· Charlotte Will · webscraping · 6 min read
Building Resilient Web Scrapers with Error Handling and Retries
Discover practical strategies for building resilient web scrapers with error handling and retries in Python. Learn how to handle common errors, implement retries, and use advanced techniques like the circuit breaker pattern.
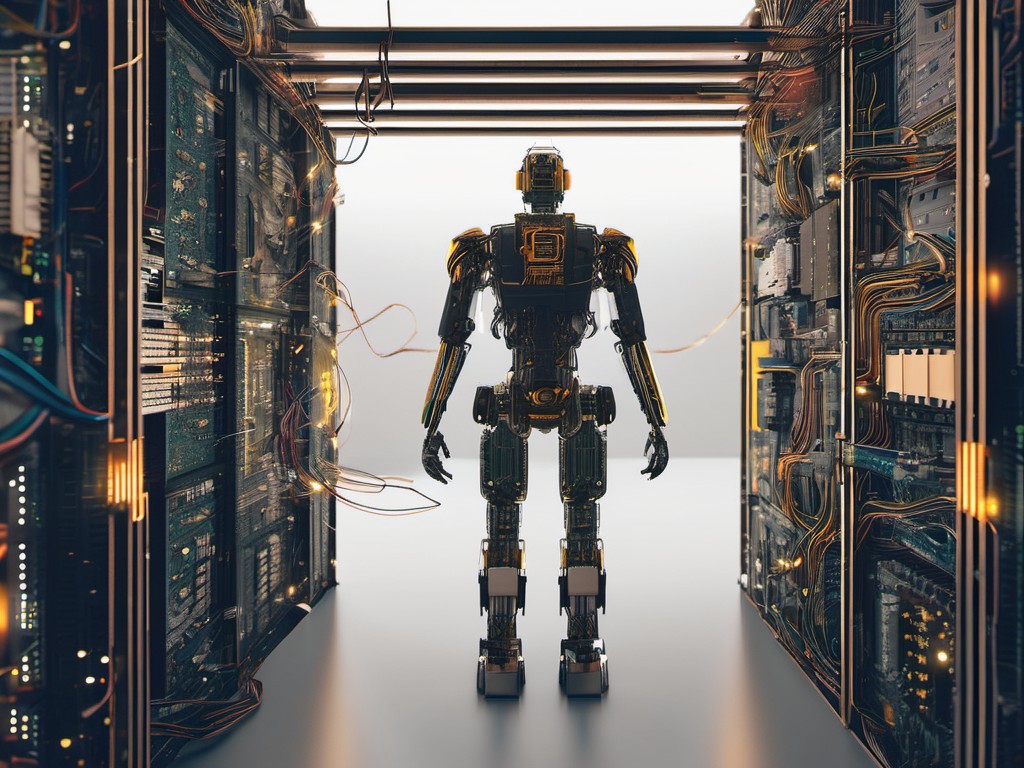
Web scraping is a powerful technique used to extract data from websites. However, it comes with its own set of challenges, particularly when dealing with dynamic content, rate limiting, or network issues. Building resilient web scrapers requires more than just writing parsing code; it involves handling errors gracefully and implementing retries to ensure your scraper can adapt to various scenarios. In this article, we will explore practical strategies for building robust web scrapers with error handling and retries in Python.
Introduction to Web Scraping
Web scraping involves automating the process of extracting information from websites. This technique is widely used for data collection, market research, and more. However, websites are dynamic and can change without notice, leading to errors during the scraping process. To handle these issues effectively, we need to build resilient web scrapers that can manage errors and retries efficiently.
Understanding Error Handling in Web Scraping
Error handling is crucial for maintaining the stability of your web scraper. Common errors include HTTP errors (e.g., 404, 503), network issues, timeouts, and parsing errors. Properly handling these errors ensures that your scraper can continue running smoothly even when faced with unexpected problems.
Types of Errors in Web Scraping
- HTTP Errors: These include status codes like 404 (Not Found), 503 (Service Unavailable), and others.
- Network Issues: Problems such as DNS resolution failures, timeouts, and connection resets.
- Parsing Errors: Issues that arise when the HTML structure changes unexpectedly.
- Rate Limiting: When a website limits the number of requests from a single IP address.
Python Error Handling for Web Scraping
Python offers several libraries and techniques to handle errors gracefully. The requests
library, for instance, provides easy-to-use methods for handling HTTP errors.
import requests
from requests.exceptions import RequestException
url = 'https://example.com'
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError for bad responses (4xx or 5xx)
except RequestException as e:
print(f"An error occurred: {e}")
Handling Exceptions in Python
Python’s try-except
blocks are essential for catching and handling exceptions. By using these blocks, you can ensure that your scraper continues running even if an error occurs.
import requests
from requests.exceptions import HTTPError
url = 'https://example.com'
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError for bad responses (4xx or 5xx)
except HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"An error occurred: {err}")
Implementing Retries in Web Scraping
Retries are a vital part of building resilient web scrapers. By implementing retries, you can ensure that your scraper makes multiple attempts to fetch data before giving up. This is especially useful when dealing with transient errors or rate limiting.
Using the tenacity
Library for Retries
The tenacity
library in Python simplifies the process of implementing retries. It provides a decorator that allows you to define retry strategies easily.
from requests import Session, HTTPError
import tenacity
session = Session()
@tenacity.retry(
wait=tenacity.wait_exponential(multiplier=1, min=4, max=10),
stop=tenacity.stop_after_attempt(3),
retry=tenacity.retry_if_exception_type((HTTPError,))
)
def fetch_url(url):
response = session.get(url)
return response
url = 'https://example.com'
response = fetch_url(url)
Building a resilient web scraper involves combining error handling and retries effectively. Here are some best practices to follow:
1. Graceful Degradation
Implement graceful degradation by logging errors rather than stopping the entire scraping process. This allows your scraper to continue fetching data from other sources even if an error occurs.
import requests
from requests.exceptions import HTTPError, Timeout
url = 'https://example.com'
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError for bad responses (4xx or 5xx)
except (HTTPError, Timeout) as e:
print(f"An error occurred: {e}")
# Continue with the next URL
2. Exponential Backoff
Exponential backoff is a retry strategy where you wait an increasing amount of time between each retry attempt. This helps reduce the load on the target server and increases the likelihood of successful requests.
import time
import requests
from requests.exceptions import HTTPError, Timeout
url = 'https://example.com'
retries = 3
backoff_factor = 0.1
for attempt in range(retries):
try:
response = requests.get(url)
response.raise_for_status()
break # Exit the loop if the request is successful
except (HTTPError, Timeout) as e:
print(f"Attempt {attempt+1} failed with error {e}")
time.sleep(backoff_factor * (2 ** attempt))
else:
print("Failed to fetch data after multiple attempts")
3. Circuit Breaker Pattern
The circuit breaker pattern helps prevent your scraper from making repeated failed requests, thus saving resources and reducing the load on the target server.
import time
from tenacity import retry, wait_exponential, stop_after_attempt
@retry(wait=wait_exponential(multiplier=1, min=4, max=10), stop=stop_after_attempt(3))
def fetch_url(session, url):
response = session.get(url)
return response
session = requests.Session()
url = 'https://example.com'
response = fetch_url(session, url)
Advanced Error Handling Techniques in Web Scraping
For more complex scenarios, consider implementing advanced error handling techniques such as:
- Rate Limiting: Use libraries like
ratelimiter
to manage the number of requests sent to a target server. - Captcha Solving: Implement CAPTCHA solving mechanisms to bypass rate limiting and automated detection systems.
- Proxy Rotation: Rotate proxies to distribute requests across multiple IP addresses, reducing the likelihood of being blocked.
Conclusion
Building resilient web scrapers requires a solid understanding of error handling and retries. By implementing these strategies, you can create robust scrapers that can adapt to various challenges encountered during data extraction. Whether you’re dealing with HTTP errors, network issues, or rate limiting, proper error handling and retries will ensure your scraper remains stable and effective.
FAQs
Why is error handling important in web scraping? Error handling is crucial for maintaining the stability of your web scraper. It ensures that your scraper can continue running smoothly even when faced with unexpected problems such as HTTP errors, network issues, or parsing errors.
What are common types of errors encountered in web scraping? Common errors include HTTP errors (e.g., 404, 503), network issues, timeouts, and parsing errors. Additionally, rate limiting is a common challenge where websites limit the number of requests from a single IP address.
How can retries be implemented in web scraping? Retries can be implemented using libraries like
tenacity
that provide decorators to define retry strategies easily. You can also manually implement retries with loops and exponential backoff techniques.What is the circuit breaker pattern, and how does it help in error handling? The circuit breaker pattern helps prevent your scraper from making repeated failed requests, thus saving resources and reducing the load on the target server. It ensures that your scraper doesn’t waste time trying to fetch data from a failing endpoint.
How can proxy rotation be used to enhance resilience in web scraping? Proxy rotation involves using multiple IP addresses for making requests, distributing them across different proxies. This helps reduce the likelihood of being blocked and increases the resilience of your web scraper against rate limiting and automated detection systems.