· Charlotte Will · webscraping · 5 min read
Advanced Strategies to Bypass CAPTCHAs in Web Scraping
Discover advanced strategies to bypass CAPTCHAs in web scraping, including using CAPTCHA solvers, headless browsers, OCR techniques, and proxy management. Enhance your data extraction capabilities with practical tips and code examples.
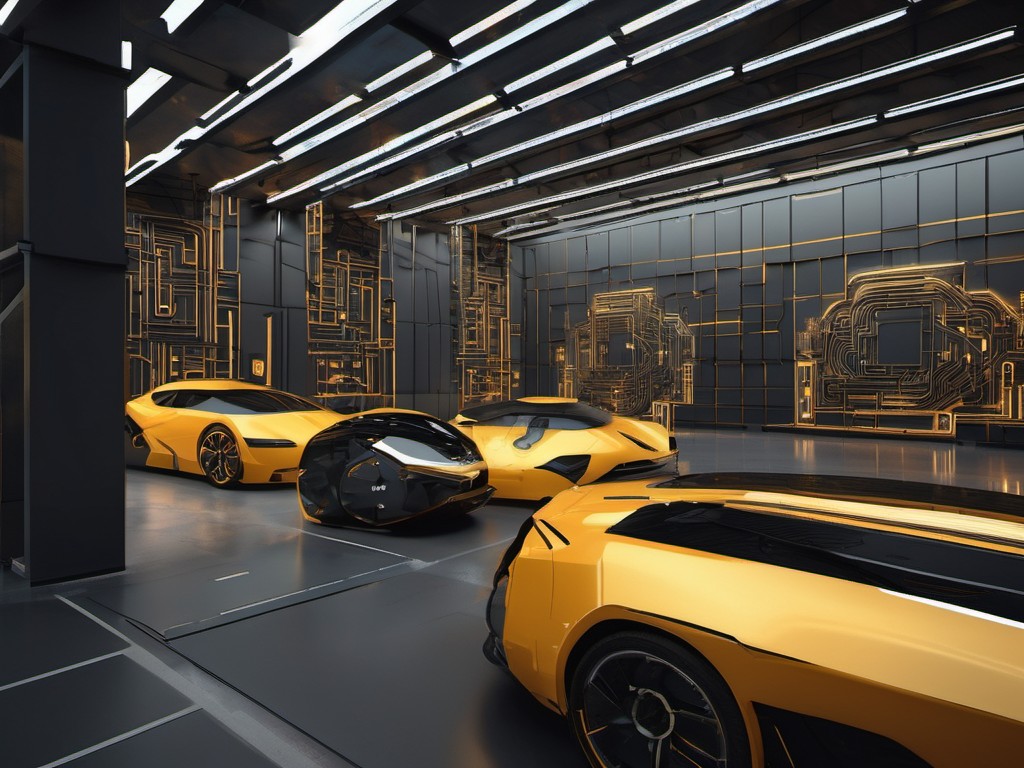
Web scraping has become an essential tool for extracting valuable data from websites, but it’s not without its challenges. One of the most significant hurdles is dealing with CAPTCHA (Completely Automated Public Turing test to tell Computers and Humans Apart) mechanisms. Designed to prevent automated bots from accessing a site, CAPTCHAs can thwart even the most sophisticated web scraping efforts. However, by employing advanced strategies, you can effectively bypass CAPTCHA protections and achieve your data extraction goals.
Understanding CAPTCHA Types
Before diving into the techniques for bypassing CAPTCHAs, it’s crucial to understand the different types of CAPTCHAs you might encounter:
- Text-based CAPTCHAs: These require users to enter a distorted text shown in an image.
- Image-based CAPTCHAs: Users must select images that match a specific criterion, such as all pictures containing cats.
- Audio CAPTCHAs: Users listen to an audio clip and type the words they hear.
- ReCAPTCHA v3: Google’s advanced CAPTCHA system that uses machine learning to analyze user behavior in real time.
Each type presents unique challenges, but with the right strategies, you can overcome them.
Advanced Techniques for Bypassing CAPTCHAs
1. Using CAPTCHA Solvers
CAPTCHA solver services and APIs can automatically solve CAPTCHAs on your behalf. Popular options include:
- 2Captcha
- Anti-Captcha
- Death By Captcha
These services typically use human solvers to decipher text-based CAPTCHAs and machine learning algorithms for image-based ones. Integrating these APIs into your web scraping pipeline can significantly reduce the hassle of dealing with CAPTCHAs directly.
2. Headless Browsers
Headless browsers simulate user interactions without a graphical interface, making them ideal for automated tasks like web scraping. Tools such as Puppeteer (for Chrome) and Playwright support headless mode and can handle JavaScript-heavy sites where traditional scrapers might fail.
To bypass CAPTCHAs with headless browsers:
- Set up the browser in headless mode.
- Use browser automation scripts to navigate through the site.
- Implement CAPTCHA solvers within the browser context if needed.
3. Optical Character Recognition (OCR)
Optical Character Recognition (OCR) technology can be employed to read and decipher text from images, making it a powerful tool for bypassing image-based CAPTCHAs. Libraries like Tesseract are widely used for OCR tasks in web scraping:
import pytesseract
from PIL import Image
# Load the image file
image = Image.open('captcha_image.png')
# Use Tesseract to do OCR on the image
text = pytesseract.image_to_string(image)
print(text)
4. Proxy Management Strategies
Rotating proxies can help you appear like a human user by changing your IP address frequently, reducing the likelihood of encountering CAPTCHAs:
- Residential Proxies: Use IP addresses assigned to real devices in residential areas.
- Datacenter Proxies: Cheaper but less reliable for avoiding detection.
- Proxy Rotation Services: Automate proxy rotation to minimize the risk of being flagged.
5. Machine Learning for CAPTCHA Solving
Machine learning algorithms can be trained to recognize and solve CAPTCHAs, especially image-based ones. Using frameworks like TensorFlow or PyTorch, you can develop custom models:
- Dataset Preparation: Collect a large dataset of CAPTCHA images and their corresponding solutions.
- Model Training: Train a convolutional neural network (CNN) to recognize patterns in the images.
- Inference: Use the trained model to solve new CAPTCHAs encountered during scraping.
6. Handling Anti-Bot Protections
Modern websites employ various anti-bot protections beyond just CAPTCHAs. These can include rate limiting, browser fingerprinting, and behavior analysis:
- Rate Limiting: Implement delays between requests to mimic human browsing patterns.
- Browser Fingerprinting: Use different browser configurations and headers to avoid detection.
- Behavior Analysis: Simulate realistic user interactions such as mouse movements and clicks.
Practical Examples and Code Snippets
Example 1: Using Puppeteer with CAPTCHA Solver API
const puppeteer = require('puppeteer');
const fetch = require('node-fetch');
async function solveCaptcha(captchaUrl) {
const response = await fetch(`https://2captcha.com/in.php?key=YOUR_API_KEY&method=post&file=${encodeURIComponent(captchaUrl)}`);
const data = await response.text();
return new Promise((resolve) => {
setTimeout(() => resolve(data), 1000);
});
}
async function scrape() {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('https://example.com');
// Assume the captcha image URL is extracted and stored in `captchaUrl`
const captchaSolution = await solveCaptcha(captchaUrl);
// Submit the CAPTCHA solution to bypass it
await page.type('#captcha-input', captchaSolution, { delay: 50 });
await page.click('#submit-button');
const data = await page.evaluate(() => {
// Extract the required data from the page
return document.querySelector('.data-class').innerText;
});
console.log(data);
await browser.close();
}
scrape().catch((err) => console.error(err));
Example 2: Using Tesseract for OCR
import pytesseract
from PIL import Image
# Load the image file
image = Image.open('captcha_image.png')
# Use Tesseract to do OCR on the image
text = pytesseract.image_to_string(image)
print("Captcha Solution:", text)
Conclusion
Bypassing CAPTCHAs in web scraping requires a combination of advanced techniques, tools, and strategies. From using CAPTCHA solver services to employing headless browsers and OCR, there are multiple approaches to overcome these obstacles. By integrating machine learning algorithms, managing proxies effectively, and handling anti-bot protections, you can enhance your web scraping capabilities significantly.
Remember to always comply with the target website’s terms of service and legal considerations when performing web scraping tasks.
FAQs
1. Can I use free CAPTCHA solver services? While free CAPTCHA solvers exist, they often come with limitations such as slower response times and reduced reliability compared to paid services.
2. How can I improve the accuracy of OCR for CAPTCHAs? Preprocessing images by enhancing contrast, reducing noise, or applying thresholding techniques can significantly improve OCR accuracy.
3. Are there any legal implications when bypassing CAPTCHAs? Bypassing CAPTCHAs may violate the terms of service of the target website and could potentially lead to legal consequences. Always ensure your actions are compliant with relevant laws and regulations.
4. How can I handle ReCAPTCHA v3 effectively? ReCAPTCHA v3 is more challenging to bypass due to its behavior analysis. Using a combination of proxies, rotating user agents, and simulating human-like interactions can help mitigate detection.
5. What are some best practices for proxy management in web scraping? Rotate proxies regularly, use residential IP addresses, and implement error handling to manage failed requests effectively. Additionally, consider using proxy management services for automated rotation and reliability.