· Charlotte Will · webscraping · 5 min read
Building a Real-Time News Aggregator with Web Scraping and Natural Language Processing (NLP)
Learn how to build a real-time news aggregator using web scraping and Natural Language Processing (NLP). This comprehensive guide covers practical techniques, code examples, and tools for extracting, processing, and categorizing news data in Python. Optimize your news aggregator with NLP techniques such as text classification and sentiment analysis, ensuring accurate and timely updates.
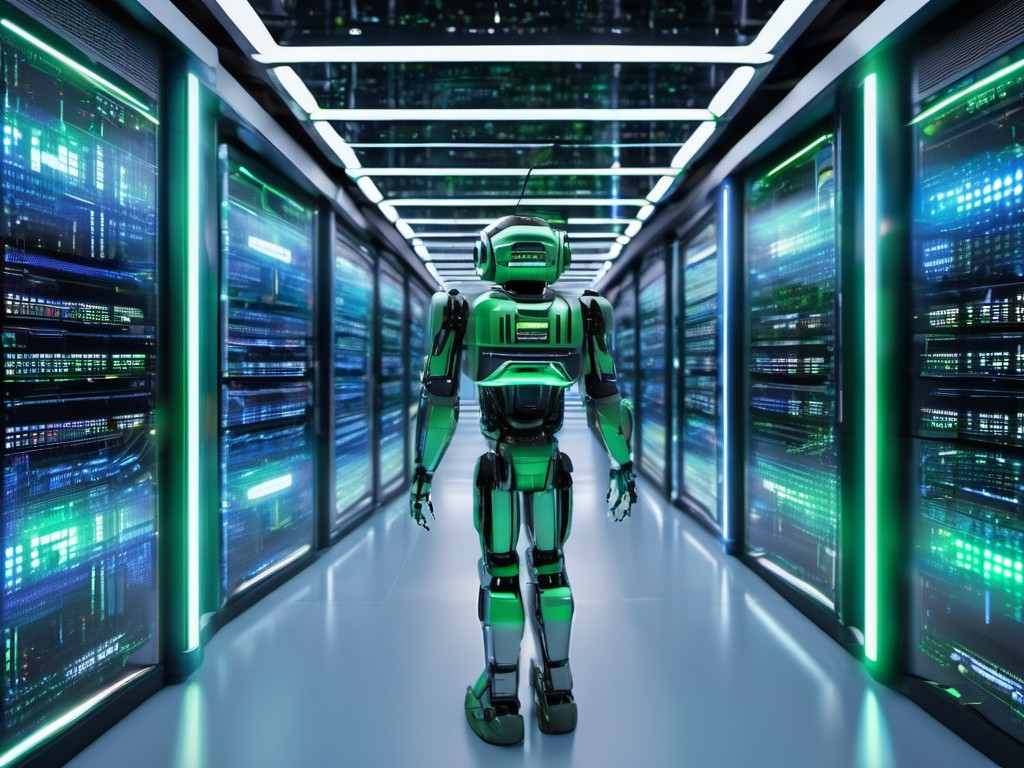
In today’s fast-paced world, staying up-to-date with the latest news is more crucial than ever. However, sifting through numerous websites to gather information can be time-consuming and inefficient. This is where a real-time news aggregator comes into play. By leveraging web scraping and Natural Language Processing (NLP), developers can create powerful tools that collect and categorize news data in real-time.
What is a Real-Time News Aggregator?
A real-time news aggregator is a software application or service designed to compile news articles from various sources on the web. These aggregators automatically gather, process, and display the latest news updates, making it easier for users to access information without having to visit multiple websites.
Key Components of a Real-Time News Aggregator
Building a real-time news aggregator involves several key components:
- Web Scraping: Extracting data from news websites.
- Data Processing: Organizing and cleaning the extracted data.
- Natural Language Processing (NLP): Analyzing and categorizing the news articles.
- Real-Time Updates: Ensuring that the aggregator displays the latest news.
- User Interface: Providing a user-friendly interface to view the compiled news.
Web Scraping: The Foundation of Data Extraction
Web scraping is the process of extracting data from websites. For a real-time news aggregator, this means fetching the latest articles and their associated metadata such as titles, publication dates, authors, and content.
Tools for Web Scraping
Several tools and libraries can help with web scraping in Python:
- BeautifulSoup: A powerful library for parsing HTML and XML documents.
- Scrapy: An open-source framework for building web scrapers.
- requests: A simple HTTP library for making requests to web pages.
Example of Web Scraping with BeautifulSoup
import requests
from bs4 import BeautifulSoup
# URL of the news website
url = "https://example-news-site.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extracting headlines
headlines = soup.find_all('h2', class_='headline')
for headline in headlines:
print(headline.text)
Natural Language Processing (NLP): Classifying and Categorizing News
NLP is essential for organizing the scraped data into meaningful categories. By analyzing the content, NLP can classify articles based on topics such as politics, technology, sports, etc.
Key Techniques in NLP
- Text Preprocessing: Cleaning and normalizing text data.
- Tokenization: Breaking down text into smaller units like words or sentences.
- Named Entity Recognition (NER): Identifying entities such as people, organizations, and locations.
- Topic Modeling: Categorizing texts based on their topics.
- Sentiment Analysis: Determining the emotional tone behind words to infer attitudes and feelings.
Libraries for NLP in Python
- NLTK (Natural Language Toolkit): A comprehensive library for building Python programs to work with human language data.
- Spacy: An open-source software library for advanced NLP in Python.
- Gensim: A robust library for topic modeling and document similarity analysis.
Example of Text Classification with NLTK
import nltk
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.naive_bayes import MultinomialNB
# Sample news articles
articles = [
"The new iPhone was released today.",
"The government announced a new policy.",
"The local football team won the championship."
]
labels = ["technology", "politics", "sports"]
# Text preprocessing and vectorization
vectorizer = CountVectorizer(stop_words='english')
X = vectorizer.fit_transform(articles)
y = labels
# Training the classifier
clf = MultinomialNB()
clf.fit(X, y)
# Predicting the category of a new article
new_article = "The company announced a new product."
X_new = vectorizer.transform([new_article])
prediction = clf.predict(X_new)
print("Predicted category:", prediction[0])
Integrating Web Scraping and NLP for Real-Time Updates
To achieve real-time updates, you need a system that continuously scrapes data from news websites and processes it with NLP. This can be accomplished using a combination of web scrapers, schedulers, and event-driven architectures.
Tools for Real-Time Data Processing
- Celery: A distributed task queue for handling real-time tasks.
- Redis: An in-memory data structure store used as a database, cache, and message broker.
- WebSockets: For pushing real-time updates to the user interface.
Example: Real-Time Scraping with Schedulers
import sched, time
from scraper import scrape_news # Assuming you have a scraper function
from nlp_processor import process_text # Assuming you have an NLP processing function
s = sched.scheduler(time.time, time.sleep)
def job():
news_data = scrape_news()
processed_data = process_text(news_data)
save_to_database(processed_data) # Assuming you have a function to save data
print("Data processing complete.")
s.enter(60, 1, job) # Schedule the next scraping job in 60 seconds
s.enter(60, 1, job)
s.run()
Building the User Interface
Creating a user-friendly interface is crucial for the success of your real-time news aggregator. You can use web frameworks like Flask or Django to build the frontend and serve the processed data to users.
Frontend Technologies
- React/Angular/Vue: For building dynamic user interfaces.
- WebSocket libraries: Like Socket.IO for real-time communication between the server and client.
Example: Real-Time Updates with WebSockets in Flask
from flask import Flask, render_template
from flask_socketio import SocketIO, send
import threading
app = Flask(__name__)
app.config['SECRET_KEY'] = 'secret!'
socketio = SocketIO(app)
def background_thread():
while True:
news_data = scrape_news()
processed_data = process_text(news_data)
send(processed_data, broadcast=True)
time.sleep(60) # Update every minute
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
socketio.start_background_task(target=background_thread)
socketio.run(app, debug=True)
Conclusion
Building a real-time news aggregator involves combining web scraping and NLP techniques to extract, process, and categorize news data in real time. By leveraging powerful Python libraries and frameworks, developers can create robust applications that keep users informed with the latest news updates.
FAQs
What are some common challenges when building a real-time news aggregator?
- Handling dynamic websites that frequently change their structure.
- Dealing with large volumes of data and ensuring timely processing.
- Avoiding legal issues by respecting website terms of service and robots.txt files.
How can I ensure the accuracy of NLP-based text classification?
- Use a well-annotated dataset for training your models.
- Continuously refine and update your models with new data.
- Employ ensemble methods to combine multiple classifiers for better performance.
Can web scraping be done without writing any code?
- Yes, there are visual scraping tools like ParseHub or Octoparse that allow you to create scrapers using a point-and-click interface. However, they may not offer the same level of customization and flexibility as coding your own scraper.
How can I handle rate limits when web scraping?
- Introduce random delays between requests to mimic human browsing behavior.
- Use proxy servers to rotate IP addresses.
- Implement retry logic with exponential backoff for failed requests.
What are some alternatives to web scraping for collecting news data?
- Utilize APIs provided by news websites, such as the News API or GDELT Project.
- Scrape RSS feeds, which are designed to be machine-readable and easier to parse than HTML.