· Charlotte Will · webscraping · 6 min read
Extracting Data from Infinite Scrolling Websites
Discover practical techniques for extracting data from infinite scroll websites using tools like Python, Selenium, and BeautifulSoup. Learn how to handle dynamic content, JavaScript execution, and anti-scraping measures with actionable advice and real-world examples.
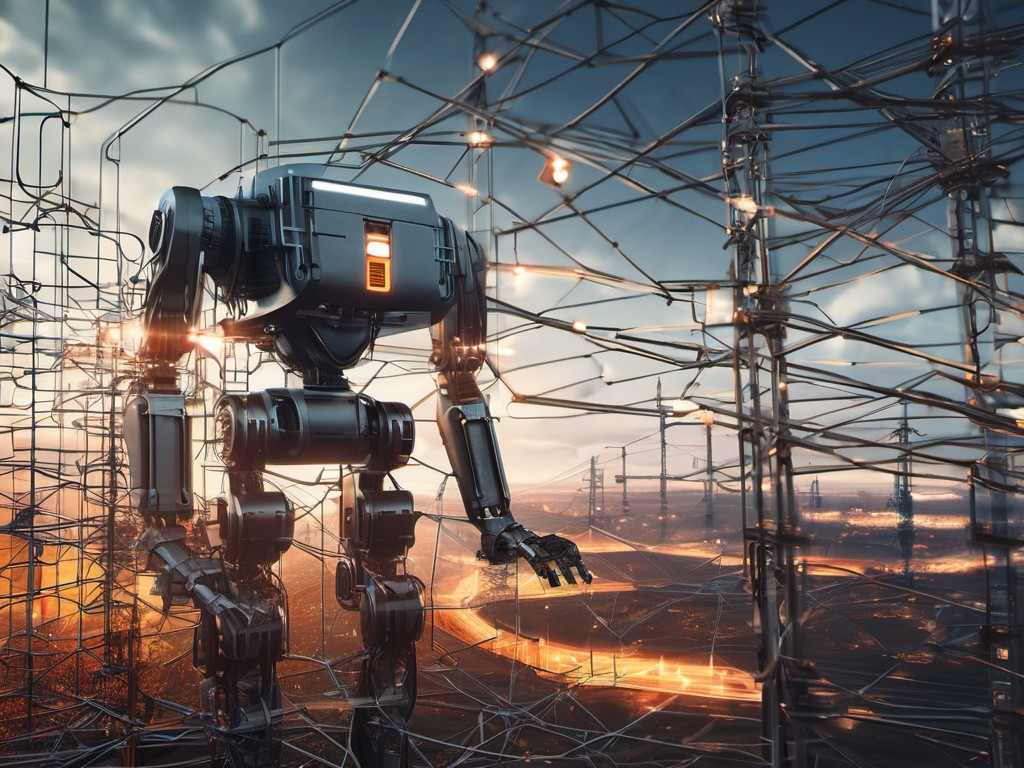
Introduction
In today’s digital age, websites employ various techniques to enhance user experience. One such technique is infinite scrolling, where content continuously loads as the user scrolls down the page. While this feature improves the browsing experience for users, it presents unique challenges when it comes to data extraction or web scraping. In this comprehensive guide, we will explore the intricacies of extracting data from infinite scroll websites, providing practical and actionable advice that caters to both beginners and intermediate web scrapers.
Understanding Infinite Scroll
How Infinite Scroll Works
Infinite scroll works by loading additional content dynamically as the user reaches the bottom of the page. This mechanism uses JavaScript to fetch more data from the server without requiring a full page reload. The primary goal is to keep users engaged and reduce the need for manual navigation, which enhances the overall browsing experience.
Common Use Cases for Infinite Scroll
Infinite scroll is commonly used in:
- Social media platforms (e.g., Twitter, Instagram)
- E-commerce websites (e.g., Amazon, eBay)
- News portals (e.g., CNN, BBC)
- Blogs and content aggregators (e.g., Medium, Reddit)
Challenges in Web Scraping Infinite Scroll Websites
Dynamic Content Loading Issues
Unlike static pages that load all content at once, infinite scroll websites dynamically load content as the user interacts with the page. This dynamic loading poses a significant challenge for traditional web scrapers, which are designed to handle static content.
JavaScript Execution Requirements
Infinite scroll relies heavily on JavaScript to fetch and render new content. Conventional web scraping tools like BeautifulSoup may not be sufficient because they do not execute JavaScript. To handle this, scrapers need to use tools that can run JavaScript.
Anti-Scraping Measures and CAPTCHAs
Websites often implement anti-scraping measures such as CAPTCHAs to prevent automated bots from scraping their content. These measures add an extra layer of complexity for web scrapers, requiring additional steps to bypass or handle these obstacles.
Tools and Techniques for Effective Data Extraction
Overview of Python Libraries
When it comes to scraping infinite scroll websites, several Python libraries can be immensely helpful:
- BeautifulSoup: For parsing HTML content.
- Selenium: For automating browser interactions and executing JavaScript.
- Scrapy: For building robust web crawlers.
- Puppeteer (via Pyppeteer): Another headless browser automation tool, similar to Selenium.
Step-by-Step Guide to Setting Up a Scraper
Configuring Drivers (e.g., ChromeDriver)
To use Selenium effectively, you need to configure the appropriate web driver (e.g., ChromeDriver for Google Chrome). Ensure that the driver version matches your browser version to avoid compatibility issues.
from selenium import webdriver
# Initialize the ChromeDriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('https://example.com')
Handling Dynamic Content with Selenium
Selenium can interact with elements on a webpage, simulate user actions like scrolling, and wait for new content to load dynamically.
# Scroll down to the bottom of the page
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Wait for new content to load (using WebDriverWait)
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.CSS_SELECTOR, ".new-content")))
Practical Examples and Code Snippets
Example 1: Scraping an E-commerce Site with Infinite Scroll
Suppose you want to scrape product information from an e-commerce site that uses infinite scroll. Here’s a simplified example using Selenium and BeautifulSoup.
from selenium import webdriver
from bs4 import BeautifulSoup
import time
# Initialize the ChromeDriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('https://example-ecommerce.com/products')
while True:
# Scroll down to load more products
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(2) # Wait for new content to load
# Parse the HTML with BeautifulSoup
soup = BeautifulSoup(driver.page_source, 'html.parser')
products = soup.find_all('.product-item')
if not products:
break
for product in products:
print(product.find('.product-title').text)
Example 2: Extracting Social Media Posts from an Infinite Scroll Page
Extracting posts from social media platforms that use infinite scroll can be achieved similarly.
from selenium import webdriver
from bs4 import BeautifulSoup
import time
# Initialize the ChromeDriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('https://example-socialmedia.com/posts')
while True:
# Scroll down to load more posts
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(2) # Wait for new content to load
# Parse the HTML with BeautifulSoup
soup = BeautifulSoup(driver.page_source, 'html.parser')
posts = soup.find_all('.post-item')
if not posts:
break
for post in posts:
print(post.find('.post-text').text)
Best Practices for Web Scraping Infinite Scroll Websites
Ethical Considerations and Legal Implications
Always ensure that your scraping activities comply with the website’s terms of service and legal requirements. Respect user privacy and do not engage in malicious activities.
Respecting Website robots.txt Rules
Before scraping a website, check its robots.txt
file to understand which pages are allowed to be crawled and which should be avoided.
Implementing Rate Limiting and Delays
To avoid overloading the server or triggering anti-scraping measures, implement rate limiting and delays in your scraper. This can help mimic human browsing behavior.
import time
# Add a delay between requests
time.sleep(3) # Wait for 3 seconds before the next request
Troubleshooting Common Issues
Handling JavaScript Errors
JavaScript errors can often disrupt your scraping process. Ensure that you handle exceptions and retry failed actions to maintain robustness.
try:
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
except Exception as e:
print(f"An error occurred: {e}")
Dealing with CAPTCHAs and Bot Detection Mechanisms
CAPTCHAs can be a significant hurdle. Consider using third-party services that offer CAPTCHA-solving capabilities or implementing advanced techniques like machine learning to solve them programmatically.
Optimizing Scraper Performance
Optimize your scraper by minimizing resource usage, reducing the number of network requests, and leveraging efficient data storage solutions.
# Close the browser after completing the task
driver.quit()
Conclusion
Extracting data from infinite scroll websites requires a blend of technical skills and an understanding of web dynamics. By using tools like Selenium and BeautifulSoup, you can overcome the challenges posed by dynamic content loading and JavaScript execution. Always remember to scrape responsibly and ethically, respecting website rules and legal boundaries.
FAQs
What are some alternatives to Selenium for infinite scroll scraping?
Alternatives to Selenium include Pyppeteer (a Python port of Puppeteer) and Playwright. Each has its strengths and can be used depending on the specific requirements of your project.
How can I handle websites that block my IP after repeated scraping attempts?
To handle IP blocking, you can use proxy servers to rotate your IP address or implement delays and rate limiting to mimic human browsing behavior. Additionally, respecting website robots.txt rules can help prevent getting blocked.
Is it legal to scrape data from any website?
The legality of web scraping depends on the website’s terms of service and local laws. Always check the site’s robots.txt
file and terms of service before beginning a scraping project, and seek legal advice if unsure.
How can I ensure my scraper does not overload the server?
Implement rate limiting and delays between requests to prevent your scraper from overloading the server. This can help mimic human browsing behavior and reduce the load on the server.
What steps can I take to respect user privacy while web scraping?
To respect user privacy, avoid scraping personal data and ensure that you comply with relevant data protection regulations such as GDPR or CCPA. Always use data responsibly and securely.