· Charlotte Will · 6 min read
A Beginner's Guide to What is Python Webscraping and Its Applications
Learn how to get started with Python web scraping, explore essential libraries like BeautifulSoup and Requests, and discover practical examples and advanced topics in this comprehensive beginner's guide.
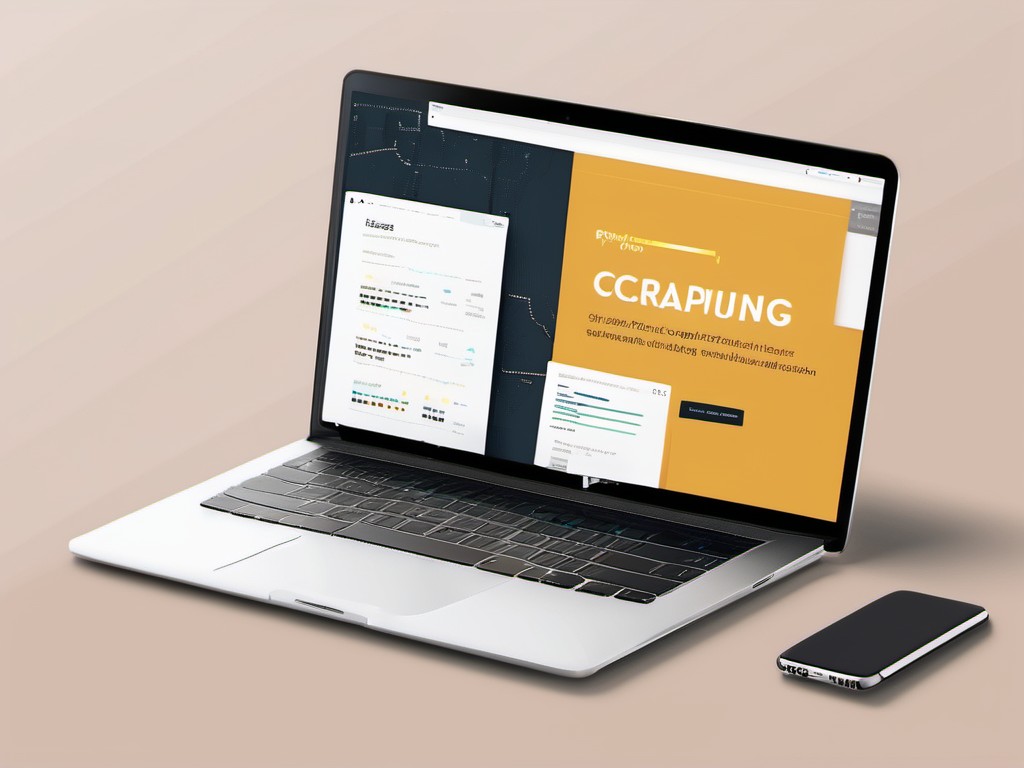
Web scraping has become an essential skill for data scientists, analysts, and developers alike. It allows you to extract valuable data from websites, enabling a wide range of applications from market research to automation tasks. Python, with its powerful libraries like BeautifulSoup, Requests, and Selenium, is one of the most popular tools for web scraping. Let’s dive into what Python web scraping is, how to get started, and explore some practical examples and advanced topics.
Introduction to Python Web Scraping
What is Web Scraping?
Web scraping, also known as web harvesting or web data extraction, involves using automated scripts to extract data from websites. This data can then be used for various purposes such as analysis, research, and more. Essentially, web scraping turns unstructured data into structured data that you can manipulate and analyze.
Why Use Python for Web Scraping?
Python is a favored language for web scraping due to its simplicity, readability, and the vast array of libraries available. Libraries like BeautifulSoup and Requests make it easy to parse HTML and send HTTP requests, while Selenium allows you to handle JavaScript-heavy websites. Additionally, Python’s extensive community support means you can find help and resources easily if you run into issues.
Getting Started with Python Web Scraping
Setting Up Your Environment
Before you start scraping, you need to set up your development environment. Here’s a quick guide:
- Install Python: Make sure you have the latest version of Python installed on your system. You can download it from python.org.
- Create a Virtual Environment: It’s good practice to create a virtual environment to keep your project dependencies isolated.
python -m venv myenv source myenv/bin/activate # On Windows use `myenv\Scripts\activate`
- Install Essential Libraries: Install the libraries you’ll need using pip.
pip install requests beautifulsoup4 selenium
Essential Libraries
BeautifulSoup
BeautifulSoup is a Python library used for web scraping to pull the data out of HTML and XML files. It creates parse trees from page source code that can be used to extract data in a hierarchical and readable manner.
from bs4 import BeautifulSoup
import requests
# Send a request to the website
response = requests.get('https://example.com')
web_content = response.text
# Create a BeautifulSoup object
soup = BeautifulSoup(web_content, 'html.parser')
# Extract data
title = soup.title.string
print(f'Title: {title}')
Requests
The requests
library is used to send HTTP requests using Python. It abstracts the complexities of making requests behind a beautiful, simple API so that you can focus on interacting with services and consuming data in your application.
import requests
# Send a GET request
response = requests.get('https://example.com')
print(response.text)
Selenium
Selenium is an open-source tool used for automating web browsers. It’s great for handling dynamic content that requires JavaScript to load.
from selenium import webdriver
# Set up the Selenium WebDriver
driver = webdriver.Chrome()
# Navigate to a URL
driver.get('https://example.com')
# Extract data
title_element = driver.find_element_by_tag_name('title')
print(f'Title: {title_element.text}')
# Close the WebDriver session
driver.quit()
Practical Examples and Tutorials
Scraping Basic Websites
Let’s start with a simple example of scraping a static website using BeautifulSoup and Requests.
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
web_content = response.text
soup = BeautifulSoup(web_content, 'html.parser')
title = soup.title.string
print(f'Title: {title}')
Handling Dynamic Content
For websites that load content dynamically using JavaScript, you’ll need to use Selenium.
from selenium import webdriver
import time
# Set up the WebDriver
driver = webdriver.Chrome()
url = 'https://example.com'
driver.get(url)
time.sleep(5) # Wait for JavaScript to load content
title_element = driver.find_element_by_tag_name('title')
print(f'Title: {title_element.text}')
# Close the WebDriver session
driver.quit()
Advanced Topics in Python Web Scraping
Dealing with CAPTCHA
CAPTCHAs are designed to prevent bots from accessing content, and dealing with them can be challenging. One common approach is using CAPTCHA-solving services like 2Captcha or Anti-Captcha, which you can integrate into your scraping scripts.
Respecting Robots.txt and Legal Considerations
Always check the robots.txt
file of a website to see if web scraping is allowed. This file provides instructions for web crawlers about which pages or files they can or cannot request from your site. Additionally, ensure you comply with legal regulations and terms of service when scraping data.
Applications of Python Web Scraping
Data Analysis
Web scraping allows you to gather large datasets that can be used for in-depth analysis. For example, you could scrape stock prices from financial websites or social media posts for sentiment analysis.
import pandas as pd
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
web_content = response.text
soup = BeautifulSoup(web_content, 'html.parser')
data = []
for item in soup.find_all('div', class_='item'):
name = item.find('span', class_='name').text
price = item.find('span', class_='price').text
data.append({'Name': name, 'Price': price})
df = pd.DataFrame(data)
print(df)
Market Research
Scrape competitor websites to gather information about their products, pricing, and customer reviews. This can provide valuable insights for your own business strategies.
Automation Tasks
Automate repetitive tasks such as extracting email addresses from a website or monitoring changes on a webpage over time. This can save significant time and effort compared to manual methods.
FAQ Section
What are the best Python libraries for web scraping?
The most commonly used libraries for web scraping in Python are BeautifulSoup, Requests, and Selenium. BeautifulSoup is great for parsing HTML and XML documents, while Requests is used to send HTTP requests. Selenium is ideal for handling dynamic content that requires JavaScript to load.
Is it legal to scrape websites?
The legality of web scraping depends on the website’s terms of service and local laws. Always check the robots.txt
file and ensure you are complying with the site’s policies. Additionally, respect user privacy and do not engage in malicious activities such as data theft or unauthorized access.
How do I handle JavaScript-heavy websites?
For websites that rely heavily on JavaScript to load content, Selenium is a powerful tool. It allows you to control a web browser programmatically and can handle dynamic content that other methods cannot.
Can I get banned from a website for scraping?
Yes, if you violate the website’s terms of service or robots.txt file, you could be banned. To avoid this, always respect the site’s policies, use appropriate headers in your requests, and add delays between requests to prevent overwhelming the server.
What are some common mistakes in web scraping and how can I avoid them?
Some common mistakes include not checking robots.txt
, sending too many requests too quickly, and not handling exceptions properly. To avoid these issues, always check the robots.txt file, use appropriate delays between requests, handle exceptions gracefully, and respect the site’s terms of service.
By following this guide, you should have a solid foundation in Python web scraping and be able to tackle various projects with confidence. Happy scraping!