· Charlotte Will · webscraping · 4 min read
Advanced Techniques for Python Web Scraping
Discover advanced techniques for Python web scraping, including handling dynamic content and real-time data extraction. Learn how to use BeautifulSoup, Requests, Scrapy, Selenium, and WebSockets to enhance your data collection capabilities. Optimize error handling and proxy management for scalable and reliable web scraping projects.
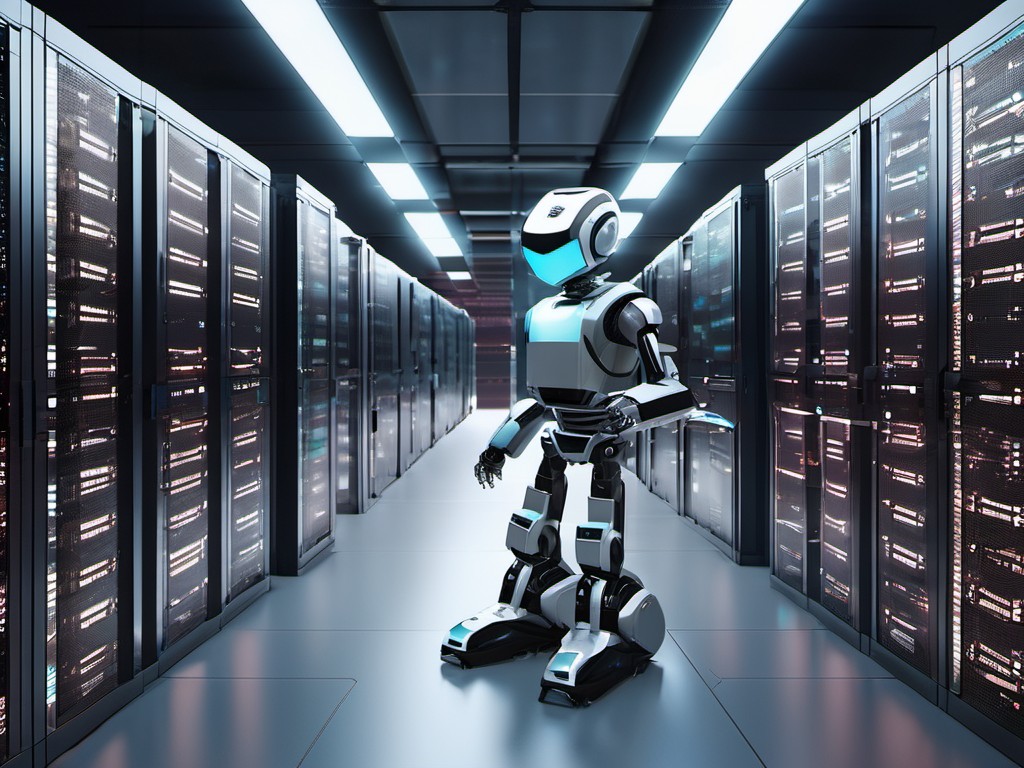
Introduction
Web scraping has become an essential tool in the arsenal of data analysts and developers alike. It allows us to extract valuable information from websites that would otherwise be challenging to obtain manually. Python, with its robust libraries and straightforward syntax, is one of the most popular languages for web scraping. In this article, we will delve into advanced techniques for Python web scraping, focusing on practical and actionable advice.
Understanding Python Web Scraping
What is Python Web Scraping?
Python web scraping involves using scripts to automatically extract data from websites. Unlike manually copying and pasting information, web scraping can handle large volumes of data efficiently. It’s particularly useful for tasks such as market research, price monitoring, lead generation, and more.
Advanced Libraries for Web Scraping
BeautifulSoup
BeautifulSoup is a popular library for parsing HTML and XML documents. It creates a parse tree from the page’s source code that can be used to extract data in a hierarchical and readable manner.
Example:
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# Extracting data
title = soup.title.string
print(title)
Requests Library
The requests
library simplifies making HTTP requests in Python. It’s often used in conjunction with BeautifulSoup to fetch the web page content before parsing it.
Example:
import requests
url = 'https://example.com'
response = requests.get(url)
print(response.text)
Scrapy
Scrapy is a powerful, open-source web scraping framework that allows you to extract data from websites and store it in various formats. It handles complex scenarios like pagination, concurrent downloads, and more.
Example:
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
title = response.css('title::text').get()
print(title)
Handling Dynamic Content
JavaScript Rendered Content
Modern web pages often use JavaScript to render content dynamically. Libraries like Selenium
can be used to handle such cases. Selenium allows you to automate browser interactions, making it possible to scrape JavaScript-rendered content.
Example:
from selenium import webdriver
from bs4 import BeautifulSoup
driver = webdriver.Chrome()
driver.get('https://example.com')
# Extracting data
soup = BeautifulSoup(driver.page_source, 'html.parser')
title = soup.title.string
print(title)
driver.quit()
AJAX Requests
Handling AJAX requests can be tricky since the data is loaded dynamically after the initial page load. Tools like Scrapy Splash
or Playwright
can help render JavaScript and capture AJAX requests effectively.
Example with Playwright:
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch(headless=False)
page = browser.new_page()
page.goto('https://example.com')
# Extracting data
title = page.title()
print(title)
browser.close()
Error Handling and Proxy Management
Advanced Error Handling Techniques
Robust error handling is crucial for reliable web scraping. This includes handling exceptions like HTTPError
, Timeout
, and TooManyRedirects
.
Example:
import requests
from requests.exceptions import HTTPError, Timeout, TooManyRedirects
try:
response = requests.get('https://example.com')
response.raise_for_status()
except HTTPError as http_err:
print(f'HTTP error occurred: {http_err}') # Python 3.6
except Timeout as timeout_err:
print(f'Timeout error occurred: {timeout_err}') # Python 3.6
except TooManyRedirects as redirect_err:
print(f'Too many redirects: {redirect_err}') # Python 3.6
Proxy Management for Large-Scale Scraping
When scraping at scale, using proxies can help avoid IP bans and rate limits. Libraries like Scrapy
have built-in support for rotating proxies.
Example with Scrapy:
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
custom_settings = {
'PROXY_POOL_ENABLE': True,
'PROXY_POOL_PAGE': 1,
}
def parse(self, response):
title = response.css('title::text').get()
print(title)
Real-Time Web Scraping
Real-time Web Scraping with WebSockets
For applications that require real-time data, WebSockets provide a reliable and efficient way to receive updates. Libraries like websockets
can be used to connect to WebSocket endpoints.
Example:
import asyncio
import websockets
async def connect():
uri = "wss://example.com/socket"
async with websockets.connect(uri) as websocket:
while True:
message = await websocket.recv()
print(message)
asyncio.get_event_loop().run_until_complete(connect())
Conclusion
Advanced Python web scraping techniques can greatly enhance your data extraction capabilities. By utilizing libraries like BeautifulSoup, Requests, and Scrapy, you can handle complex scenarios involving dynamic content and real-time updates. Additionally, robust error handling and proxy management are essential for reliable and scalable scraping operations.
FAQ Section
Q: What is the best library for Python web scraping? A: The choice of library depends on your specific needs. BeautifulSoup and Requests are great for simple tasks, while Scrapy excels in handling large-scale projects with complex requirements.
Q: How do I handle JavaScript-rendered content? A: You can use tools like Selenium or Playwright to render JavaScript and extract data from dynamically loaded content.
Q: What are some common errors in web scraping, and how can I handle them? A: Common errors include HTTP errors, timeouts, and too many redirects. You can handle these using try-except blocks and appropriate exceptions from the
requests
library.Q: How do proxies help in web scraping? A: Proxies help you rotate your IP address to avoid being banned or rate-limited by websites. They are particularly useful for large-scale scraping projects.
Q: Can I perform real-time web scraping with Python? A: Yes, you can use WebSockets to receive real-time updates from websites. The
websockets
library in Python provides a straightforward way to connect and handle WebSocket messages.