· Charlotte Will · webscraping · 4 min read
Extracting Data from Web Forms Using Advanced Web Scraping Techniques
Master advanced web scraping techniques to extract data from web forms efficiently. Learn practical methods using Python and tools like Selenium, Scrapy, and machine learning for real-time data collection and analysis.
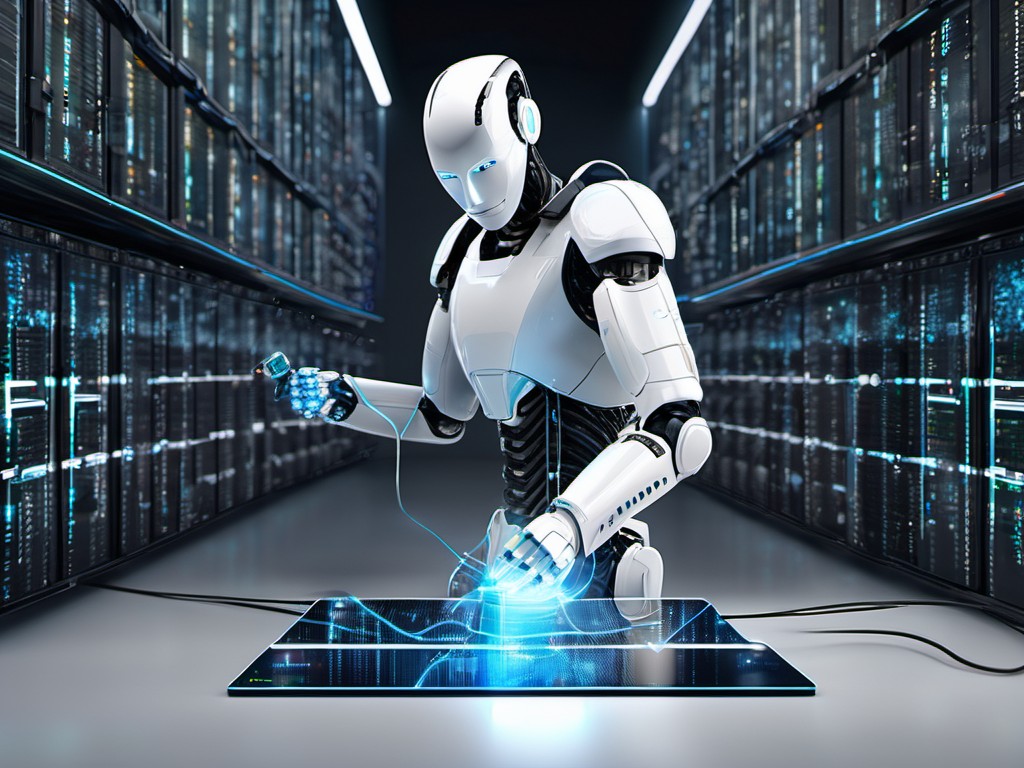
Web scraping is an essential tool for gathering data from web forms, automating repetitive tasks, and gaining insights into competitors or market trends. As businesses increasingly rely on digital platforms, the ability to extract data efficiently becomes paramount. In this guide, we’ll delve into advanced techniques for scraping web forms using Python and other tools.
Understanding Web Scraping
Web scraping involves collecting data from websites by sending requests and parsing HTML content. This process can be as simple or complex as needed, depending on the structure of the target website. For web forms specifically, extracting data often requires dealing with dynamic content generated via JavaScript.
Why Use Advanced Web Scraping Techniques?
Basic scraping methods may fall short when dealing with modern, interactive websites. Advanced techniques enable you to handle:
- Dynamic content loaded with JavaScript.
- Form submissions and authentication.
- Real-time data extraction.
- Machine learning integration for analyzing collected data.
Preparation: Tools and Libraries
Before diving into the techniques, let’s outline the essential tools for successful web scraping:
Python Libraries
- BeautifulSoup: Great for parsing HTML and XML documents.
- Requests: Handles HTTP requests effortlessly.
- Selenium: Automates browser actions, useful for JavaScript-heavy sites.
- Scrapy: A powerful web scraping framework.
- Pandas: Excellent for data manipulation and analysis.
Web Scraping Tools
- Scrapy: Python-based open-source scraping tool.
- Octoparse: User-friendly, no-code solution for web scraping.
- ParseHub: Supports JavaScript rendering without manual setup.
Advanced Techniques for Extracting Data from Web Forms
Handling Dynamic Content with Selenium
Sites often load form data dynamically using JavaScript. Selenium can mimic user interactions to render and scrape this content:
from selenium import webdriver
from bs4 import BeautifulSoup
import time
# Set up the WebDriver (assuming Chrome)
driver = webdriver.Chrome()
# Navigate to the target form page
driver.get('http://example.com/form')
# Wait for dynamic content to load
time.sleep(5)
# Parse the rendered HTML
soup = BeautifulSoup(driver.page_source, 'html.parser')
# Extract data from the form fields
form_data = soup.find_all('input', {'type': 'text'})
for field in form_data:
print(field['value'])
Automating Form Submissions
Automating form submissions can be crucial for scraping data that requires user input:
from selenium.webdriver.common.by import By
# Locate the input fields and submit button
username_field = driver.find_element(By.NAME, 'username')
password_field = driver.find_element(By.NAME, 'password')
submit_button = driver.find_element(By.NAME, 'submit')
# Fill in the form fields
username_field.send_keys('your_username')
password_field.send_keys('your_password')
# Submit the form
submit_button.click()
Real-Time Data Scraping with Scrapy
Real-time data extraction can be achieved using Scrapy’s ability to handle multiple requests concurrently:
import scrapy
from scrapy.crawler import CrawlerProcess
class FormSpider(scrapy.Spider):
name = 'form_spider'
start_urls = ['http://example.com/form']
def parse(self, response):
# Extract data from the form fields
form_data = response.css('input[type="text"]::attr(value)').getall()
for data in form_data:
yield {'field_data': data}
process = CrawlerProcess({
'USER_AGENT': 'Mozilla/5.0'
})
process.crawl(FormSpider)
process.start()
Integrating Machine Learning for Data Analysis
After extracting the data, you can use machine learning to analyze it:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Assuming extracted_data is a list of dictionaries
df = pd.DataFrame(extracted_data)
# Preprocess data (e.g., handling missing values, encoding categoricals)
X = df.drop('target', axis=1)
y = df['target']
# Split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a machine learning model
model = RandomForestClassifier()
model.fit(X_train, y_train)
Common Challenges and Solutions
Handling CAPTCHAs
CAPTCHAs can be challenging to bypass. Consider using CAPTCHA solving services or leveraging machine learning models trained to solve CAPTCHAs.
Respecting Robots.txt
Always check the robots.txt
file of a website before scraping. Respect the rules set by the site owner to avoid legal issues and potential bans.
Conclusion
Advanced web scraping techniques enable you to extract valuable data from web forms efficiently. By leveraging tools like Selenium, Scrapy, and integrating machine learning, you can automate data collection and gain insights that drive business decisions. Always remember to respect website policies and legal boundaries while scraping.
FAQs
What is the best tool for web scraping dynamic content? Selenium is often recommended for handling dynamic content as it can interact with JavaScript-rendered pages.
How do I automate form submissions using Python? You can use Selenium to locate and fill form fields, then submit the form programmatically.
Can Scrapy handle real-time data extraction? Yes, Scrapy supports concurrent request handling, making it suitable for real-time data scraping tasks.
How can I integrate machine learning with web scraped data? After extracting the data, you can preprocess it using libraries like Pandas and train machine learning models using libraries such as Scikit-learn.
What should I do if a website has CAPTCHAs? Consider using CAPTCHA solving services or leveraging machine learning models trained to solve CAPTCHAs, while also respecting the site’s terms of service.