· Charlotte Will · Amazon API · 5 min read
Advanced Techniques for Real-Time Web Scraping with WebSockets
Discover advanced techniques for real-time web scraping using WebSockets, ensuring efficient and fast data extraction. Learn how to set up a WebSocket server, optimize connections, handle errors, and implement best practices for secure and effective web scraping applications.
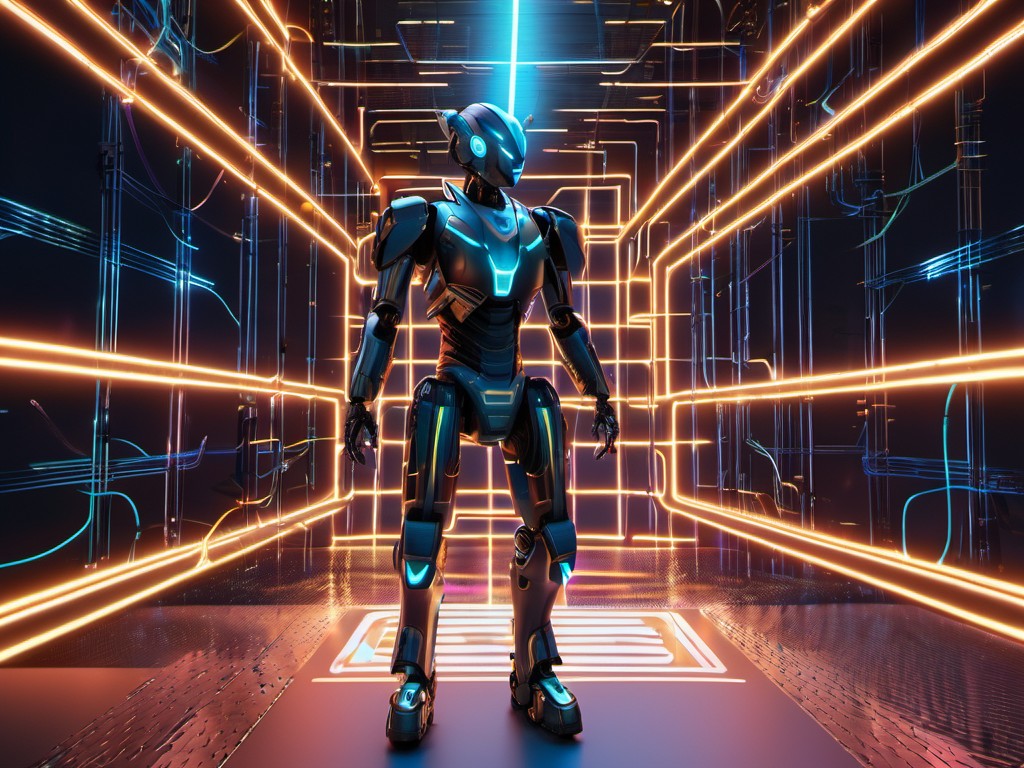
Real-time web scraping has become increasingly important in today’s data-driven world. Traditional web scraping methods often involve making repeated HTTP requests to a website, which can be slow and inefficient. Enter WebSockets: a protocol that provides full-duplex communication channels over a single TCP connection. In this article, we’ll explore advanced techniques for real-time web scraping using WebSockets.
What are WebSockets?
WebSockets are a modern alternative to the traditional HTTP protocol, designed to provide bidirectional communication between a client and server. Unlike HTTP, which uses a request-response model, WebSockets maintain an open connection, allowing both parties to send and receive data in real time. This makes them ideal for applications requiring live updates, such as chat applications, online gaming, and—you guessed it—real-time web scraping.
How WebSockets Work
- Handshake: The process begins with an HTTP handshake to establish a connection.
- Upgrade: Once the handshake is complete, the connection is upgraded to WebSocket protocol.
- Data Exchange: Both client and server can send data at any time without needing to reestablish a connection.
Why Use WebSockets for Real-Time Web Scraping?
Real-time web scraping with WebSockets offers several advantages over traditional methods:
- Efficiency: Reduces the overhead of establishing new connections repeatedly.
- Speed: Provides instant data updates, minimizing latency.
- Scalability: Handles multiple concurrent connections efficiently.
- Resource Management: More efficient use of network and server resources.
Implementing WebSockets for Real-Time Web Scraping
Setting Up a WebSocket Server
To set up a WebSocket server, you can use various libraries depending on your preferred programming language. For example:
- Python: Use the
websockets
library. - Node.js: Use the built-in
ws
module. - JavaScript: Use the browser’s built-in WebSocket API.
Example in Python
import asyncio
import websockets
async def echo(websocket, path):
async for message in websocket:
await websocket.send(message)
start_server = websockets.serve(echo, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
Connecting to a WebSocket Server
Once your server is up and running, you can connect to it using a client-side implementation. Here’s an example in JavaScript:
const socket = new WebSocket('ws://localhost:8765');
socket.onopen = function(e) {
console.log("[open] Connection established");
};
socket.onmessage = function(event) {
console.log(`[message] Data received from server: ${event.data}`);
};
socket.onclose = function(event) {
if (event.wasClean) {
console.log(`[close] Connection closed cleanly, code=${event.code} reason=${event.reason}`);
} else {
console.log('[close] Connection died');
}
};
socket.onerror = function(error) {
console.log(`[error] ${error.message}`);
};
Optimizing WebSocket Connections for Web Scraping
Handle Reconnection Logic
WebSocket connections can be unstable, so it’s crucial to implement reconnection logic. This involves detecting disconnections and automatically reestablishing the connection after a delay or on certain conditions.
Message Queueing
To handle scenarios where data might arrive while the client is offline, implement message queueing. Store incoming messages when the client is disconnected and send them once the connection is re-established.
Handling WebSocket Errors in Web Scraping
Errors can occur during WebSocket communication, such as network issues or server errors. Here are some strategies to handle these scenarios:
- Timeouts: Implement timeouts to close connections that remain idle for too long.
- Error Handling: Catch and log any errors that occur during data transmission.
- Retries: Implement retry logic with exponential backoff for transient errors.
- Graceful Degradation: Ensure your application can fall back to traditional HTTP requests if WebSockets are not available.
Advanced Web Scraping Techniques with WebSockets
Real-Time Data Synchronization
WebSockets allow you to synchronize data in real time, ensuring that the scraped data is always up-to-date. This can be particularly useful for monitoring changes on dynamic websites.
Combining Web Scraping and WebSockets
Integrate traditional web scraping techniques with WebSocket connections to achieve a hybrid approach. For example, use HTTP requests to extract static content and WebSockets for real-time updates.
Best Practices for Real-Time Web Scraping with WebSockets
- Security: Ensure your WebSocket connection is secure by using wss (WebSocket Secure) over HTTPS.
- Authentication: Implement proper authentication mechanisms to protect against unauthorized access.
- Rate Limiting: Respect the server’s rate limits to avoid getting blocked or banned.
- Data Validation: Validate incoming data to ensure its integrity and consistency.
- Logging: Maintain detailed logs of WebSocket connections, messages, and errors for debugging and monitoring purposes.
Conclusion
Real-time web scraping with WebSockets offers numerous advantages over traditional methods, including efficiency, speed, scalability, and resource management. By implementing advanced techniques such as handling reconnections, message queuing, error handling, and real-time data synchronization, you can create powerful web scraping applications that provide live updates.
FAQs
What is the difference between WebSockets and HTTP?
- WebSockets allow for full-duplex communication over a single TCP connection, whereas HTTP uses a request-response model with separate connections for each message exchange.
Can WebSockets be used with traditional web scraping tools?
- Yes, many modern web scraping tools support WebSockets. You can often configure them to use WebSocket endpoints alongside traditional HTTP requests.
How do I handle disconnections in a WebSocket-based scraper?
- Implement reconnection logic with message queueing to store and resend messages when the connection is reestablished.
Can WebSockets be used for scraping websites that don’t support them?
- No, WebSockets require server-side support. If a website doesn’t support WebSockets, you’ll need to rely on traditional HTTP requests or other real-time communication methods like Server-Sent Events (SSE).
What are some common issues with using WebSockets for web scraping?
- Common issues include network instability, server disconnections, message ordering problems, and security concerns. Proper error handling, reconnection logic, and security measures can help mitigate these issues.