· Charlotte Will · 6 min read
A Beginner's Guide to Making API Calls with Python for Web Development
Learn how to make API calls using Python for web development in this beginner-friendly guide. Master GET, POST, PUT, and DELETE requests, handle responses, and optimize your code with error handling and asynchronous calls. Perfect for aspiring developers looking to enhance their skills in modern web development.
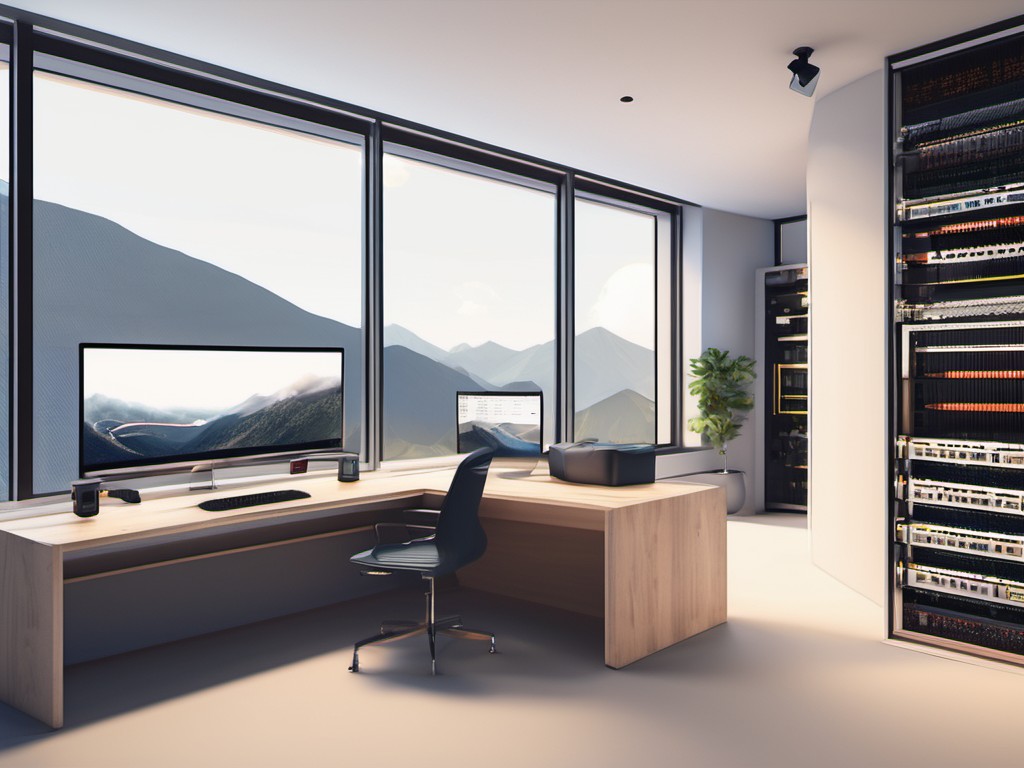
Welcome, aspiring web developer! Today, we’re going to dive into the exciting world of APIs (Application Programming Interfaces) and learn how to make API calls using Python. APIs are the backbone of modern web development, allowing different software applications to communicate with each other seamlessly. Let’s get started on this journey together!
Why Use APIs in Web Development?
Imagine you’re building a website that needs real-time weather updates. You could create your own weather database and update it manually, but that would be cumbersome and time-consuming. Instead, you can use an API from a service like OpenWeatherMap to fetch the latest weather data effortlessly. APIs help streamline processes, making web development more efficient and powerful.
What Are API Calls?
API calls are requests made to a server to retrieve or send data. When you make an API call, your application sends a request (usually via HTTP) to the server, which then processes that request and returns a response. This response often contains valuable data that your application can use.
Prerequisites
Before we dive in, ensure you have:
- A basic understanding of Python.
- An internet connection (to make actual API calls).
requests
library installed (you can install it usingpip install requests
).
Setting Up Your Environment
First things first, let’s set up your development environment:
- Install Python if you haven’t already.
- Open a terminal or command prompt.
- Create a new directory for your project and navigate into it.
- Use
pip install requests
to install the necessary library.
Now, let’s create a simple Python script to make our first API call!
Making Your First API Call
Open a text editor or IDE (like VSCode) and create a new file named api_call.py
. We’ll use the JSONPlaceholder API for this tutorial, as it’s designed for testing and prototyping.
import requests
def fetch_todos():
response = requests.get('https://jsonplaceholder.typicode.com/todos')
data = response.json()
return data
if __name__ == "__main__":
todos = fetch_todos()
print(todos)
Save the file and run it using python api_call.py
. You should see a list of todo items printed on your screen!
Understanding the Code
Let’s break down what’s happening in our script:
- Importing Requests Library: The
requests
library makes it easy to send HTTP requests using Python. - Defining a Function (fetch_todos): We define a function that will handle making the API call.
- Sending a GET Request:
requests.get()
sends an HTTP GET request to the specified URL. - Fetching Data: The server responds with data, which we convert from JSON format using
response.json()
. - Returning Data: We return the fetched data for further use.
- Main Block: The script checks if it’s being run directly and calls our function to print the data.
Exploring Different HTTP Methods
APIs typically support multiple HTTP methods like GET, POST, PUT, DELETE, etc. Let’s explore a few:
GET Request
We’ve already seen a GET request in action. It’s used to retrieve data from the server.
response = requests.get('https://api.example.com/data')
POST Request
A POST request is used to send data to the server, often to create new resources.
url = 'https://jsonplaceholder.typicode.com/todos'
data = {'title': 'Learn Python', 'completed': False}
response = requests.post(url, json=data)
print(response.json())
PUT Request
A PUT request is used to update existing resources on the server.
todo_id = 1
url = f'https://jsonplaceholder.typicode.com/todos/{todo_id}'
data = {'title': 'Updated Title', 'completed': True}
response = requests.put(url, json=data)
print(response.json())
DELETE Request
A DELETE request is used to remove resources from the server.
todo_id = 1
url = f'https://jsonplaceholder.typicode.com/todos/{todo_id}'
response = requests.delete(url)
print(response.status_code) # Should print 200 if successful
Handling API Responses
APIs can return various status codes to indicate the result of a request. Here are some common ones:
200 OK
: The request was successful.404 Not Found
: The requested resource could not be found.500 Internal Server Error
: Something went wrong on the server side.
Let’s handle these responses gracefully in our code:
import requests
def fetch_todos():
response = requests.get('https://jsonplaceholder.typicode.com/todos')
if response.status_code == 200:
data = response.json()
return data
else:
print(f"Error: {response.status_code}")
return None
if __name__ == "__main__":
todos = fetch_todos()
if todos is not None:
print(todos)
Using Headers and Query Parameters
Sometimes, you need to send additional information with your API requests. This can be done using headers and query parameters.
Headers
Headers are used to pass metadata about the request. For example, you might need to include an authorization token:
headers = {'Authorization': 'Bearer YOUR_TOKEN'}
response = requests.get('https://api.example.com/data', headers=headers)
Query Parameters
Query parameters are used to send additional data in the URL. For example, paginating a list of items:
params = {'page': 2, 'limit': 10}
response = requests.get('https://api.example.com/data', params=params)
Error Handling
Errors are inevitable in web development. Let’s add some error handling to our script:
import requests
def fetch_todos():
try:
response = requests.get('https://jsonplaceholder.typicode.com/todos')
if response.status_code == 200:
data = response.json()
return data
else:
print(f"Error: {response.status_code}")
return None
except requests.RequestException as e:
print(f"An error occurred: {e}")
return None
if __name__ == "__main__":
todos = fetch_todos()
if todos is not None:
print(todos)
Advanced Topics
Asynchronous Requests
If you need to make multiple API calls, consider using asynchronous requests with the aiohttp
library.
import aiohttp
import asyncio
async def fetch_todos():
async with aiohttp.ClientSession() as session:
async with session.get('https://jsonplaceholder.typicode.com/todos') as response:
data = await response.json()
return data
async def main():
todos = await fetch_todos()
print(todos)
if __name__ == "__main__":
asyncio.run(main())
Rate Limiting
Many APIs have rate limits to prevent abuse. Be mindful of these limits and consider implementing exponential backoff strategies to handle throttling.
Conclusion
Congratulations! You’ve taken your first steps into the world of API calls with Python. Remember, practice makes perfect – keep experimenting with different APIs and HTTP methods to solidify your understanding. Before you go, let’s address some common FAQs:
FAQs
1. How can I find the documentation for a specific API?
Most APIs provide comprehensive documentation on their official website or platforms like Postman and Swagger. Look for sections titled “API Documentation” or “Developer Resources”.
2. What should I do if my API call returns an error?
First, check the status code and message returned by the server. Common errors include missing parameters, incorrect URLs, or rate limits. Refer to the API documentation for specific error codes and solutions.
3. How can I secure my API keys?
Never hardcode your API keys directly into your source code. Use environment variables or configuration files that are not included in version control systems like Git.
4. What’s the difference between synchronous and asynchronous requests?
Synchronous requests block the execution of your program until a response is received, while asynchronous requests allow other tasks to run concurrently. Asynchronous requests are beneficial for making multiple API calls simultaneously.
5. How can I test my API calls locally before deploying them?
Use tools like Postman or curl to manually send HTTP requests and test your API endpoints. This helps ensure that your code works as expected before integrating it into a larger application.
Happy coding! 🚀