· Charlotte Will · webscraping · 5 min read
Building a Real-Time Price Monitoring System with Web Scraping
Discover how to build a real-time price monitoring system using web scraping techniques. Learn about tools, libraries, and best practices for efficient data extraction. Boost your ecommerce strategies with actionable advice on automating price tracking.
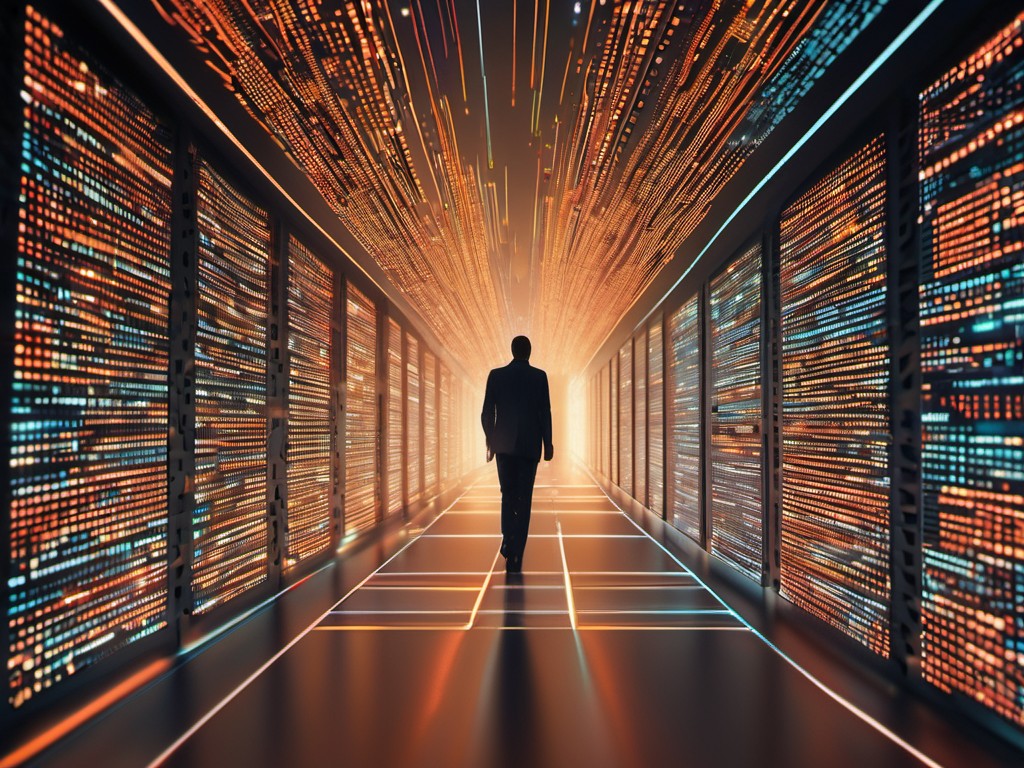
Real-time price monitoring is crucial in today’s dynamic ecommerce landscape. Whether you are an online retailer, a market research analyst, or just someone looking for the best deals, keeping track of prices can provide significant advantages. Web scraping offers an efficient way to automate this process and extract real-time pricing data from various websites. This article will guide you through building your own real-time price monitoring system using web scraping techniques.
What is Real-Time Price Monitoring?
Real-time price monitoring involves continuously tracking the prices of products across different ecommerce platforms in real-time. This helps businesses stay competitive, understand market trends, and adjust their pricing strategies accordingly. By automating this process with web scraping tools, you can collect and analyze data more efficiently than manually.
Why Use Web Scraping for Price Tracking?
Web scraping is a powerful technique that allows you to extract valuable data from websites automatically. When it comes to price tracking, web scraping offers several advantages:
- Automation: Automate the process of collecting pricing information, saving time and effort.
- Real-Time Data: Access up-to-date pricing data instantly.
- Competitive Analysis: Compare your prices with those of competitors to make informed decisions.
- Market Trends: Monitor market trends and identify opportunities for price adjustments.
- Efficiency: Scrape multiple websites simultaneously, gathering large amounts of data quickly.
Tools and Libraries for Web Scraping
Several tools and libraries can help you build a real-time price monitoring system:
1. Beautiful Soup
A Python library that makes it easy to scrape information from web pages by providing simple methods to navigate, search, and modify the parse tree.
2. Scrapy
An open-source web crawling framework for Python that can be used for a variety of applications such as data mining, information processing, and historical archival.
3. Selenium
A powerful tool for automating web browsers. It is especially useful for scraping websites that use JavaScript to load content dynamically.
4. Requests and lxml
Combining these two libraries allows you to make HTTP requests to the target website and parse the HTML content efficiently.
Building Your Real-Time Price Monitoring System
Let’s walk through the steps to build your real-time price monitoring system:
Step 1: Setting Up the Environment
First, ensure you have Python installed on your machine. Then, set up a virtual environment and install the necessary libraries:
pip install requests beautifulsoup4 lxml schedule
Step 2: Writing the Scraper Script
Create a Python script to perform web scraping. Here is a basic example using requests
and BeautifulSoup
:
import requests
from bs4 import BeautifulSoup
def get_price(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
price_element = soup.find('span', class_='price')
return price_element.text if price_element else "Price not found"
Step 3: Implementing Data Extraction Logic
Enhance the script to extract specific data points relevant to your needs, such as product names and prices.
def extract_data(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
products = []
for item in soup.find_all('div', class_='product'):
name = item.find('h2', class_='name').text
price = item.find('span', class_='price').text
products.append({'name': name, 'price': price})
return products
Step 4: Automating the Process with Schedulers
To make your script run at regular intervals, use a scheduling library like schedule
.
import schedule
import time
def job():
url = "https://example.com/products"
data = extract_data(url)
print("Data extracted:", data)
# Schedule the job to run every 5 minutes
schedule.every(5).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1)
Advanced Techniques for Enhanced Performance
1. Handling Dynamic Content
Use Selenium to handle websites that load content dynamically with JavaScript.
from selenium import webdriver
def get_price_with_selenium(url):
driver = webdriver.Chrome()
driver.get(url)
price_element = driver.find_element_by_class_name('price')
return price_element.text
driver.quit()
2. Using WebSockets for Real-Time Updates
Integrate WebSocket connections to receive real-time updates from websites that support this feature.
3. Cloud Services for Scalability
Consider using cloud services like AWS Lambda and Google Cloud Functions for scalable and efficient web scraping operations.
For more details, refer to our article on Building a Real-Time Price Monitoring System with Web Scraping and Cloud Services.
Best Practices and Ethical Considerations
- Respect Robots.txt: Always check the target website’s
robots.txt
file to ensure you are not violating their scraping policies. - Rate Limiting: Avoid overwhelming servers by implementing rate limiting in your scripts.
- Data Storage: Use databases like MongoDB or PostgreSQL to store and analyze the extracted data efficiently.
- Error Handling: Implement robust error handling to manage network issues, website changes, and other potential problems.
- Legal Compliance: Ensure your scraping activities comply with legal regulations and the terms of service of the websites you are targeting.
For more advanced techniques, read our article on Advanced Techniques for Real-Time Web Scraping.
Conclusion
Building a real-time price monitoring system with web scraping can significantly enhance your ecommerce strategies. By leveraging automation tools and Python libraries, you can efficiently gather and analyze pricing data to stay competitive in the market. Always remember to follow best practices and ethical guidelines to ensure your scraping activities are legal and responsible.
FAQ Section
1. What is web scraping?
Web scraping is a technique used to extract data from websites programmatically. It involves sending HTTP requests to the target website, parsing the HTML content, and extracting the desired information.
2. Is web scraping legal?
The legality of web scraping depends on the terms of service of the targeted website and local laws. Always ensure you are respecting the website’s robots.txt
file and not violating any legal regulations.
3. How can I handle websites that use JavaScript to load content?
To handle websites with dynamic content loaded via JavaScript, use tools like Selenium or Puppeteer, which can render JavaScript before extracting data.
4. What are some common issues encountered in web scraping?
Common issues include changes in the website’s HTML structure (which can break your scraper), rate limiting by the server, and legal complications if you violate the website’s terms of service or local laws.
5. Can I use cloud services for web scraping?
Yes, using cloud services like AWS Lambda and Google Cloud Functions can make your web scraping operations more scalable and efficient. These services allow you to run scraping tasks in parallel and handle large volumes of data effectively.