· Charlotte Will · webscraping · 5 min read
Building a Distributed Web Scraping System with Apache Kafka
Discover how to build a scalable distributed web scraping system using Apache Kafka for real-time data processing and handling large-scale projects efficiently. Learn practical steps, architecture overview, and tips for achieving high performance and reliability in your web scraping operations.
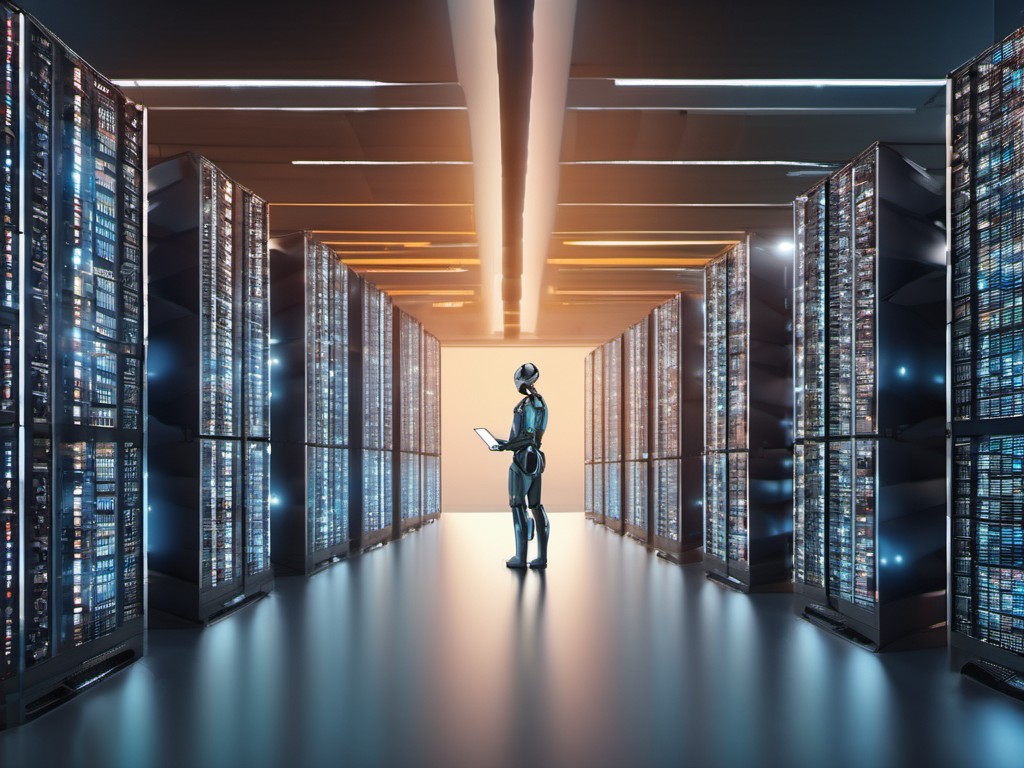
Web scraping has become an essential tool for businesses to gather data from websites efficiently. However, as the scale of web scraping operations increases, so does the complexity of managing them. Building a distributed web scraping system can help you handle large-scale web scraping projects more effectively. In this article, we’ll dive into how you can build a scalable web scraping architecture using Apache Kafka for real-time data processing.
What is Web Scraping?
Web scraping involves extracting information from websites by automatically fetching and parsing the HTML content. This process allows you to gather data on products, prices, reviews, or any other relevant information that can be found on web pages.
Why Use a Distributed System for Web Scraping?
As your web scraping requirements grow, managing everything on a single machine becomes impractical. A distributed system enables you to:
- Scale horizontally: Add more machines to handle increased load without significantly impacting performance.
- Improve reliability: Distribute tasks across multiple nodes so that the failure of one node doesn’t bring down the entire system.
- Process data in real-time: Distribute the scraped data to different processing units for immediate analysis and action.
Introduction to Apache Kafka
Apache Kafka is an open-source platform designed for building real-time data pipelines and streaming applications. It excels at handling large volumes of data with high throughput and low latency, making it ideal for web scraping systems.
Key Features of Apache Kafka
- Distributed: Kafka clusters can be distributed across multiple nodes to ensure reliability and scalability.
- Fault-tolerant: Data is replicated across brokers, ensuring that no data is lost in case of a node failure.
- Real-time processing: Kafka allows for real-time data streaming and processing, making it perfect for applications requiring immediate action based on incoming data.
Architectural Overview
Here’s an outline of the architecture we’ll build:
- Scrapers: Multiple scraper instances will extract data from websites and send it to Kafka topics.
- Kafka Cluster: A cluster of Kafka brokers that receive, store, and distribute the scraped data.
- Consumers: Different consumer applications will process the data in real-time for various purposes (e.g., storing in a database, triggering alerts).
Step-by-Step Implementation
1. Setting Up Kafka Cluster
First, you’ll need to set up a Kafka cluster. You can do this on your local machine for testing or use cloud services like AWS MSK or Confluent Cloud for production environments.
# Start Zookeeper (required by Kafka)
bin/zookeeper-server-start.sh config/zookeeper.properties
# Start Kafka server
bin/kafka-server-start.sh config/server.properties
2. Creating Kafka Topics
Create topics in Kafka where your scrapers will send the data. For example:
bin/kafka-topics.sh --create --topic web_scrape_data --bootstrap-server localhost:9092 --partitions 3 --replication-factor 1
3. Writing Scrapers
Develop your scrapers using your preferred programming language (Python, Java, etc.). Ensure each scraper instance can send data to the Kafka topic.
from kafka import KafkaProducer
import requests
producer = KafkaProducer(bootstrap_servers='localhost:9092')
def fetch_data(url):
response = requests.get(url)
return response.text
def send_to_kafka(topic, data):
producer.send(topic, data.encode('utf-8'))
producer.flush()
url = 'http://example.com'
data = fetch_data(url)
send_to_kafka('web_scrape_data', data)
4. Creating Consumers
Create consumer applications that will process the incoming data. For example, you can have one consumer store the data in a database and another trigger alerts based on certain conditions.
from kafka import KafkaConsumer
import json
consumer = KafkaConsumer('web_scrape_data', bootstrap_servers='localhost:9092')
for message in consumer:
data = message.value.decode('utf-8')
# Process the data as needed
5. Scaling the System
To scale your system, you can:
- Add more scraper instances: Distribute the scraping load across multiple machines.
- Increase Kafka brokers: Add more nodes to your Kafka cluster to handle increased data throughput.
- Distribute consumers: Deploy consumer applications on different machines to process data in parallel.
Real-Time Data Processing with Kafka
Kafka’s strength lies in its ability to handle real-time data streams efficiently. By integrating Kafka with other tools like Apache Flink or Spark Streaming, you can perform complex real-time processing on your scraped data.
For more information on real-time data processing, refer to Building a Real-Time Price Monitoring System with Web Scraping and Cloud Services.
Building Distributed Web Scraping Systems with Apache Kafka for Scalability
When building a distributed web scraping system, scalability is paramount. By leveraging Apache Kafka, you can create a highly scalable architecture that handles large volumes of data efficiently.
For more details on achieving scalability, refer to Building Distributed Web Scraping Systems with Apache Kafka for Scalability.
Conclusion
Building a distributed web scraping system with Apache Kafka enables you to handle large-scale data extraction and real-time processing efficiently. By following the outlined steps, you can create a scalable and fault-tolerant architecture that meets your web scraping needs.
FAQs
What is the role of Zookeeper in a Kafka cluster?
- Zookeeper is used by Kafka to manage and coordinate the brokers in a cluster. It helps with leader election, configuration management, and other administrative tasks.
How do I ensure data reliability in my Kafka setup?
- Kafka provides data replication across multiple brokers. You can configure the
replication-factor
to determine how many copies of each message should be stored in the cluster.
- Kafka provides data replication across multiple brokers. You can configure the
Can I use Apache Kafka for small-scale web scraping projects?
- Yes, while Kafka excels at handling large volumes of data, it can also be used effectively in small-scale projects due to its flexibility and performance characteristics.
How do I monitor the health of my Kafka cluster?
- You can use tools like Confluent Control Center or Kafka Manager to monitor the health and performance of your Kafka cluster. These tools provide insights into broker status, topic metrics, and consumer group activities.
What are some best practices for securing a Kafka cluster?
- Best practices include using SSL/TLS for data encryption, configuring authentication mechanisms like SASL/PLAIN or SASL/SSL, and managing access control through ACLs (Access Control Lists).