and analysis in Python.