· Charlotte Will · webscraping · 6 min read
Automating Web Scraping with Puppeteer and Node.js
Discover how to automate web scraping with Puppeteer and Node.js in this comprehensive guide. Learn practical techniques, best practices, and real-world examples to extract data efficiently from websites. Ideal for beginners and intermediate developers.
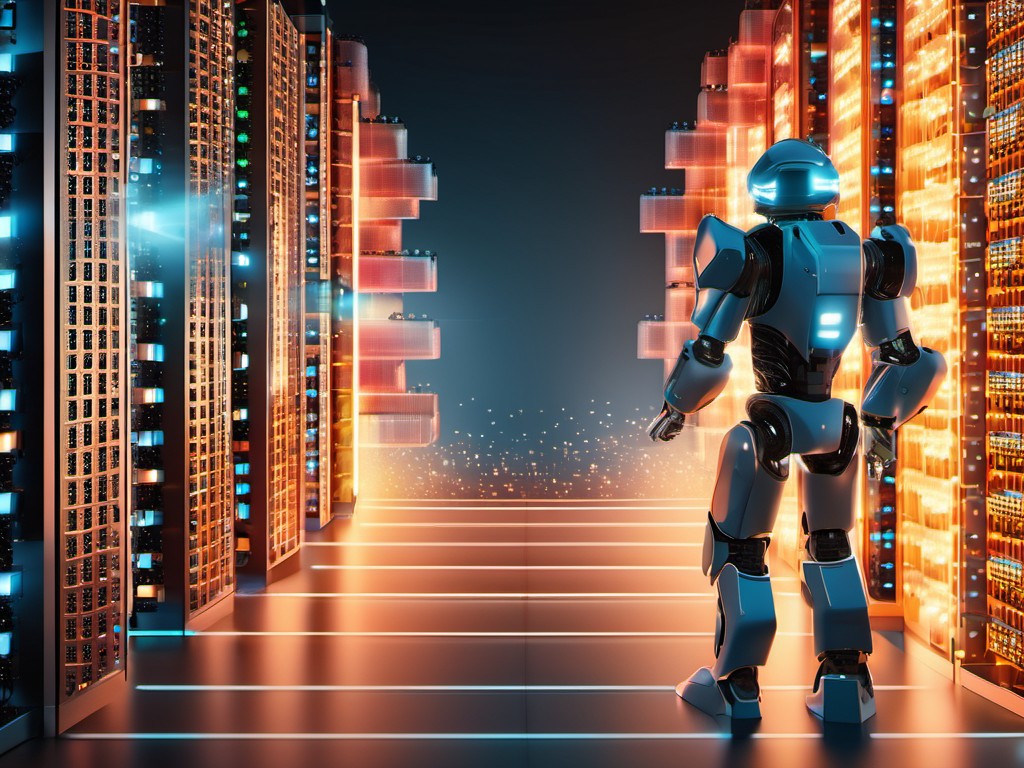
Web scraping has become an essential tool for extracting data from websites, enabling businesses to gather insights, monitor competitors, and automate workflows. One of the most powerful combinations for web scraping is using Puppeteer alongside Node.js. This article will guide you through the process of automating web scraping with Puppeteer and Node.js, providing practical advice and actionable steps to help both beginners and intermediate developers get started.
What is Web Scraping?
Web scraping involves extracting data from websites programmatically. This can be done for various purposes, such as gathering product information, monitoring prices, or collecting research data. Automating this process with tools like Puppeteer and Node.js allows you to efficiently scrape large amounts of data without manual intervention.
Why Use Puppeteer and Node.js?
Puppeteer
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium over the DevTools Protocol. It allows you to automate browser tasks, including web scraping, with ease. Some of its key features include:
- Headless Mode: Runs in a headless environment, making it suitable for server-side execution.
- Full Control Over Browser: Allows interaction with the DOM, network traffic, and more.
- Fast and Reliable: Built on top of Chrome’s rendering engine, ensuring fast and reliable web scraping.
Node.js
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It is known for its non-blocking, event-driven architecture, making it ideal for building scalable network applications. When combined with Puppeteer, Node.js provides a robust environment for automating web scraping tasks.
Setting Up Your Environment
Before diving into the coding part, ensure you have the necessary tools installed on your system:
- Node.js: Download and install Node.js from nodejs.org.
- npm (Node Package Manager): Comes bundled with Node.js.
- Puppeteer: Install Puppeteer using npm by running
npm install puppeteer
.
Basic Web Scraping Example
Let’s start with a basic example to scrape data from a website. We will create a simple Node.js script that uses Puppeteer to extract the title of a webpage.
Step 1: Create a New Project
Open your terminal and run the following commands to set up a new project:
mkdir puppeteer-web-scraping
cd puppeteer-web-scraping
npm init -y
Step 2: Install Puppeteer
Install Puppeteer by running:
npm install puppeteer
Step 3: Create the Script
Create a new file named scrape.js
and add the following code:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Extract the title of the webpage
const title = await page.title();
console.log(`Website Title: ${title}`);
await browser.close();
})();
Step 4: Run the Script
Run the script using Node.js by executing:
node scrape.js
You should see the title of the webpage printed to your console. This simple example demonstrates how easy it is to get started with Puppeteer and Node.js for web scraping.
Advanced Web Scraping Techniques
Now that we have covered the basics, let’s explore some advanced techniques to enhance our web scraping capabilities.
Handling Dynamic Content
Many modern websites load content dynamically using JavaScript. Puppeteer can handle this by waiting for specific elements to appear on the page before extracting data.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/dynamic-content');
// Wait for the dynamic content to load
await page.waitForSelector('#dynamic-content');
// Extract the dynamic content
const dynamicContent = await page.$eval('#dynamic-content', el => el.innerText);
console.log(`Dynamic Content: ${dynamicContent}`);
await browser.close();
})();
Navigating Through Pagination
Websites with paginated content require navigating through multiple pages to extract all the data. Puppeteer makes this easy by allowing you to programmatically click on “next” buttons or links.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
let currentPage = 1;
const maxPages = 3; // Set the maximum number of pages to scrape
while (currentPage <= maxPages) {
await page.goto(`https://example.com/products?page=${currentPage}`);
// Extract product data
const products = await page.$$eval('.product-item', items =>
items.map(item => ({
title: item.querySelector('.product-title').innerText,
price: item.querySelector('.product-price').innerText
}))
);
console.log(`Page ${currentPage} Products:`, products);
currentPage++;
}
await browser.close();
})();
Best Practices for Web Scraping
When automating web scraping, it’s essential to follow best practices to ensure your scripts are efficient, reliable, and ethical.
Respect Robots.txt
Always check the robots.txt
file of a website before scraping. This file contains directives that indicate which parts of the site can be crawled. Respect these rules to avoid legal issues.
Use Headless Mode
Run Puppeteer in headless mode to minimize resource usage and improve performance. You can enable headless mode by adding { headless: true }
to the puppeteer.launch()
function.
const browser = await puppeteer.launch({ headless: true });
Implement Rate Limiting
Avoid overwhelming a website’s server by implementing rate limiting in your scraping scripts. Puppeteer allows you to set a delay between requests using page.waitForTimeout()
.
await page.goto('https://example.com');
await page.waitForTimeout(2000); // Wait for 2 seconds before the next request
Handle Errors Gracefully
Web scraping scripts should handle errors gracefully to ensure they don’t crash unexpectedly. Use try-catch blocks to catch and log any errors that occur during execution.
Conclusion
Automating web scraping with Puppeteer and Node.js offers a powerful and flexible solution for extracting data from websites. By following the steps outlined in this article, you can create efficient and reliable web scraping scripts tailored to your specific needs. Whether you are a beginner or an intermediate developer, mastering these techniques will help you unlock valuable insights and automate workflows with ease.
FAQs
1. What is Puppeteer?
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium over the DevTools Protocol. It is widely used for web scraping, automated testing, and other browser automation tasks.
2. How do I install Puppeteer?
You can install Puppeteer using npm by running npm install puppeteer
in your terminal.
3. Can Puppeteer handle dynamic content?
Yes, Puppeteer can handle dynamic content by waiting for specific elements to appear on the page before extracting data. You can use methods like page.waitForSelector()
to achieve this.
4. What is headless mode in Puppeteer?
Headless mode allows Puppeteer to run in a headless environment, meaning it doesn’t open a visual browser window. This is ideal for server-side execution and minimizes resource usage. You can enable headless mode by adding { headless: true }
to the puppeteer.launch()
function.
5. How do I respect the robots.txt file of a website?
Before scraping a website, always check its robots.txt
file for directives that indicate which parts of the site can be crawled. Respect these rules to avoid legal issues and potential blocking by the website.